From Web to Desktop with NativePHP: A PHP Developer’s Journey and Lessons Learned
Hey everyone! I’m a PHP and Laravel developer who’s spent years building web applications. Recently, I stumbled across NativePHP—a tool that promises to take PHP beyond the browser and into desktop apps. I was curious, so I decided to test it with my own project, a simple CRM system. The result? Honestly, it blew me away! In this article, I’ll share my experience in three parts: Understanding what NativePHP is and how it works. Walking you through my hands-on project. Breaking down its strengths and weaknesses. If you’re a PHP developer wondering about desktop apps, stick with me—this might inspire you to try something new! 1. Understanding NativePHP: A New World for PHP Developers Let’s start with the basics. NativePHP is a framework that lets you turn Laravel projects into native desktop applications. It’s built to work with tools like Electron (the same tech behind apps like VS Code) or Tauri, which are popular for creating cross-platform desktop apps. The big idea? You can write PHP code—something we usually run on servers—and make it work as a standalone app on someone’s computer. Here’s how it happens: NativePHP takes your Laravel project, adds a PHP runtime (so the app can understand PHP without a server), and combines it with a front-end made of HTML, CSS, and JavaScript. It then packages everything into a single file that users can download and run. No need for them to install PHP, Apache, or anything else—it’s all included. For me, this was a game-changer. I’ve always thought of PHP as a web-only language, but NativePHP proves it can do more. The best part? If you know Laravel, you’re already halfway there. You can use Blade templates for the interface, Eloquent for data, and even Livewire if you want real-time updates. 2. Hands-On: Turning My Laravel CRM into a Desktop App Now, let’s get practical. I have a small Laravel project—a CRM system I built for managing clients. It worked fine as a web app, but one client asked, “Can I use this without a browser?” That’s when I decided to try NativePHP. Step 1: Setting It Up I started fresh to keep things clean. First, I created a new Laravel project: composer create-project laravel/laravel my-crm Then, I added NativePHP with Electron as the engine: composer require nativephp/electron php artisan native:install This step installed the necessary tools, including some Node.js dependencies. It took about five minutes, and I was ready to test it. I ran: php artisan native:serve A window popped up on my screen—my CRM was now a desktop app! The client list loaded perfectly, and the “Add Client” button worked just like it did online. I couldn’t believe how fast it came together. Step 2: Customizing the App Next, I wanted to tweak it. In the app/Providers/NativeAppServiceProvider.php file, I changed the window size and added a basic tray menu: use Native\Laravel\Facades\Window; use Native\Laravel\Facades\Menu; public function boot(): void { Window::open() ->width(900) ->height(700); Menu::new() ->add('Quit', fn() => app()->quit()) ->register(); } This made the app feel more polished. The window was bigger, and users could close it from the system tray. Step 3: Testing and Building I tested it on my Windows laptop and a friend’s Mac—it worked on both! To create a final version, I used: php artisan native:build This gave me an executable file (.exe for Windows, .dmg for Mac). My client installed it like any other app—no server setup, no browser. They loved it because it felt faster and more professional than opening it in Chrome. 3. The Pros and Cons: Is NativePHP Worth It? After playing with NativePHP, I’ve got a clear picture of its strengths and weaknesses. The Pros: ✅ Super Easy for Laravel Fans – If you know Laravel, you’ll feel at home. The setup is quick, and you don’t need to learn a new language or framework. ✅ Fast Results – Going from a web app to a desktop app takes almost no time. A few commands, some tweaks, and you’ve got something ready to share. ✅ Cross-Platform Magic – It works on Windows, macOS, and Linux with one codebase. ✅ PHP’s New Power – Using PHP for desktop apps feels fresh. I can use my server-side skills in a new way. ✅ User-Friendly – Clients don’t need technical knowledge to use it—just install and go. The Cons: ❌ Still in Beta – NativePHP isn’t fully polished yet. I hit a couple of bugs—like the build failing until I updated my Node.js version. ❌ Big File Size – Using Electron means the app can get heavy. My CRM ended up at 120 MB, which is a lot compared to a web app. ❌ Database Limits – Right now, it’s happiest with SQLite. If you rely on MySQL or PostgreSQL, you’ll need a workaround. ❌ Not Super Fast – While it’s quick to build, the app itself isn’t as snappy as something written in C++ or Rust. My Verdict: Nat
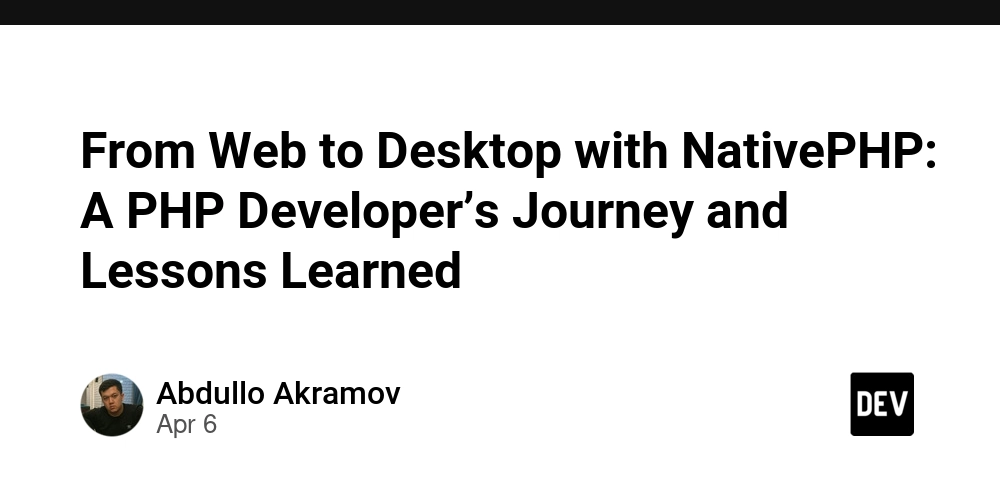
Hey everyone! I’m a PHP and Laravel developer who’s spent years building web applications. Recently, I stumbled across NativePHP—a tool that promises to take PHP beyond the browser and into desktop apps. I was curious, so I decided to test it with my own project, a simple CRM system. The result? Honestly, it blew me away!
In this article, I’ll share my experience in three parts:
- Understanding what NativePHP is and how it works.
- Walking you through my hands-on project.
- Breaking down its strengths and weaknesses.
If you’re a PHP developer wondering about desktop apps, stick with me—this might inspire you to try something new!
1. Understanding NativePHP: A New World for PHP Developers
Let’s start with the basics. NativePHP is a framework that lets you turn Laravel projects into native desktop applications. It’s built to work with tools like Electron (the same tech behind apps like VS Code) or Tauri, which are popular for creating cross-platform desktop apps.
The big idea? You can write PHP code—something we usually run on servers—and make it work as a standalone app on someone’s computer. Here’s how it happens:
- NativePHP takes your Laravel project, adds a PHP runtime (so the app can understand PHP without a server), and combines it with a front-end made of HTML, CSS, and JavaScript.
- It then packages everything into a single file that users can download and run. No need for them to install PHP, Apache, or anything else—it’s all included.
For me, this was a game-changer. I’ve always thought of PHP as a web-only language, but NativePHP proves it can do more. The best part? If you know Laravel, you’re already halfway there. You can use Blade templates for the interface, Eloquent for data, and even Livewire if you want real-time updates.
2. Hands-On: Turning My Laravel CRM into a Desktop App
Now, let’s get practical. I have a small Laravel project—a CRM system I built for managing clients. It worked fine as a web app, but one client asked, “Can I use this without a browser?” That’s when I decided to try NativePHP.
Step 1: Setting It Up
I started fresh to keep things clean. First, I created a new Laravel project:
composer create-project laravel/laravel my-crm
Then, I added NativePHP with Electron as the engine:
composer require nativephp/electron
php artisan native:install
This step installed the necessary tools, including some Node.js dependencies. It took about five minutes, and I was ready to test it. I ran:
php artisan native:serve
A window popped up on my screen—my CRM was now a desktop app! The client list loaded perfectly, and the “Add Client” button worked just like it did online. I couldn’t believe how fast it came together.
Step 2: Customizing the App
Next, I wanted to tweak it. In the app/Providers/NativeAppServiceProvider.php
file, I changed the window size and added a basic tray menu:
use Native\Laravel\Facades\Window;
use Native\Laravel\Facades\Menu;
public function boot(): void {
Window::open()
->width(900)
->height(700);
Menu::new()
->add('Quit', fn() => app()->quit())
->register();
}
This made the app feel more polished. The window was bigger, and users could close it from the system tray.
Step 3: Testing and Building
I tested it on my Windows laptop and a friend’s Mac—it worked on both! To create a final version, I used:
php artisan native:build
This gave me an executable file (.exe
for Windows, .dmg
for Mac). My client installed it like any other app—no server setup, no browser. They loved it because it felt faster and more professional than opening it in Chrome.
3. The Pros and Cons: Is NativePHP Worth It?
After playing with NativePHP, I’ve got a clear picture of its strengths and weaknesses.
The Pros:
✅ Super Easy for Laravel Fans – If you know Laravel, you’ll feel at home. The setup is quick, and you don’t need to learn a new language or framework.
✅ Fast Results – Going from a web app to a desktop app takes almost no time. A few commands, some tweaks, and you’ve got something ready to share.
✅ Cross-Platform Magic – It works on Windows, macOS, and Linux with one codebase.
✅ PHP’s New Power – Using PHP for desktop apps feels fresh. I can use my server-side skills in a new way.
✅ User-Friendly – Clients don’t need technical knowledge to use it—just install and go.
The Cons:
❌ Still in Beta – NativePHP isn’t fully polished yet. I hit a couple of bugs—like the build failing until I updated my Node.js version.
❌ Big File Size – Using Electron means the app can get heavy. My CRM ended up at 120 MB, which is a lot compared to a web app.
❌ Database Limits – Right now, it’s happiest with SQLite. If you rely on MySQL or PostgreSQL, you’ll need a workaround.
❌ Not Super Fast – While it’s quick to build, the app itself isn’t as snappy as something written in C++ or Rust.
My Verdict:
NativePHP isn’t perfect, but it’s a fantastic option for PHP developers who want to try desktop apps. It turned my CRM into something my client loves, and it didn’t take me out of my PHP comfort zone. It’s best for small-to-medium projects where speed and ease matter more than raw performance.
Final Thoughts: Why I’m Excited About NativePHP
Trying NativePHP showed me that PHP doesn’t have to stay in the browser. As a Laravel developer, I’m used to solving problems for the web, but now I can offer desktop solutions too—all with the tools I already know.
My little CRM experiment proved it’s practical and fun. Sure, there are rough edges, but for a tool this new, it’s impressive.
If you’re a PHP developer, I recommend giving NativePHP a shot. Start with a small project, play around, and see where it takes you.
Have you tried it yet? Or do you have questions about my experience? Let’s chat in the comments—I’d love to hear your thoughts!