From Go to Rust: A Developer's Journey Through Systems Programming
As a Golang developer building robust, scalable backend systems, I've always appreciated Go's elegant simplicity. Its minimal syntax and powerful standard library have been my trusted tools for creating efficient software solutions for years. But recently, I decided to step outside my comfort zone and explore Rust, a language I'd been hearing so much about. What began as casual curiosity evolved into a fascinating journey that's changed how I think about systems programming. The Engineer's Perspective on Language Philosophy With my background in Electrical Engineering, I've always approached programming from a systems perspective, trying to understand how things work from first principles. This mindset helped me appreciate both languages for what they truly offer: Go: As someone who builds distributed systems and event-driven architectures with technologies like Kafka, I value Go's approach to simplicity. Its straightforward concurrency model makes developing scalable services almost intuitive. Rust: My interest in low-level programming and computational efficiency drew me to Rust's promise of performance without sacrificing safety. What I discovered was a language that complements my methodical approach to problem-solving. The transition between these languages feels like switching between different engineering disciplines—each with its own principles, trade-offs, and elegant solutions to complex problems. Syntax: From Familiar Comfort to Expressive Power Coming from Go's minimalist syntax, Rust initially felt overwhelming. The differences start with the fundamentals: In Go (my comfort zone): // Simple and straightforward func ProcessChunks(data []byte, chunkSize int) []string { var results []string // Processing logic return results } In Rust (my new challenge): // More explicit, with lifetime annotations and ownership details fn process_chunks Vec { let mut results = Vec::new(); // Processing logic with ownership considerations results } After working with both languages on various projects—from my text indexer "Jam-Text" in Go to newer experiments in Rust—I've found that Rust's verbosity actually encourages more thoughtful program design. The compiler forces me to think about issues I might have overlooked in Go. Error Handling: From Checking Nil to Embracing Results Error handling was one of the biggest mental shifts. During the Jam-Text hackathon project, our team wrote plenty of Go code with the familiar pattern: index, err := CreateTextIndex(documents) if err != nil { log.Fatalf("Failed to create index: %v", err) } Rust's approach felt alien at first: let index = create_text_index(documents)?; The ? operator seemed like magic compared to Go's explicit error checking. But after using it in practice, I realized how it transforms error handling from boilerplate into expressive flow control. This reminded me of designing circuits in my engineering days—where signal propagation followed natural paths with built-in safeguards. Concurrency: Goroutines vs. Ownership-Based Threading Having implemented parallel processing in my Go projects, especially for chunk handling in the text indexer, I was skeptical about Rust's more complex threading model: In Go: // From my Jam-Text project for _, chunk := range chunks { wg.Add(1) go func(c []byte) { defer wg.Done() // Process chunk concurrently }(chunk) } In Rust: let handles: Vec = chunks .iter() .map(|chunk| { thread::spawn(move || { // Process chunk with ownership guarantees }) }) .collect(); for handle in handles { handle.join().unwrap(); } The Rust version requires more consideration about data ownership, but after working through borrow checker errors (and there were many!), I found my concurrent code had fewer subtle bugs. The compiler was catching race conditions that might have shipped to production in my Go code. The Borrow Checker: From Frustration to Enlightenment If I'm being honest, my first few weeks with Rust were humbling. Coming from Go's garbage collector, Rust's ownership system felt like trying to solve a puzzle where the rules kept changing. I struggled with basic operations that would have been trivial in Go. The breakthrough came when I approached it like an engineering problem rather than a programming task. I drew out memory diagrams on paper, thinking about ownership transfers the way I'd think about signal flow in a circuit. Suddenly, the borrow checker wasn't my enemy—it was a collaborator helping me design better systems. Practical Applications: Where Each Language Shines Through my journey with both languages, I've developed a practical sense of when to use each: Go: For my distributed systems work, API services, and when rapid development is crucial. My custom implementation of the Unix ls command i
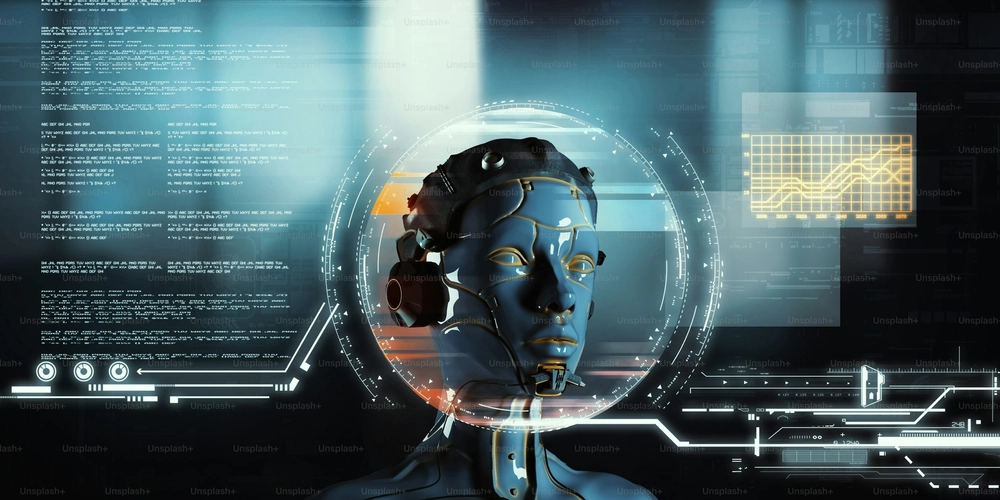
As a Golang developer building robust, scalable backend systems, I've always appreciated Go's elegant simplicity. Its minimal syntax and powerful standard library have been my trusted tools for creating efficient software solutions for years. But recently, I decided to step outside my comfort zone and explore Rust, a language I'd been hearing so much about. What began as casual curiosity evolved into a fascinating journey that's changed how I think about systems programming.
The Engineer's Perspective on Language Philosophy
With my background in Electrical Engineering, I've always approached programming from a systems perspective, trying to understand how things work from first principles. This mindset helped me appreciate both languages for what they truly offer:
Go: As someone who builds distributed systems and event-driven architectures with technologies like Kafka, I value Go's approach to simplicity. Its straightforward concurrency model makes developing scalable services almost intuitive.
Rust: My interest in low-level programming and computational efficiency drew me to Rust's promise of performance without sacrificing safety. What I discovered was a language that complements my methodical approach to problem-solving.
The transition between these languages feels like switching between different engineering disciplines—each with its own principles, trade-offs, and elegant solutions to complex problems.
Syntax: From Familiar Comfort to Expressive Power
Coming from Go's minimalist syntax, Rust initially felt overwhelming. The differences start with the fundamentals:
In Go (my comfort zone):
// Simple and straightforward
func ProcessChunks(data []byte, chunkSize int) []string {
var results []string
// Processing logic
return results
}
In Rust (my new challenge):
// More explicit, with lifetime annotations and ownership details
fn process_chunks<'a>(data: &'a [u8], chunk_size: usize) -> Vec<String> {
let mut results = Vec::new();
// Processing logic with ownership considerations
results
}
After working with both languages on various projects—from my text indexer "Jam-Text" in Go to newer experiments in Rust—I've found that Rust's verbosity actually encourages more thoughtful program design. The compiler forces me to think about issues I might have overlooked in Go.
Error Handling: From Checking Nil to Embracing Results
Error handling was one of the biggest mental shifts. During the Jam-Text hackathon project, our team wrote plenty of Go code with the familiar pattern:
index, err := CreateTextIndex(documents)
if err != nil {
log.Fatalf("Failed to create index: %v", err)
}
Rust's approach felt alien at first:
let index = create_text_index(documents)?;
The ?
operator seemed like magic compared to Go's explicit error checking. But after using it in practice, I realized how it transforms error handling from boilerplate into expressive flow control. This reminded me of designing circuits in my engineering days—where signal propagation followed natural paths with built-in safeguards.
Concurrency: Goroutines vs. Ownership-Based Threading
Having implemented parallel processing in my Go projects, especially for chunk handling in the text indexer, I was skeptical about Rust's more complex threading model:
In Go:
// From my Jam-Text project
for _, chunk := range chunks {
wg.Add(1)
go func(c []byte) {
defer wg.Done()
// Process chunk concurrently
}(chunk)
}
In Rust:
let handles: Vec<_> = chunks
.iter()
.map(|chunk| {
thread::spawn(move || {
// Process chunk with ownership guarantees
})
})
.collect();
for handle in handles {
handle.join().unwrap();
}
The Rust version requires more consideration about data ownership, but after working through borrow checker errors (and there were many!), I found my concurrent code had fewer subtle bugs. The compiler was catching race conditions that might have shipped to production in my Go code.
The Borrow Checker: From Frustration to Enlightenment
If I'm being honest, my first few weeks with Rust were humbling. Coming from Go's garbage collector, Rust's ownership system felt like trying to solve a puzzle where the rules kept changing. I struggled with basic operations that would have been trivial in Go.
The breakthrough came when I approached it like an engineering problem rather than a programming task. I drew out memory diagrams on paper, thinking about ownership transfers the way I'd think about signal flow in a circuit. Suddenly, the borrow checker wasn't my enemy—it was a collaborator helping me design better systems.
Practical Applications: Where Each Language Shines
Through my journey with both languages, I've developed a practical sense of when to use each:
Go: For my distributed systems work, API services, and when rapid development is crucial. My custom implementation of the Unix
ls
command in Go is a perfect example of leveraging Go's standard library and simplicity for system utilities.Rust: When performance and memory safety are critical. I've started migrating some computational components of my projects to Rust, especially those dealing with data processing algorithms similar to the SimHash fingerprinting we implemented in Jam-Text.
Lessons for Golang Developers Considering Rust
If you're a Gopher thinking about exploring Rust, here's what I wish someone had told me:
Start small: Don't try to rewrite your entire Go application. Begin with a self-contained utility or module.
Expect the learning curve: Your productivity will drop temporarily—embrace it as part of the learning process.
Think in ownership: Before writing code, sketch out which functions and structures own which data.
Learn to love the compiler: Those cryptic error messages are actually saving you from subtle bugs.
Bring your Go discipline: Go's emphasis on simplicity and readability remains valuable in Rust code.
The Unexpected Benefits
What surprised me most wasn't the technical capabilities of Rust, but how learning it improved my Go code. I now write more memory-conscious Go, think more carefully about mutability, and design better abstractions. The mental models from Rust have made me a better programmer overall.
During my time at Zone01 Kisumu as a software developer, I've had the opportunity to work with both languages, and I've found that this dual perspective makes me a more versatile problem solver. Each language has taught me something valuable about the other.
Conclusion: Two Languages, One Engineering Mindset
As someone with a background in Electrical Engineering who approaches programming from first principles, I've come to see Go and Rust not as competing technologies but as complementary tools in my development arsenal. Go offers simplicity and productive development for scalable services, while Rust provides control and safety for performance-critical components.
The journey from Go to Rust isn't about leaving one language behind—it's about expanding your mental models and having more tools to solve complex problems. For a methodical thinker interested in distributed systems and computational efficiency, having both languages at your disposal is invaluable.
I'm continuing to build with Go while exploring Rust's ecosystem, just as I continue developing my expertise in container orchestration, infrastructure automation, and event-driven architectures. The intersection of these technologies provides endless opportunities for creating efficient, robust software solutions.
Have you made a similar journey between programming languages? I'd love to hear about your experiences in the comments. And if you're working on interesting projects in either Go or Rust, let's connect!