Frontend Security Fundamentals Every Developer Should Know
Modern frontend applications are powerful, but that power comes with responsibility. Security is not just a backend concern — your client-side code can and will be exploited if not written with care. This post outlines foundational practices every frontend engineer should understand and apply. 1. Content Security Policy (CSP) A well-configured CSP acts as a gatekeeper against XSS and data injection attacks. Define explicitly what sources are trusted. Avoid unsafe-inline unless absolutely required (and use nonces/hashes if you must). 2. Avoid Dangerous DOM Manipulation Using innerHTML or document.write exposes your app to injection attacks. Instead, prefer DOM-safe methods like textContent, createElement, and appendChild. // Unsafe element.innerHTML = userInput; // Safe element.textContent = userInput; 3. Sanitize External Input Even if the frontend is not directly vulnerable, passing unsanitized input to the backend or displaying it carelessly can compromise integrity. Use libraries like DOMPurify when rendering dynamic HTML from unknown sources: import DOMPurify from 'dompurify'; const cleanHTML = DOMPurify.sanitize(rawHTML); 4. Secure Local Storage Usage Storing sensitive tokens (e.g., JWTs) in localStorage or sessionStorage is discouraged, as they are accessible via JavaScript and vulnerable to XSS. Preferred alternative: HttpOnly cookies, set from the server, inaccessible to JS. 5. Prevent Clickjacking Use proper headers to disallow framing of your site. X-Frame-Options: DENY Content-Security-Policy: frame-ancestors 'none' 6. Dependency Hygiene Supply chain attacks are rising. Audit your packages regularly: npm audit fix npm audit --production Consider using Snyk, Dependabot, or OSS Review Toolkit in CI pipelines. Summary Security is a process, not a product. These practices won’t make your app unbreakable, but they drastically reduce the attack surface. Being proactive with frontend security not only protects users — it protects your reputation.
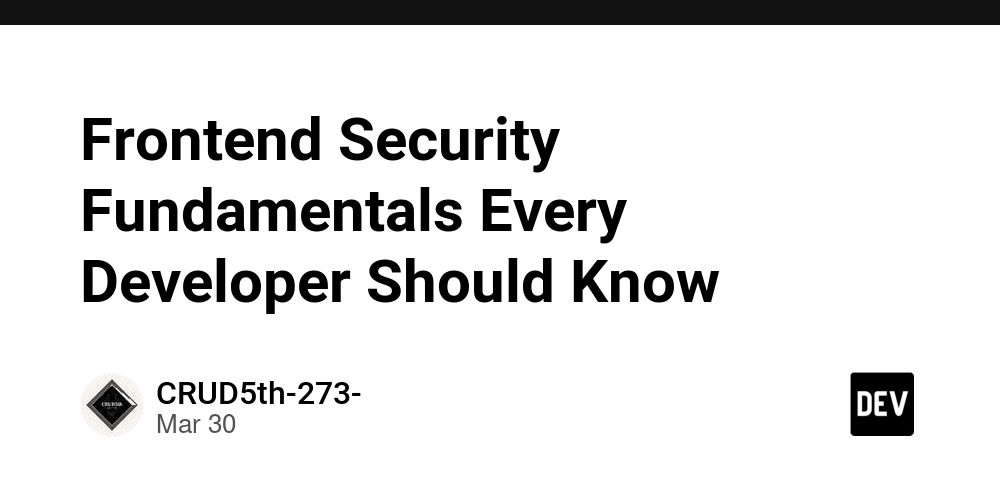
Modern frontend applications are powerful, but that power comes with responsibility.
Security is not just a backend concern — your client-side code can and will be exploited if not written with care.
This post outlines foundational practices every frontend engineer should understand and apply.
1. Content Security Policy (CSP)
A well-configured CSP acts as a gatekeeper against XSS and data injection attacks.
http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self'; object-src 'none';" />
Define explicitly what sources are trusted. Avoid unsafe-inline
unless absolutely required (and use nonces/hashes if you must).
2. Avoid Dangerous DOM Manipulation
Using innerHTML
or document.write
exposes your app to injection attacks.
Instead, prefer DOM-safe methods like textContent
, createElement
, and appendChild
.
// Unsafe
element.innerHTML = userInput;
// Safe
element.textContent = userInput;
3. Sanitize External Input
Even if the frontend is not directly vulnerable, passing unsanitized input to the backend or displaying it carelessly can compromise integrity.
Use libraries like DOMPurify when rendering dynamic HTML from unknown sources:
import DOMPurify from 'dompurify';
const cleanHTML = DOMPurify.sanitize(rawHTML);
4. Secure Local Storage Usage
Storing sensitive tokens (e.g., JWTs) in localStorage
or sessionStorage
is discouraged, as they are accessible via JavaScript and vulnerable to XSS.
Preferred alternative: HttpOnly cookies, set from the server, inaccessible to JS.
5. Prevent Clickjacking
Use proper headers to disallow framing of your site.
X-Frame-Options: DENY
Content-Security-Policy: frame-ancestors 'none'
6. Dependency Hygiene
Supply chain attacks are rising. Audit your packages regularly:
npm audit fix
npm audit --production
Consider using Snyk, Dependabot, or OSS Review Toolkit in CI pipelines.
Summary
Security is a process, not a product.
These practices won’t make your app unbreakable, but they drastically reduce the attack surface.
Being proactive with frontend security not only protects users — it protects your reputation.