How to use the array filter() method in JavaScript
Written by Abiola Farounbi✏️ The array filter() method does exactly what its name suggests; it filters an array based on a given condition, returning a new array that contains only the elements that meet the specified condition. In this article, we will learn how the array filter() method works, practical use cases, advanced techniques, and best practices to help you write efficient filtering logic. Syntax and parameters of the array filter()method The array filter() method is defined using this format: const newArray = originalArray.filter(callbackFunction(element,index, array),thisArg); The method takes in the following parameters and arguments: callbackFunction — Defines the rule for filtering. It is invoked once for each element in the array: element — The current element being processed index (optional) — The index of the current element. It starts counting from 0 **array (optional)** — The original array to be filtered Return — The callbackFunction returns a Boolean value. If true, the element is included in the new array thisArg (optional) — A value to use as this inside the callbackFunction. This parameter is ignored if the callback is an arrow function, as arrow functions don’t have this binding Return value — The filter() method returns a new array containing only the elements that satisfy the filtering condition Note: If no element passes the condition, it will return an empty array. How the array filter() method works To understand the step-by-step process of how the array filter() method works, let's look at an example scenario without the filter method, vs. with the filter method. Assume that we have an array of words and only want to get the words longer than five characters from the array. Without filter(), we would traditionally use a for loop like this: With the for loop, we had to take an extra step by manually iterating through the array and adding each matching value individually. Now, let’s simplify this process using the filter() method: The filter() method itself is an iterative method, so it does not require the additional step of using a for loop, and based on the result from the callback function, each element from the array is automatically added to a new array. It’s important to note that this method is designed specifically for arrays. To use it with other JavaScript data types, they must first be converted into an array. Practical use cases of the array filter() method This method can be implemented in solving logic problems in real-world applications and solutions. Here are some examples that are most commonly used: Basic filtering Let’s consider an example where we want to filter out a list of product prices to find those above a certain threshold. To solve this effectively, we can use an arrow function to define the filtering condition concisely inside the filter method: const productPrices = [15.99, 25.50, 10.00, 30.75, 5.49, 22.00]; const expensiveProducts = productPrices.filter(price => price > 20); console.log(expensiveProducts) // Result: [25.5, 30.75, 22] Searching in an array One of the most common use cases for the filter() method is implementing a search feature. It can be used to filter a list of names based on a search query, returning only the items that match. To ensure case-insensitive matching, it's best to convert both the search query and the array elements to lowercase before filtering: const names = ['John', 'Alice', 'Jonathan', 'Bob', 'Joanna']; const searchQuery = 'jo'; const searchResults = names.filter(name => name.toLowerCase().includes(searchQuery.toLowerCase()) ); console.log(searchResults) // Result: ['John', 'Jonathan', 'Joanna'] Filtering objects by specific properties The filter() method can be used on arrays of objects, allowing you to filter them based on specific property values. Let’s consider a content platform where each post is represented as an object with properties like id, title, and tags. If you want to display only the posts that are tagged as "tech", you can use the filter() method to extract only those posts: const posts = [ { id: 1, title: 'AI Breakthrough', tags: ['tech', 'science']}, { id: 2, title: 'Travel Guide', tags: ['lifestyle'] }, { id: 3, title: 'New JavaScript Framework', tags: ['tech'] }, ]; const techPosts = posts.filter(post => post.tags.includes('tech')) console.log(techPosts) // Result: [ // { id: 1, title: 'AI Breakthrough', tags: [ 'tech', 'science' ] }, // { id: 3, title: 'New JavaScript Framework', tags: [ 'tech' ] } // ] Filtering invalid data When working with raw data, it's common to encounter invalid values or edge cases that must be removed before further processing. Instead of manually checking and cleaning the data, we can use the filter() method to do this efficiently: const rawData = ["Hello", "", 42, null, undefined, "Java
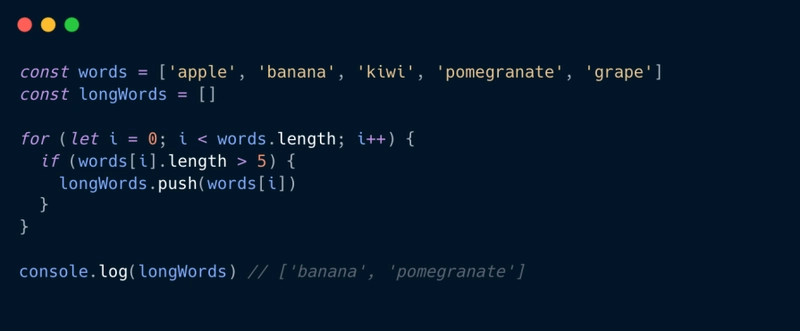
Written by Abiola Farounbi✏️
The array filter()
method does exactly what its name suggests; it filters an array based on a given condition, returning a new array that contains only the elements that meet the specified condition.
In this article, we will learn how the array filter()
method works, practical use cases, advanced techniques, and best practices to help you write efficient filtering logic.
Syntax and parameters of the array filter()
method
The array filter()
method is defined using this format:
const newArray = originalArray.filter(callbackFunction(element,index, array),thisArg);
The method takes in the following parameters and arguments:
-
callbackFunction
— Defines the rule for filtering. It is invoked once for each element in the array:-
element
— The current element being processed -
index
(optional) — The index of the current element. It starts counting from 0 -
**array
(optional)** — The original array to be filtered - Return — The
callbackFunction
returns a Boolean value. Iftrue
, the element is included in the new array
-
-
thisArg
(optional) — A value to use asthis
inside thecallbackFunction
. This parameter is ignored if the callback is an arrow function, as arrow functions don’t havethis
binding - Return value — The
filter()
method returns a new array containing only the elements that satisfy the filtering condition
Note: If no element passes the condition, it will return an empty array.
How the array filter()
method works
To understand the step-by-step process of how the array filter()
method works, let's look at an example scenario without the filter method, vs. with the filter method.
Assume that we have an array of words and only want to get the words longer than five characters from the array. Without filter()
, we would traditionally use a for
loop like this: With the
for
loop, we had to take an extra step by manually iterating through the array and adding each matching value individually.
Now, let’s simplify this process using the filter()
method: The
filter()
method itself is an iterative method, so it does not require the additional step of using a for
loop, and based on the result from the callback function, each element from the array is automatically added to a new array.
It’s important to note that this method is designed specifically for arrays. To use it with other JavaScript data types, they must first be converted into an array.
Practical use cases of the array
filter()
method
This method can be implemented in solving logic problems in real-world applications and solutions. Here are some examples that are most commonly used:
Basic filtering
Let’s consider an example where we want to filter out a list of product prices to find those above a certain threshold.
To solve this effectively, we can use an arrow function to define the filtering condition concisely inside the filter method:
const productPrices = [15.99, 25.50, 10.00, 30.75, 5.49, 22.00];
const expensiveProducts = productPrices.filter(price => price > 20);
console.log(expensiveProducts)
// Result: [25.5, 30.75, 22]
Searching in an array
One of the most common use cases for the filter()
method is implementing a search feature. It can be used to filter a list of names based on a search query, returning only the items that match.
To ensure case-insensitive matching, it's best to convert both the search query and the array elements to lowercase before filtering:
const names = ['John', 'Alice', 'Jonathan', 'Bob', 'Joanna'];
const searchQuery = 'jo';
const searchResults = names.filter(name =>
name.toLowerCase().includes(searchQuery.toLowerCase())
);
console.log(searchResults)
// Result: ['John', 'Jonathan', 'Joanna']
Filtering objects by specific properties
The filter()
method can be used on arrays of objects, allowing you to filter them based on specific property values.
Let’s consider a content platform where each post is represented as an object with properties like id
, title
, and tags
. If you want to display only the posts that are tagged as "tech"
, you can use the filter()
method to extract only those posts:
const posts = [
{ id: 1, title: 'AI Breakthrough', tags: ['tech', 'science']},
{ id: 2, title: 'Travel Guide', tags: ['lifestyle'] },
{ id: 3, title: 'New JavaScript Framework', tags: ['tech'] },
];
const techPosts = posts.filter(post => post.tags.includes('tech'))
console.log(techPosts)
// Result: [
// { id: 1, title: 'AI Breakthrough', tags: [ 'tech', 'science' ] },
// { id: 3, title: 'New JavaScript Framework', tags: [ 'tech' ] }
// ]
Filtering invalid data
When working with raw data, it's common to encounter invalid values or edge cases that must be removed before further processing. Instead of manually checking and cleaning the data, we can use the filter()
method to do this efficiently:
const rawData = ["Hello", "", 42, null, undefined, "JavaScript"];
const cleanData = rawData.filter(item => item !== null && item !== undefined && item !== '');
console.log(cleanData);
//Result: [ 'Hello', 42, 'JavaScript' ]
A simpler alternative to filter out unwanted values is using Boolean
as the callback function. This removes all falsy values, including null
, undefined
, false
, ""
(empty strings), and NaN
(Not a Number):
const cleanData = rawData.filter(Boolean);
Filtering of duplicate values
When working with arrays, duplicates can often exist. Whether it’s from user input or an external source, we need a way to remove them efficiently. This can be done by combining the filter()
method with the indexOf()
method:
const duplicates = [1, 2, 2, 3, 4, 4, 5];
const uniqueValues = duplicates.filter((item, index, arr) =>
arr.indexOf(item) === index
//Compares the first occurrence index with the current index
);
console.log(uniqueValues)
// Result: [1, 2, 3, 4, 5]
indexOf(item)
finds the first index of the element in the array. By comparing the current index to this first index, we ensure only the first occurrence of each item is kept in the array. This method works well for arrays of primitive values (numbers, strings). It doesn't handle deduplication for objects, as each object reference is unique.
Advanced techniques: Chaining filter()
with other array methods
Now that we have a solid understanding of how the filter()
method works, we can take it a step further by combining it with other array methods like map()
and reduce()
using chaining.
Chaining in JavaScript is a method that allows multiple functions to be linked together, with each function passing its output as the input to the next. This works because many JavaScript methods return an object, enabling consecutive method calls in a seamless flow.
By chaining array methods, we can efficiently perform complex transformations while keeping the code concise and readable.
Before diving into chaining, let’s quickly review how these methods work individually:
-
**filter()**
– Narrows down the array by selecting only elements that meet a specific condition -
map()
– Transforms each element in an array and returns a new array with modified values -
reduce()
– Aggregates array values into a single result (such as sum, average, count)
Let's explore how these methods can be combined for advanced use cases.
Chaining filter()
with map()
A common use case for chaining filter()
and map()
is refining API response data. Often, the responses contain irregularities, such as empty values or invalid entries. By combining these methods, we can filter out invalid or unwanted data and transform the remaining data into a structured format.
In a content management system (CMS), it is common to retrieve a list of articles via an API that may include entries with missing titles or those still in draft status. To ensure that only published articles with valid titles are displayed to users, we can chain these methods:
const dummyData = [
{ id: 1, title: ' JavaScript Guide ', status: 'DRAFT' },
{ id: 2, title: 'React Basics', status: 'PUBLISHED' },
{ id: 3, title: ' ', status: 'PUBLISHED' }, // Invalid title
];
const publishedArticles = dummyData
.filter(item => item.title.trim() !== '' && item.status === 'PUBLISHED')
// Remove empty titles and drafts
.map(item => ({
...item, // Copy all values from the original array
title: item.title.trim().toLowerCase(), // Normalize title
}));
console.log(publishedArticles);
// Result: [{ id: 2, title: 'react basics', status: 'PUBLISHED' }]
Chaining filter()
with reduce()
These array methods can be useful when we need to filter specific elements and then accumulate the result.
For example, consider an ecommerce platform where we have a list of products, but not all of them are currently in stock.
We want to calculate the total value of all available products by filtering out out-of-stock items and adding up the prices of the remaining products:
const products = [
{ name: 'Laptop', price: 1000, inStock: true },
{ name: 'Phone', price: 500, inStock: false },
{ name: 'Tablet', price: 750, inStock: true },
]
const totalStockValue = products
.filter((product) => product.inStock) // Keep only in-stock products
.reduce((sum, product) => sum + product.price, 0) // Add up prices
console.log(totalStockValue)
// Result: 1750
Best practices for writing efficient filtering logic
While the filter()
method is incredibly simple to use, following the few best practices listed below can help ensure your code is both efficient and easy to maintain.
Optimize your callback
Keep the logic inside your callback simple by avoiding heavy computations or complex calculations. Precompute values when possible, and use built-in functions for better performance.
Make use of arrow functions
This keeps your code concise, readable, and reduces boilerplate.
Break down complex chains
It’s good to chain methods like filter()
, map()
, and reduce()
since it avoids reusing intermediate variables. However, deep nesting might become too unreadable. You could consider splitting them into name-oriented functions such that your code will be easier to read.
Maintain immutability
When transforming objects, be cautious not to mistakenly mutate your data. Use the spread operator ...
to create new objects, ensuring your original data remains unchanged.
Consider performance implications
For very large datasets, for-loops can sometimes outperform filter()
. Although filter()
is concise and readable, in performance-sensitive contexts, an optimized loop might be the better choice.
Conclusion
In this article, we‘ve discussed the array filter()
method, from its basic syntax and use cases to more advanced techniques like chaining with map()
and reduce()
.
By understanding and applying the best practices outlined here, you can write cleaner, more efficient, and more maintainable code.
I hope this tutorial was useful to you! If you have any questions, feel free to reach out to me on X. Happy coding!
LogRocket: Debug JavaScript errors more easily by understanding the context
Debugging code is always a tedious task. But the more you understand your errors, the easier it is to fix them.
LogRocket allows you to understand these errors in new and unique ways. Our frontend monitoring solution tracks user engagement with your JavaScript frontends to give you the ability to see exactly what the user did that led to an error.
LogRocket records console logs, page load times, stack traces, slow network requests/responses with headers + bodies, browser metadata, and custom logs. Understanding the impact of your JavaScript code will never be easier!