BigInt: Handling Large Integers in JavaScript
BigInt: Handling Large Integers in JavaScript Introduction JavaScript, as a language designed primarily for web development, faced a significant limitation when dealing with large integer values. Standard JavaScript numbers (the Number type) are based on the IEEE 754 double-precision floating-point format, which can safely represent integers only up to (2^{53} - 1) (or 9,007,199,254,740,991). For applications requiring integer calculations beyond this range, a solution was needed. Enter BigInt—a new primitive data type introduced in ECMAScript 2020 (ES11) that allows representing whole numbers of arbitrary precision. This article provides a detailed exploration of BigInt, its technical underpinnings, usage, and its implications in modern JavaScript development. Historical Context and Evolution Prior to ECMAScript 2020, developers commonly either relied on the built-in Number type with its limitations or resorted to libraries like BigInteger.js, bignumber.js, or Decimal.js for handling big integer calculations. While these libraries have benefited many developers by providing large number manipulations, they often come with performance overhead and unnecessary complexity for many applications. With the release of BigInt in ES2020, JavaScript's standard library has been enhanced to natively support large integers through a new primitive type. The design of BigInt aimed at providing developers with a seamless and efficient method of working with large integers directly within the language itself, thereby improving performance, usability, and readability of the code. Specification and Implementation The BigInt proposal originated from the insights gained from communities surrounding JavaScript usage in fields requiring massive numeric calculations. Notably, it was formalized in the TC39 proposal process, which emphasizes on the need for solutions backed by usability studies and industry consensus. BigInts can be declared using either the BigInt constructor or by appending an "n" to the end of an integer literal, i.e., 123n. It is essential to note that BigInts are not interchangeable with regular numbers; any operations mixing the two types will lead to a TypeError. Technical Overview Basic Usage and Creation Creating a BigInt in JavaScript can be done in multiple ways: // Using BigInt constructor const bigIntFromConstructor = BigInt(12345678901234567890); // Using literal notation const bigIntFromLiteral = 12345678901234567890n; console.log(bigIntFromConstructor); // Output: 12345678901234567890n console.log(bigIntFromLiteral); // Output: 12345678901234567890n Mathematical Operations BigInts support standard arithmetic operations: Addition (+) Subtraction (-) Multiplication (*) Division (/) Exponentiation (**) Modulus (%) One significant behavior to note is that division of two BigInts will return a truncated integer. const a = 12345678901234567890n; const b = 1000000000000n; const addition = a + b; // 12345678902234567890n const division = a / b; // 12345678901n (integer division) const multiplication = a * b; // 12345678901234567890000000000000n console.log(addition, division, multiplication); Edge Cases and Advanced Implementations When working with BigInts, edge cases become crucial: Mixing Types: Trying to perform operations between BigInt and Number leads to a TypeError. const bigIntValue = 123n; const normalValue = 123; // A regular Number // TypeError: Cannot mix BigInt and other types, use explicit conversion const result = bigIntValue + normalValue; Type Conversion: To work around the mixing type error, explicit conversion is required. const result = bigIntValue + BigInt(normalValue); // works. Precision Issues: Be cautious with very large calculations; the introduction of precision cannot be underestimated. Real-World Use Cases Cryptography: BigInts are frequently used in cryptographic algorithms (RSA, Diffie-Hellman) where large prime numbers are crucial for public-key encryption. Blockchain Technologies: In platforms like Ethereum or Bitcoin, where transaction hashes can be significantly large numbers, BigInt serves to process transactions involving large amounts without precision errors. Scientific Computations: Simulations involving astronomical computations often exceed standard numerical limits. Performance Considerations and Optimization In performance-sensitive applications, using BigInt over large numbers should be weighed against the increased complexity it may introduce. Although BigInt performs efficiently for basic arithmetic operations, it can incur performance penalties when handling very large applications due to its arbitrary precision nature, which can lead to larger memory footprints. To optimize BigInt usage: Benchmark operations to ensure the cost-benefit analysis is positive before adopti
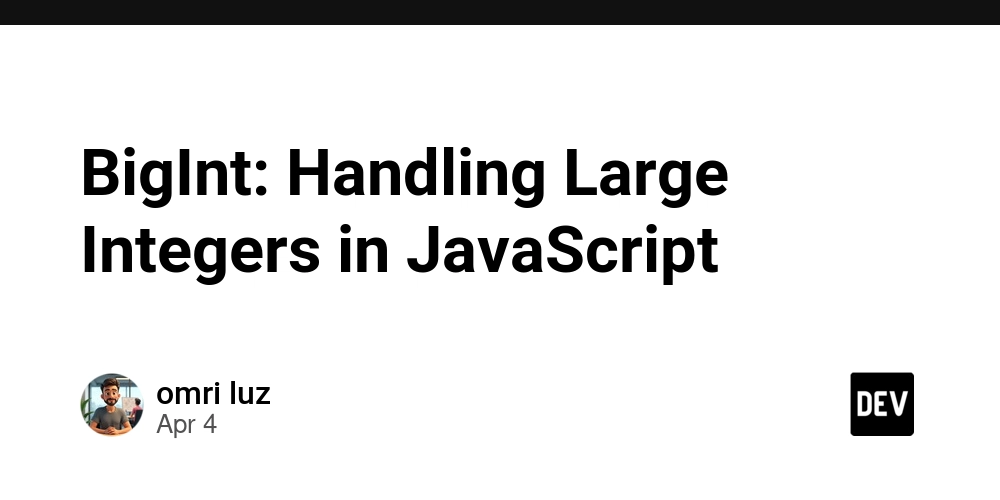
BigInt: Handling Large Integers in JavaScript
Introduction
JavaScript, as a language designed primarily for web development, faced a significant limitation when dealing with large integer values. Standard JavaScript numbers (the Number
type) are based on the IEEE 754 double-precision floating-point format, which can safely represent integers only up to (2^{53} - 1) (or 9,007,199,254,740,991). For applications requiring integer calculations beyond this range, a solution was needed. Enter BigInt—a new primitive data type introduced in ECMAScript 2020 (ES11) that allows representing whole numbers of arbitrary precision. This article provides a detailed exploration of BigInt, its technical underpinnings, usage, and its implications in modern JavaScript development.
Historical Context and Evolution
Prior to ECMAScript 2020, developers commonly either relied on the built-in Number
type with its limitations or resorted to libraries like BigInteger.js, bignumber.js, or Decimal.js for handling big integer calculations. While these libraries have benefited many developers by providing large number manipulations, they often come with performance overhead and unnecessary complexity for many applications.
With the release of BigInt in ES2020, JavaScript's standard library has been enhanced to natively support large integers through a new primitive type. The design of BigInt aimed at providing developers with a seamless and efficient method of working with large integers directly within the language itself, thereby improving performance, usability, and readability of the code.
Specification and Implementation
The BigInt proposal originated from the insights gained from communities surrounding JavaScript usage in fields requiring massive numeric calculations. Notably, it was formalized in the TC39 proposal process, which emphasizes on the need for solutions backed by usability studies and industry consensus.
BigInts can be declared using either the BigInt
constructor or by appending an "n" to the end of an integer literal, i.e., 123n
. It is essential to note that BigInts are not interchangeable with regular numbers; any operations mixing the two types will lead to a TypeError
.
Technical Overview
Basic Usage and Creation
Creating a BigInt in JavaScript can be done in multiple ways:
// Using BigInt constructor
const bigIntFromConstructor = BigInt(12345678901234567890);
// Using literal notation
const bigIntFromLiteral = 12345678901234567890n;
console.log(bigIntFromConstructor); // Output: 12345678901234567890n
console.log(bigIntFromLiteral); // Output: 12345678901234567890n
Mathematical Operations
BigInts support standard arithmetic operations:
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Exponentiation (
**
) - Modulus (
%
)
One significant behavior to note is that division of two BigInts will return a truncated integer.
const a = 12345678901234567890n;
const b = 1000000000000n;
const addition = a + b; // 12345678902234567890n
const division = a / b; // 12345678901n (integer division)
const multiplication = a * b; // 12345678901234567890000000000000n
console.log(addition, division, multiplication);
Edge Cases and Advanced Implementations
When working with BigInts, edge cases become crucial:
- Mixing Types: Trying to perform operations between BigInt and Number leads to a TypeError.
const bigIntValue = 123n;
const normalValue = 123; // A regular Number
// TypeError: Cannot mix BigInt and other types, use explicit conversion
const result = bigIntValue + normalValue;
- Type Conversion: To work around the mixing type error, explicit conversion is required.
const result = bigIntValue + BigInt(normalValue); // works.
- Precision Issues: Be cautious with very large calculations; the introduction of precision cannot be underestimated.
Real-World Use Cases
Cryptography: BigInts are frequently used in cryptographic algorithms (RSA, Diffie-Hellman) where large prime numbers are crucial for public-key encryption.
Blockchain Technologies: In platforms like Ethereum or Bitcoin, where transaction hashes can be significantly large numbers, BigInt serves to process transactions involving large amounts without precision errors.
Scientific Computations: Simulations involving astronomical computations often exceed standard numerical limits.
Performance Considerations and Optimization
In performance-sensitive applications, using BigInt over large numbers should be weighed against the increased complexity it may introduce. Although BigInt performs efficiently for basic arithmetic operations, it can incur performance penalties when handling very large applications due to its arbitrary precision nature, which can lead to larger memory footprints.
To optimize BigInt usage:
- Benchmark operations to ensure the cost-benefit analysis is positive before adopting BigInt in critical paths,
- Measure performance against libraries if your application has existing dependencies.
Debugging and Pitfalls
Debugging issues related to BigInt can arise from:
- Type Errors: Mixing BigInt with other types without type checks can obscure issues, leading to runtime exceptions.
- Unexpected Results: Due to how division behaves with BigInt resulting in floored values, ensure that logic expecting decimal results handles such cases correctly.
Advanced debugging techniques include:
-
Using Console Logging: Always check your types; use
console.log(typeof value)
frequently to catch potential type mismatches early. - Unit Testing: Implementing rigorous tests to check edge cases is essential for large number computations.
- TypeScript: Consider using TypeScript to enforce type checks, especially when mixing different number types.
Comparisons with Alternative Approaches
When reviewing BigInt against libraries like bignumber.js and decimal.js, consider:
- Performance: Native support offered by BigInt often leads to better performance due to optimized operations built directly into the JavaScript engine.
- Simplicity: BigInt maintains simplicity in usage by adhering to JavaScript’s typical arithmetic operators, whereas libraries may introduce complex syntax.
- Cross-Environment: Built-in support for BigInt across modern browsers offers broader compatibility as compared to external libraries requiring installation and configuration.
Conclusion
BigInt presents a powerful addition to JavaScript for dealing with large integers without sacrificing performance or developer productivity. Its integration alleviates many challenges faced by developers dealing with numeric limitations inherent in the traditional Number
type. Understanding its nuances is essential for advanced JavaScript practitioners, especially those in domains demanding stringent precision and performance.
For further reading and official references, developers are encouraged to consult the MDN Web Docs on BigInt. Additionally, refer to the ECMA-262 specification for in-depth technical details regarding its implementation.
Through this comprehensive exploration of BigInt, JavaScript developers can optimize and refine their applications, ensuring robustness and precision in numerical computations.