Monitoring SSL Certificate with CLI
Monitoring SSL Certificate with CLI: A Practical Guide Ever had that sinking feeling when you discover your production site is down because of an expired SSL certificate? You're not alone. Despite being entirely preventable, SSL certificate expirations remain one of the most common causes of outages, affecting companies of all sizes. What makes these incidents particularly frustrating is that they're completely avoidable with proper SSL certificate monitoring. Let's explore why this happens and how you can use simple CLI tools to prevent these issues. Why SSL Certificate Expirations Are Silent Killers When an SSL certificate expires, browsers immediately display security warnings, APIs stop working, and users lose trust in your service. Unlike other types of failures that might have gradual degradation, SSL expirations are binary – working perfectly one minute, completely broken the next. Here's how certificate expiration typically plays out: Timeline of a Certificate Expiration Incident: 1. Certificate expires at midnight 2. First user hits the site, sees security warning 3. Support tickets start trickling in 4. More users report the issue 5. Someone finally checks the certificate 6. Emergency renewal process begins 7. Certificate is renewed and deployed 8. Service gradually returns to normal 9. Team promises "never again"... until next time The real problem? Most teams don't have proper procedures to monitor SSL expiry dates proactively. The Hidden Costs of Certificate Expirations Certificate expirations impact more than just technical operations: Lost Revenue: Every minute your site displays a security warning is a minute customers can't make purchases Engineering Disruption: Emergency certificate renewals pull engineers away from planned work Brand Damage: Users increasingly associate security warnings with poor site management Support Overhead: Customer service teams get flooded with tickets during outages Setting Up CLI-Based SSL Certificate Monitoring Let's dive into practical solutions using command-line tools that you can implement today. Option 1: OpenSSL - The Swiss Army Knife OpenSSL is available on virtually every system and provides powerful certificate inspection capabilities. Basic Certificate Check To check a certificate directly from a website: # Check certificate expiration date openssl s_client -connect example.com:443 -servername example.com /dev/null | openssl x509 -noout -dates # Sample output: # notBefore=Apr 20 12:00:00 2023 GMT # notAfter=Apr 19 12:00:00 2024 GMT Extract Days Until Expiration For SSL certificate expiry monitoring, it's useful to get the exact number of days remaining: # Calculate days until expiration EXPIRY=$(openssl s_client -connect example.com:443 -servername example.com /dev/null | openssl x509 -noout -enddate | cut -d= -f2) EXPIRY_SECONDS=$(date -d "$EXPIRY" +%s) CURRENT_SECONDS=$(date +%s) DAYS_LEFT=$(( ($EXPIRY_SECONDS - $CURRENT_SECONDS) / 86400 )) echo "$DAYS_LEFT days until certificate expiration" Create a Simple Monitoring Script Let's turn this into a reusable monitoring script: #!/bin/bash # ssl-check.sh - Simple SSL certificate monitor DOMAINS=("example.com" "api.example.com" "admin.example.com") WARNING_THRESHOLD=30 # Alert when less than 30 days remaining for domain in "${DOMAINS[@]}"; do echo "Checking $domain..." # Get certificate expiration date EXPIRY=$(openssl s_client -connect $domain:443 -servername $domain /dev/null | openssl x509 -noout -enddate | cut -d= -f2) # Calculate days until expiration EXPIRY_SECONDS=$(date -d "$EXPIRY" +%s) CURRENT_SECONDS=$(date +%s) DAYS_LEFT=$(( ($EXPIRY_SECONDS - $CURRENT_SECONDS) / 86400 )) echo "$domain expires in $DAYS_LEFT days" # Check if expiration is approaching if [ $DAYS_LEFT -lt $WARNING_THRESHOLD ]; then echo "WARNING: $domain certificate expires in less than $WARNING_THRESHOLD days!" # Add notification logic here (email, Slack, etc.) fi done Set this script to run daily via cron: # Run SSL check daily at 9am 0 9 * * * /path/to/ssl-check.sh Option 2: SSL-Cert-Check - Purpose-Built for Monitoring While OpenSSL is versatile, dedicated tools like ssl-cert-check make SSL certificate monitoring even easier. Installation # On Debian/Ubuntu sudo apt-get install ssl-cert-check # On CentOS/RHEL sudo yum install ssl-cert-check # Alternative: Download and make executable wget https://github.com/Matty9191/ssl-cert-check/raw/master/ssl-cert-check chmod +x ssl-cert-check Basic Usage # Check a single domain ./ssl-cert-check -s example.com -p 443 # Check multiple domains from a file echo "example.com" > domains.txt echo "api.example.com" >> domains.txt ./ssl-cert-check -f domains.txt -p 443 Set Expiry Warnings # Alert when certificates expire in less than 30 da
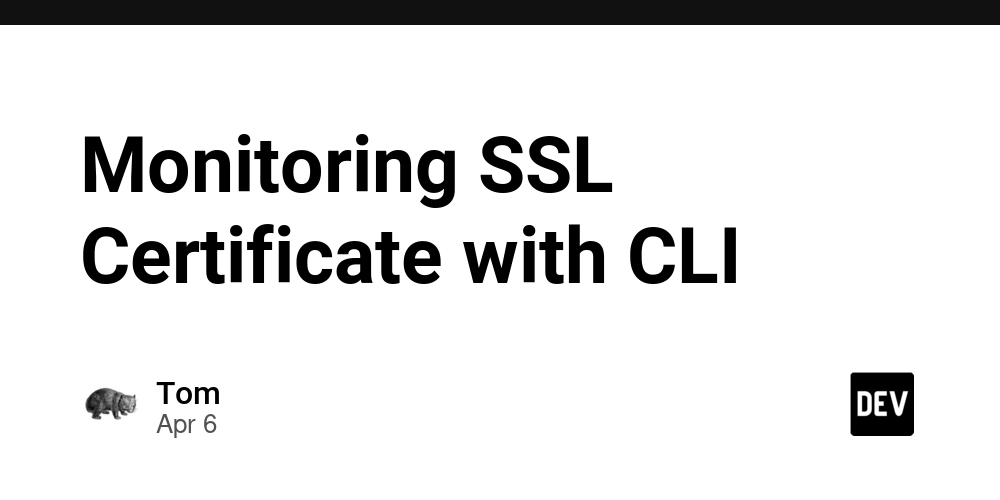
Monitoring SSL Certificate with CLI: A Practical Guide
Ever had that sinking feeling when you discover your production site is down because of an expired SSL certificate? You're not alone.
Despite being entirely preventable, SSL certificate expirations remain one of the most common causes of outages, affecting companies of all sizes. What makes these incidents particularly frustrating is that they're completely avoidable with proper SSL certificate monitoring.
Let's explore why this happens and how you can use simple CLI tools to prevent these issues.
Why SSL Certificate Expirations Are Silent Killers
When an SSL certificate expires, browsers immediately display security warnings, APIs stop working, and users lose trust in your service. Unlike other types of failures that might have gradual degradation, SSL expirations are binary – working perfectly one minute, completely broken the next.
Here's how certificate expiration typically plays out:
Timeline of a Certificate Expiration Incident:
1. Certificate expires at midnight
2. First user hits the site, sees security warning
3. Support tickets start trickling in
4. More users report the issue
5. Someone finally checks the certificate
6. Emergency renewal process begins
7. Certificate is renewed and deployed
8. Service gradually returns to normal
9. Team promises "never again"... until next time
The real problem? Most teams don't have proper procedures to monitor SSL expiry dates proactively.
The Hidden Costs of Certificate Expirations
Certificate expirations impact more than just technical operations:
Lost Revenue: Every minute your site displays a security warning is a minute customers can't make purchases
Engineering Disruption: Emergency certificate renewals pull engineers away from planned work
Brand Damage: Users increasingly associate security warnings with poor site management
Support Overhead: Customer service teams get flooded with tickets during outages
Setting Up CLI-Based SSL Certificate Monitoring
Let's dive into practical solutions using command-line tools that you can implement today.
Option 1: OpenSSL - The Swiss Army Knife
OpenSSL is available on virtually every system and provides powerful certificate inspection capabilities.
Basic Certificate Check
To check a certificate directly from a website:
# Check certificate expiration date
openssl s_client -connect example.com:443 -servername example.com /dev/null | openssl x509 -noout -dates
# Sample output:
# notBefore=Apr 20 12:00:00 2023 GMT
# notAfter=Apr 19 12:00:00 2024 GMT
Extract Days Until Expiration
For SSL certificate expiry monitoring, it's useful to get the exact number of days remaining:
# Calculate days until expiration
EXPIRY=$(openssl s_client -connect example.com:443 -servername example.com /dev/null | openssl x509 -noout -enddate | cut -d= -f2)
EXPIRY_SECONDS=$(date -d "$EXPIRY" +%s)
CURRENT_SECONDS=$(date +%s)
DAYS_LEFT=$(( ($EXPIRY_SECONDS - $CURRENT_SECONDS) / 86400 ))
echo "$DAYS_LEFT days until certificate expiration"
Create a Simple Monitoring Script
Let's turn this into a reusable monitoring script:
#!/bin/bash
# ssl-check.sh - Simple SSL certificate monitor
DOMAINS=("example.com" "api.example.com" "admin.example.com")
WARNING_THRESHOLD=30 # Alert when less than 30 days remaining
for domain in "${DOMAINS[@]}"; do
echo "Checking $domain..."
# Get certificate expiration date
EXPIRY=$(openssl s_client -connect $domain:443 -servername $domain /dev/null | openssl x509 -noout -enddate | cut -d= -f2)
# Calculate days until expiration
EXPIRY_SECONDS=$(date -d "$EXPIRY" +%s)
CURRENT_SECONDS=$(date +%s)
DAYS_LEFT=$(( ($EXPIRY_SECONDS - $CURRENT_SECONDS) / 86400 ))
echo "$domain expires in $DAYS_LEFT days"
# Check if expiration is approaching
if [ $DAYS_LEFT -lt $WARNING_THRESHOLD ]; then
echo "WARNING: $domain certificate expires in less than $WARNING_THRESHOLD days!"
# Add notification logic here (email, Slack, etc.)
fi
done
Set this script to run daily via cron:
# Run SSL check daily at 9am
0 9 * * * /path/to/ssl-check.sh
Option 2: SSL-Cert-Check - Purpose-Built for Monitoring
While OpenSSL is versatile, dedicated tools like ssl-cert-check make SSL certificate monitoring even easier.
Installation
# On Debian/Ubuntu
sudo apt-get install ssl-cert-check
# On CentOS/RHEL
sudo yum install ssl-cert-check
# Alternative: Download and make executable
wget https://github.com/Matty9191/ssl-cert-check/raw/master/ssl-cert-check
chmod +x ssl-cert-check
Basic Usage
# Check a single domain
./ssl-cert-check -s example.com -p 443
# Check multiple domains from a file
echo "example.com" > domains.txt
echo "api.example.com" >> domains.txt
./ssl-cert-check -f domains.txt -p 443
Set Expiry Warnings
# Alert when certificates expire in less than 30 days
./ssl-cert-check -s example.com -p 443 -x 30
# With email alerts
./ssl-cert-check -s example.com -p 443 -x 30 -e admin@example.com
Automate with Cron
# Daily check with email notification
0 9 * * * /path/to/ssl-cert-check -f /path/to/domains.txt -x 30 -e admin@example.com
Option 3: Certbot - For Let's Encrypt Certificates
If you're using Let's Encrypt certificates, Certbot is your best friend for both issuance and renewal management.
Check Certificate Status
sudo certbot certificates
# Sample output:
# Found the following certificates:
# Certificate Name: example.com
# Domains: example.com www.example.com
# Expiry Date: 2023-07-21 12:00:00+00:00 (VALID: 30 days)
# Certificate Path: /etc/letsencrypt/live/example.com/fullchain.pem
# Private Key Path: /etc/letsencrypt/live/example.com/privkey.pem
Test Renewal Process
sudo certbot renew --dry-run
Set Up Automatic Renewal
# Certbot creates this for you automatically in modern installations
sudo systemctl status certbot.timer
# If needed, create a cron job
0 3 * * * /usr/bin/certbot renew --quiet
Building a Comprehensive SSL Monitoring Strategy
While CLI tools provide a good foundation, a complete SSL certificate expiry monitoring strategy should include:
1. Centralized Certificate Inventory
Maintain a complete inventory of all certificates across your organization. For each certificate, track:
Domain(s) covered
Issuing authority
Expiration date
Renewal process
Owner/team responsible
This is especially important as organizations grow and responsibilities become distributed.
2. Tiered Alert System
Create a tiered notification system based on certificate importance and time to expiration:
Critical certificates (payment systems, main website):
- 90 days: Initial notification to certificate owner
- 60 days: Reminder to certificate owner
- 30 days: Notification to certificate owner + team lead
- 14 days: Daily reminders + management notification
- 7 days: Urgent notices to all stakeholders
Standard certificates (internal tools, staging environments):
- 30 days: Notification to certificate owner
- 14 days: Reminder to certificate owner + team
- 7 days: Daily reminders
3. Automated Renewal Where Possible
For many certificate types, renewal can be fully automated:
Let's Encrypt certificates with Certbot
AWS Certificate Manager with auto-renewal
Azure Key Vault with automatic rotation
For certificates requiring manual steps, document the process clearly and assign backup personnel.
4. Regular Validation and Testing
Periodically validate your monitoring system:
# Test expiration detection with an expired test certificate
openssl req -x509 -newkey rsa:4096 -keyout test-key.pem -out test-cert.pem -days 0 -nodes
./ssl-check.sh # Should trigger alerts
# Test renewal processes in staging environments
# Test notification systems with manual triggers
Common Pitfalls to Avoid
Even with monitoring in place, teams often fall into these traps:
1. Neglecting Subdomain Certificates
Main domains often get attention, but subdomains like api.example.com or admin.example.com are frequently overlooked. Ensure your monitoring covers all domains and subdomains.
2. Missing Internal Certificates
While public-facing certificates are obvious, internal certificates for VPNs, microservices, and development environments are easy to forget. Include these in your inventory.
3. Ignoring Certificate Chain Issues
A certificate may be valid, but if intermediate certificates expire, you'll still have problems. Test the entire certificate chain:
openssl s_client -connect example.com:443 -servername example.com -showcerts
4. Ownership Gaps During Team Changes
When team members leave or change roles, certificate ownership can fall through the cracks. Establish clear handover procedures for certificate responsibilities.
Next Steps: Beyond Basic Monitoring
As your infrastructure grows, consider these advanced approaches:
1. Certificate Lifecycle Management
Move beyond simple expiration monitoring to managing the entire certificate lifecycle:
Automated discovery of new certificates
Certificate request workflows
Approval processes
Deployment automation
Post-deployment validation
2. Certificate Transparency Monitoring
Monitor Certificate Transparency logs to detect unauthorized certificates issued for your domains:
# Using ct-monitor (https://github.com/crtsh/ct-monitor)
ct-monitor --domain example.com
3. Integration with Existing Tools
Connect your SSL certificate monitoring with broader monitoring and incident management systems:
Send alerts to PagerDuty or OpsGenie
Log certificate events to your SIEM
Include certificate health in dashboards
Add certificate checks to CI/CD pipelines
Conclusion
Effective SSL certificate expiry monitoring is a fundamental practice for maintaining service reliability. By implementing simple CLI tools and establishing clear processes, you can prevent the unnecessary outages and disruptions caused by expired certificates.
Remember that while the technical implementation is straightforward, the organizational aspects—clear ownership, reliable notification channels, and documented processes—are equally important for success.
Start small with basic monitoring scripts, then gradually build toward a comprehensive certificate management strategy that grows with your organization.
For more detailed guidance on implementing effective SSL certificate monitoring with additional CLI examples and automation techniques, check out our comprehensive guide on the Bubobot blog.
SSLMonitoring #DevOpsTips #WebSecurity
Read more at https://bubobot.com/blog/monitoring-ssl-certificate-expiry-with-cli-1?utm_source=dev.to