Java StrictMath scalb() Method: Syntax, Usage, and Examples
Java provides a variety of mathematical methods to help developers perform precise calculations. Among these, the StrictMath scalb() method in Java is a powerful function that scales floating-point numbers efficiently. This method is particularly useful in scenarios where shifting the binary exponent of a floating-point number is required. In this blog, we will explore the StrictMath scalb() method in Java, understand its syntax, and discuss practical applications. Whether you are a beginner or an experienced developer, this Java tutorial will help you grasp the importance of this method in mathematical computations. What is the StrictMath scalb() Method in Java? The StrictMath scalb() method is part of the StrictMath class, which is known for providing consistent mathematical computations across different platforms. This method scales a floating-point number by a power of two, making it equivalent to multiplying the number by 2^scaleFactor. Unlike simple multiplication, scalb() ensures precision and efficiency, especially in performance-critical applications such as graphics rendering, scientific computing, and financial calculations. Syntax of the StrictMath scalb() Method The StrictMath scalb() method in Java follows this syntax: public static double scalb(double d, int scaleFactor) public static float scalb(float f, int scaleFactor) Parameters d or f – The floating-point number that needs to be scaled. scaleFactor – An integer that represents the power of two by which the floating-point number will be multiplied. Return Value The method returns a floating-point number that is the result of multiplying the input value by 2^scaleFactor. Why Use the StrictMath scalb() Method? 1. Precision and Accuracy Unlike traditional multiplication, scalb() is optimized for floating-point calculations, reducing rounding errors that might occur in complex computations. 2. Performance Optimization Since multiplying by powers of two is a fundamental operation in computing, scalb() is implemented in a way that makes it more efficient than direct multiplication using loops or recursion. 3. Consistency Across Platforms The StrictMath class ensures that results remain consistent across different hardware and operating systems, which is critical for applications requiring deterministic output. Real-World Applications 1. Scientific and Engineering Calculations Many scientific applications involve scaling floating-point numbers efficiently. Whether it's processing sensor data or performing physics simulations, scalb() is a reliable method for such computations. 2. Graphics and Game Development In graphics rendering, transformations often require adjusting values in powers of two. Using scalb() ensures that these operations are performed efficiently, enhancing performance in real-time applications like video games and simulations. 3. Financial Computations Many financial models require precise calculations involving large numbers. The scalb() method ensures that these computations are handled accurately, reducing the risk of errors in financial transactions. Differences Between Math.scalb() and StrictMath.scalb() Java also provides a scalb() method in the Math class. However, there are some differences: 1. Precision vs. Performance StrictMath.scalb() ensures platform-independent results by using precise algorithms. Math.scalb() might use hardware optimizations for better performance but may yield slightly different results on different platforms. 2. Use Case Considerations If you need consistency across all platforms, prefer StrictMath.scalb(). If performance is a priority and slight variations in output are acceptable, Math.scalb() might be the better choice. Key Takeaways The StrictMath scalb() method in Java is used to scale floating-point numbers efficiently. It provides precise results by multiplying the input value by 2^scaleFactor. This method is particularly useful in scientific computing, graphics programming, and financial applications. Unlike regular multiplication, scalb() reduces errors in floating-point arithmetic. StrictMath.scalb() ensures consistent output across different platforms, making it ideal for applications requiring deterministic results. Conclusion The StrictMath scalb() method in Java is a powerful tool for performing efficient floating-point scaling. Whether you are working on scientific simulations, financial models, or game development, this method ensures precision and performance. By understanding its syntax, benefits, and real-world applications, you can enhance your Java programming skills and optimize numerical computations in your projects. If you're exploring Java further, check out more Java tutorials to expand your knowledge and become a more proficient developer.
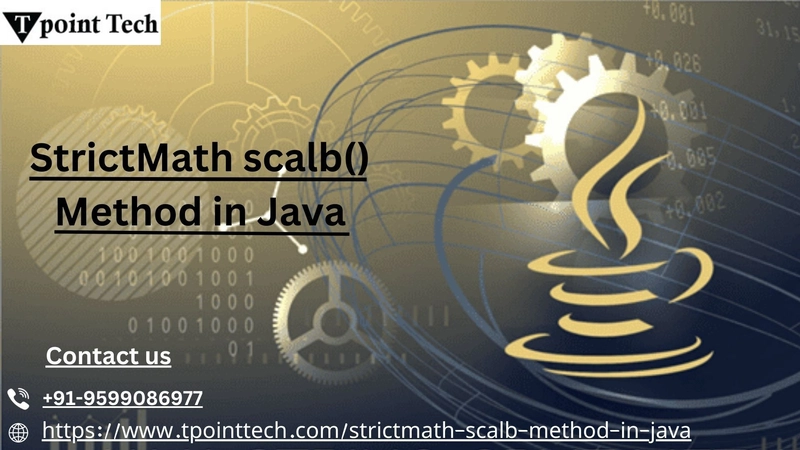
Java provides a variety of mathematical methods to help developers perform precise calculations. Among these, the StrictMath scalb() method in Java is a powerful function that scales floating-point numbers efficiently. This method is particularly useful in scenarios where shifting the binary exponent of a floating-point number is required.
In this blog, we will explore the StrictMath scalb() method in Java, understand its syntax, and discuss practical applications. Whether you are a beginner or an experienced developer, this Java tutorial will help you grasp the importance of this method in mathematical computations.
What is the StrictMath scalb() Method in Java?
The StrictMath scalb() method is part of the StrictMath class, which is known for providing consistent mathematical computations across different platforms. This method scales a floating-point number by a power of two, making it equivalent to multiplying the number by 2^scaleFactor.
Unlike simple multiplication, scalb() ensures precision and efficiency, especially in performance-critical applications such as graphics rendering, scientific computing, and financial calculations.
Syntax of the StrictMath scalb() Method
The StrictMath scalb() method in Java follows this syntax:
- public static double scalb(double d, int scaleFactor)
- public static float scalb(float f, int scaleFactor)
Parameters
- d or f – The floating-point number that needs to be scaled.
- scaleFactor – An integer that represents the power of two by which the floating-point number will be multiplied.
Return Value
- The method returns a floating-point number that is the result of multiplying the input value by 2^scaleFactor.
Why Use the StrictMath scalb() Method?
1. Precision and Accuracy
Unlike traditional multiplication, scalb() is optimized for floating-point calculations, reducing rounding errors that might occur in complex computations.
2. Performance Optimization
Since multiplying by powers of two is a fundamental operation in computing, scalb() is implemented in a way that makes it more efficient than direct multiplication using loops or recursion.
3. Consistency Across Platforms
The StrictMath class ensures that results remain consistent across different hardware and operating systems, which is critical for applications requiring deterministic output.
Real-World Applications
1. Scientific and Engineering Calculations
Many scientific applications involve scaling floating-point numbers efficiently. Whether it's processing sensor data or performing physics simulations, scalb() is a reliable method for such computations.
2. Graphics and Game Development
In graphics rendering, transformations often require adjusting values in powers of two. Using scalb() ensures that these operations are performed efficiently, enhancing performance in real-time applications like video games and simulations.
3. Financial Computations
Many financial models require precise calculations involving large numbers. The scalb() method ensures that these computations are handled accurately, reducing the risk of errors in financial transactions.
Differences Between Math.scalb() and StrictMath.scalb()
Java also provides a scalb() method in the Math class. However, there are some differences:
1. Precision vs. Performance
- StrictMath.scalb() ensures platform-independent results by using precise algorithms.
- Math.scalb() might use hardware optimizations for better performance but may yield slightly different results on different platforms.
2. Use Case Considerations
- If you need consistency across all platforms, prefer StrictMath.scalb().
- If performance is a priority and slight variations in output are acceptable, Math.scalb() might be the better choice.
Key Takeaways
- The StrictMath scalb() method in Java is used to scale floating-point numbers efficiently.
- It provides precise results by multiplying the input value by 2^scaleFactor.
- This method is particularly useful in scientific computing, graphics programming, and financial applications.
- Unlike regular multiplication, scalb() reduces errors in floating-point arithmetic.
- StrictMath.scalb() ensures consistent output across different platforms, making it ideal for applications requiring deterministic results.
Conclusion
The StrictMath scalb() method in Java is a powerful tool for performing efficient floating-point scaling. Whether you are working on scientific simulations, financial models, or game development, this method ensures precision and performance. By understanding its syntax, benefits, and real-world applications, you can enhance your Java programming skills and optimize numerical computations in your projects.
If you're exploring Java further, check out more Java tutorials to expand your knowledge and become a more proficient developer.