Time Durations and Calculations in Go 3/10
The time.Duration Type and How It Works When working with time intervals in Go, understanding the time.Duration type is essential for writing precise, reliable code. Unlike many other languages that represent time as simple integers, Go's standard library provides a specialized type that helps prevent common timing errors while offering powerful functionality. At its core, time.Duration is an int64 that represents a time span in nanoseconds. This might seem like an odd choice at first, but using nanoseconds as the base unit allows Go to represent both very small time intervals (like microseconds) and very large ones (like hours) with a single type. Let's start with a basic example: package main import ( "fmt" "time" ) func main() { // Creating a duration of 5 seconds duration := 5 * time.Second // What's actually stored is nanoseconds fmt.Printf("5 seconds equals %d nanoseconds\n", duration) // We can perform arithmetic with durations twoMinutes := 2 * time.Minute totalTime := duration + twoMinutes fmt.Printf("5 seconds + 2 minutes = %v\n", totalTime) // Durations can be negative too negativeDuration := -1 * time.Hour fmt.Printf("Negative duration: %v\n", negativeDuration) } When you run this code, you'll see output like: 5 seconds equals 5000000000 nanoseconds 5 seconds + 2 minutes = 2m5s Negative duration: -1h0m0s What makes time.Duration especially useful is that Go provides predefined constants that make working with different time units intuitive: const ( Nanosecond Duration = 1 Microsecond = 1000 * Nanosecond Millisecond = 1000 * Microsecond Second = 1000 * Millisecond Minute = 60 * Second Hour = 60 * Minute ) These constants allow you to express time intervals in a way that's both human-readable and precise. Instead of trying to remember how many milliseconds are in an hour, you can simply write 2 * time.Hour and let Go handle the conversion. Another key benefit is type safety. Since time.Duration is its own type, the compiler will prevent you from accidentally mixing it with regular integers in ways that don't make sense. This helps avoid subtle bugs that plague time calculations in many applications. Converting Durations into Different Units (Milliseconds(), Seconds(), etc.) One of the most practical features of Go's time.Duration type is the ability to easily convert between different time units. The time package provides several methods that allow you to extract duration values in specific units, making it straightforward to work with time values in the most appropriate scale for your application. Let's explore these conversion methods with some practical examples: package main import ( "fmt" "time" ) func main() { // Create a complex duration complexDuration := 3*time.Hour + 25*time.Minute + 45*time.Second + 800*time.Millisecond fmt.Printf("Complex duration: %v\n", complexDuration) // Convert to different units hours := complexDuration.Hours() minutes := complexDuration.Minutes() seconds := complexDuration.Seconds() milliseconds := complexDuration.Milliseconds() microseconds := complexDuration.Microseconds() nanoseconds := complexDuration.Nanoseconds() fmt.Printf("Hours: %.2f\n", hours) fmt.Printf("Minutes: %.2f\n", minutes) fmt.Printf("Seconds: %.2f\n", seconds) fmt.Printf("Milliseconds: %d\n", milliseconds) fmt.Printf("Microseconds: %d\n", microseconds) fmt.Printf("Nanoseconds: %d\n", nanoseconds) } This code outputs: Complex duration: 3h25m45.8s Hours: 3.43 Minutes: 205.76 Seconds: 12345.80 Milliseconds: 12345800 Microseconds: 12345800000 Nanoseconds: 12345800000000 Notice a few important details here: The methods .Hours(), .Minutes(), and .Seconds() return float64 values, allowing for fractional units The methods .Milliseconds(), .Microseconds(), and .Nanoseconds() return int64 values When converting to a larger unit (like hours), you get the total duration expressed in that unit (not just the hour component) This flexibility is extremely valuable when building time-sensitive applications. For instance, if you're developing: A performance monitoring tool - you might want millisecond precision A countdown timer for a user interface - seconds might be appropriate A report on long-running processes - hours might be the most readable unit The conversion methods make it easy to retrieve the appropriate value without manual calculation, which reduces the chance of errors. They also permit you to store time internally as a Duration (maintaining precision) while displaying it to users in the most appropriate unit. A common use case is calculating time-based rates: func calculateTransferRate(bytesSent int64, duration time.Duration) float64 { // Convert bytes to megabytes and duration to second
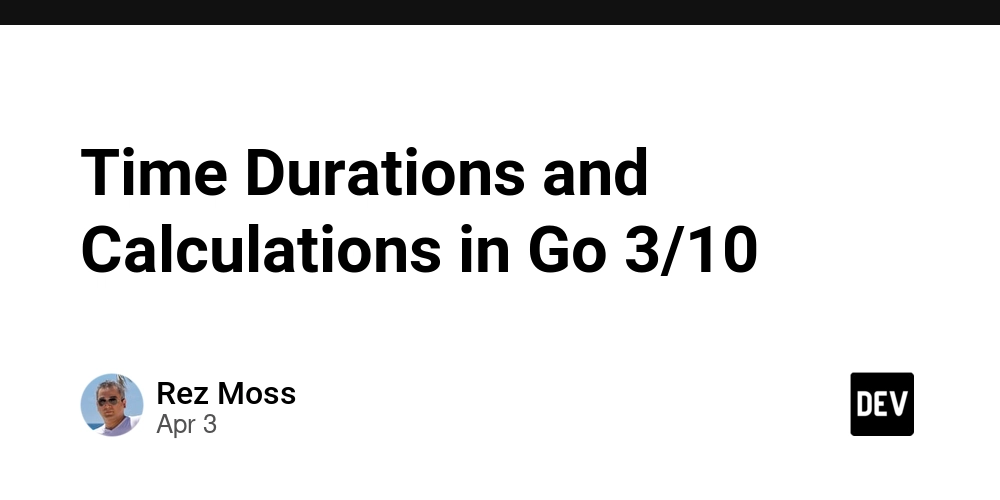
The time.Duration
Type and How It Works
When working with time intervals in Go, understanding the time.Duration
type is essential for writing precise, reliable code. Unlike many other languages that represent time as simple integers, Go's standard library provides a specialized type that helps prevent common timing errors while offering powerful functionality.
At its core, time.Duration
is an int64 that represents a time span in nanoseconds. This might seem like an odd choice at first, but using nanoseconds as the base unit allows Go to represent both very small time intervals (like microseconds) and very large ones (like hours) with a single type.
Let's start with a basic example:
package main
import (
"fmt"
"time"
)
func main() {
// Creating a duration of 5 seconds
duration := 5 * time.Second
// What's actually stored is nanoseconds
fmt.Printf("5 seconds equals %d nanoseconds\n", duration)
// We can perform arithmetic with durations
twoMinutes := 2 * time.Minute
totalTime := duration + twoMinutes
fmt.Printf("5 seconds + 2 minutes = %v\n", totalTime)
// Durations can be negative too
negativeDuration := -1 * time.Hour
fmt.Printf("Negative duration: %v\n", negativeDuration)
}
When you run this code, you'll see output like:
5 seconds equals 5000000000 nanoseconds
5 seconds + 2 minutes = 2m5s
Negative duration: -1h0m0s
What makes time.Duration
especially useful is that Go provides predefined constants that make working with different time units intuitive:
const (
Nanosecond Duration = 1
Microsecond = 1000 * Nanosecond
Millisecond = 1000 * Microsecond
Second = 1000 * Millisecond
Minute = 60 * Second
Hour = 60 * Minute
)
These constants allow you to express time intervals in a way that's both human-readable and precise. Instead of trying to remember how many milliseconds are in an hour, you can simply write 2 * time.Hour
and let Go handle the conversion.
Another key benefit is type safety. Since time.Duration
is its own type, the compiler will prevent you from accidentally mixing it with regular integers in ways that don't make sense. This helps avoid subtle bugs that plague time calculations in many applications.
Converting Durations into Different Units (Milliseconds()
, Seconds()
, etc.)
One of the most practical features of Go's time.Duration
type is the ability to easily convert between different time units. The time
package provides several methods that allow you to extract duration values in specific units, making it straightforward to work with time values in the most appropriate scale for your application.
Let's explore these conversion methods with some practical examples:
package main
import (
"fmt"
"time"
)
func main() {
// Create a complex duration
complexDuration := 3*time.Hour + 25*time.Minute + 45*time.Second + 800*time.Millisecond
fmt.Printf("Complex duration: %v\n", complexDuration)
// Convert to different units
hours := complexDuration.Hours()
minutes := complexDuration.Minutes()
seconds := complexDuration.Seconds()
milliseconds := complexDuration.Milliseconds()
microseconds := complexDuration.Microseconds()
nanoseconds := complexDuration.Nanoseconds()
fmt.Printf("Hours: %.2f\n", hours)
fmt.Printf("Minutes: %.2f\n", minutes)
fmt.Printf("Seconds: %.2f\n", seconds)
fmt.Printf("Milliseconds: %d\n", milliseconds)
fmt.Printf("Microseconds: %d\n", microseconds)
fmt.Printf("Nanoseconds: %d\n", nanoseconds)
}
This code outputs:
Complex duration: 3h25m45.8s
Hours: 3.43
Minutes: 205.76
Seconds: 12345.80
Milliseconds: 12345800
Microseconds: 12345800000
Nanoseconds: 12345800000000
Notice a few important details here:
- The methods
.Hours()
,.Minutes()
, and.Seconds()
returnfloat64
values, allowing for fractional units - The methods
.Milliseconds()
,.Microseconds()
, and.Nanoseconds()
returnint64
values - When converting to a larger unit (like hours), you get the total duration expressed in that unit (not just the hour component)
This flexibility is extremely valuable when building time-sensitive applications. For instance, if you're developing:
- A performance monitoring tool - you might want millisecond precision
- A countdown timer for a user interface - seconds might be appropriate
- A report on long-running processes - hours might be the most readable unit
The conversion methods make it easy to retrieve the appropriate value without manual calculation, which reduces the chance of errors. They also permit you to store time internally as a Duration
(maintaining precision) while displaying it to users in the most appropriate unit.
A common use case is calculating time-based rates:
func calculateTransferRate(bytesSent int64, duration time.Duration) float64 {
// Convert bytes to megabytes and duration to seconds
megabytes := float64(bytesSent) / 1024 / 1024
seconds := duration.Seconds()
// Return MB/s
return megabytes / seconds
}
These conversion methods ensure you don't need to remember conversion factors or manually implement time unit conversions, making your code both more readable and more maintainable.
Creating Custom Durations for Calculations
Working with custom time durations is a common need in many applications, from implementing timeouts to scheduling tasks. Go's time.Duration
type makes this straightforward while maintaining type safety and readability.
Let's explore several approaches to creating and working with custom durations:
package main
import (
"fmt"
"time"
)
func main() {
// Method 1: Using predefined constants
timeout := 30 * time.Second
pollingInterval := 250 * time.Millisecond
// Method 2: Building composite durations
meetingLength := 1*time.Hour + 30*time.Minute
// Method 3: From numeric values
customSeconds := time.Duration(45) * time.Second
// Method 4: From floating-point values (careful with precision!)
hoursAsFloat := 1.75
customHours := time.Duration(hoursAsFloat * float64(time.Hour))
// Display our custom durations
fmt.Printf("Timeout: %v\n", timeout)
fmt.Printf("Polling interval: %v\n", pollingInterval)
fmt.Printf("Meeting length: %v\n", meetingLength)
fmt.Printf("Custom seconds: %v\n", customSeconds)
fmt.Printf("Custom hours (from float): %v\n", customHours)
}
Output:
Timeout: 30s
Polling interval: 250ms
Meeting length: 1h30m0s
Custom seconds: 45s
Custom hours (from float): 1h45m0s
When working with custom durations, you'll often need to perform calculations with them. Go makes this intuitive by supporting arithmetic operations directly on the Duration
type:
func durationCalculations() {
baseDuration := 1 * time.Minute
// Addition and subtraction
extendedDuration := baseDuration + 30*time.Second
shortenedDuration := baseDuration - 15*time.Second
// Multiplication and division with scalars
doubleDuration := baseDuration * 2
halfDuration := baseDuration / 2
fmt.Printf("Base: %v\n", baseDuration)
fmt.Printf("Extended: %v\n", extendedDuration)
fmt.Printf("Shortened: %v\n", shortenedDuration)
fmt.Printf("Double: %v\n", doubleDuration)
fmt.Printf("Half: %v\n", halfDuration)
// Comparing durations
timeout := 5 * time.Second
operationTime := 3 * time.Second
if operationTime < timeout {
fmt.Println("Operation completed within timeout")
}
// Finding the larger/smaller of two durations
fmt.Printf("Max duration: %v\n", max(timeout, operationTime))
fmt.Printf("Min duration: %v\n", min(timeout, operationTime))
}
// Go 1.21+ has built-in min/max, but for earlier versions:
func max(a, b time.Duration) time.Duration {
if a > b {
return a
}
return b
}
func min(a, b time.Duration) time.Duration {
if a < b {
return a
}
return b
}
One particularly useful pattern is creating helper functions that work with durations for domain-specific calculations:
// Calculate retry backoff with exponential increase
func calculateBackoff(attempt int, baseDuration time.Duration, maxDuration time.Duration) time.Duration {
// Calculate exponential backoff: baseDuration * 2^attempt
backoff := baseDuration * time.Duration(1<<attempt) // 1<
// Cap at maximum duration
if backoff > maxDuration {
return maxDuration
}
return backoff
}
func demonstrateBackoff() {
base := 100 * time.Millisecond
max := 10 * time.Second
for attempt := 0; attempt < 8; attempt++ {
backoff := calculateBackoff(attempt, base, max)
fmt.Printf("Attempt %d: retry after %v\n", attempt, backoff)
}
}
Custom durations are also invaluable when dealing with rate limiting, scheduling, and implementing timeout logic. The type safety of time.Duration
helps prevent errors that would be common with simple integer representations of time.
Rounding and Truncating Time Values (Round()
, Truncate()
)
When working with time values in Go, you'll often need to adjust the precision of your timestamps or durations. The time
package provides two key methods for this purpose: Round()
and Truncate()
. Despite their similar-sounding names, they serve distinct functions that are important to understand.
Let's start by examining how these methods work with the time.Duration
type:
package main
import (
"fmt"
"time"
)
func main() {
// Create a precise duration
preciseDuration := 1*time.Hour + 23*time.Minute + 45*time.Second + 678*time.Millisecond
fmt.Printf("Original duration: %v\n", preciseDuration)
// Round to different units
roundToSecond := preciseDuration.Round(time.Second)
roundToMinute := preciseDuration.Round(time.Minute)
roundTo5Minutes := preciseDuration.Round(5 * time.Minute)
fmt.Printf("Rounded to nearest second: %v\n", roundToSecond)
fmt.Printf("Rounded to nearest minute: %v\n", roundToMinute)
fmt.Printf("Rounded to nearest 5 minutes: %v\n", roundTo5Minutes)
// Truncate to different units
truncateToSecond := preciseDuration.Truncate(time.Second)
truncateToMinute := preciseDuration.Truncate(time.Minute)
truncateTo15Minutes := preciseDuration.Truncate(15 * time.Minute)
fmt.Printf("Truncated to second: %v\n", truncateToSecond)
fmt.Printf("Truncated to minute: %v\n", truncateToMinute)
fmt.Printf("Truncated to 15 minutes: %v\n", truncateTo15Minutes)
}
Running this code will produce output similar to:
Original duration: 1h23m45.678s
Rounded to nearest second: 1h23m46s
Rounded to nearest minute: 1h24m0s
Rounded to nearest 5 minutes: 1h25m0s
Truncated to second: 1h23m45s
Truncated to minute: 1h23m0s
Truncated to 15 minutes: 1h15m0s
The key differences between Round()
and Truncate()
are:
Round() adjusts the duration to the nearest multiple of the specified unit. It follows standard rounding rules: if the remainder is less than half the unit, it rounds down; otherwise, it rounds up.
Truncate() simply removes any component smaller than the specified unit, effectively rounding down to the nearest multiple of that unit.
These methods are also available for time.Time
objects, which is particularly useful for aligning timestamps to specific boundaries:
func timeRoundingExample() {
// Get the current time
now := time.Now()
fmt.Printf("Current time: %v\n", now.Format("15:04:05.000"))
// Round to different units
roundedToSecond := now.Round(time.Second)
roundedToMinute := now.Round(time.Minute)
fmt.Printf("Rounded to second: %v\n", roundedToSecond.Format("15:04:05.000"))
fmt.Printf("Rounded to minute: %v\n", roundedToMinute.Format("15:04:05.000"))
// Truncate to different units
truncatedToSecond := now.Truncate(time.Second)
truncatedToMinute := now.Truncate(time.Minute)
truncatedToHour := now.Truncate(time.Hour)
fmt.Printf("Truncated to second: %v\n", truncatedToSecond.Format("15:04:05.000"))
fmt.Printf("Truncated to minute: %v\n", truncatedToMinute.Format("15:04:05.000"))
fmt.Printf("Truncated to hour: %v\n", truncatedToHour.Format("15:04:05.000"))
}
These rounding and truncating operations are essential for various practical applications:
- Log aggregation: Grouping log entries by minute or hour
- Time-series data: Aligning data points to consistent intervals
- Scheduling: Setting task execution times to specific boundaries
- UI display: Showing simplified time representations
- Rate limiting: Implementing time-window-based rate controls
Here's a real-world example of using these methods to implement a simple time-bucketing function:
// Group events into time buckets of specified size
func timeBucket(eventTime time.Time, bucketSize time.Duration) time.Time {
return eventTime.Truncate(bucketSize)
}
func demoTimeBucketing() {
events := []time.Time{
time.Date(2023, 6, 15, 10, 15, 30, 0, time.UTC),
time.Date(2023, 6, 15, 10, 18, 45, 0, time.UTC),
time.Date(2023, 6, 15, 10, 32, 10, 0, time.UTC),
time.Date(2023, 6, 15, 11, 05, 20, 0, time.UTC),
}
// Group by 15-minute buckets
bucketSize := 15 * time.Minute
buckets := make(map[time.Time]int)
for _, event := range events {
bucket := timeBucket(event, bucketSize)
buckets[bucket]++
}
// Print the buckets and counts
for bucket, count := range buckets {
fmt.Printf("Bucket %v: %d events\n", bucket.Format("15:04"), count)
}
}
Understanding when to use Round()
versus Truncate()
is crucial for accurate time-based operations in your Go applications.
Parsing Duration Strings with ParseDuration()
Working with time durations often involves dealing with human-readable text representations of time intervals. In Go, the time.ParseDuration()
function provides a powerful way to convert textual time descriptions into the time.Duration
type. This function makes it easy to handle user input, configuration values, or command-line arguments that specify time durations.
Let's examine how to use this function effectively:
package main
import (
"fmt"
"time"
)
func main() {
// Basic duration parsing
duration, err := time.ParseDuration("1h30m")
if err != nil {
fmt.Printf("Error parsing duration: %v\n", err)
return
}
fmt.Printf("Parsed duration: %v (%d nanoseconds)\n", duration, duration.Nanoseconds())
// Parse more complex durations
examples := []string{
"300ms",
"1.5h",
"2h45m15s",
"1h30m300ms",
"30s500ns",
"-10m", // Negative durations are supported
"1.5d", // This will fail - days aren't supported
"1h invalid", // This will fail - invalid format
}
for _, example := range examples {
d, err := time.ParseDuration(example)
if err != nil {
fmt.Printf("'%s' -> Error: %v\n", example, err)
} else {
fmt.Printf("'%s' -> %v (%s)\n", example, d, formatDurationComponents(d))
}
}
}
// Helper function to show the component parts of a duration
func formatDurationComponents(d time.Duration) string {
hours := int(d.Hours())
minutes := int(d.Minutes()) % 60
seconds := int(d.Seconds()) % 60
milliseconds := int(d.Milliseconds()) % 1000
return fmt.Sprintf("%dh %dm %ds %dms", hours, minutes, seconds, milliseconds)
}
When run, this code produces output similar to:
Parsed duration: 1h30m0s (5400000000000 nanoseconds)
'300ms' -> 300ms (0h 0m 0s 300ms)
'1.5h' -> 1h30m0s (1h 30m 0s 0ms)
'2h45m15s' -> 2h45m15s (2h 45m 15s 0ms)
'1h30m300ms' -> 1h30m0.3s (1h 30m 0s 300ms)
'30s500ns' -> 30.0000005s (0h 0m 30s 0ms)
'-10m' -> -10m0s (0h -10m 0s 0ms)
'1.5d' -> Error: time: unknown unit "d" in duration "1.5d"
'1h invalid' -> Error: time: invalid duration "1h invalid"
Here are some key points about ParseDuration()
:
Supported units: The function supports nanoseconds (
ns
), microseconds (µs
orus
), milliseconds (ms
), seconds (s
), minutes (m
), and hours (h
). Notably, there's no built-in support for days, weeks, months, or years.Format requirements: The format must be a sequence of decimal numbers, each with an optional fraction and a unit suffix. For example, "300ms", "1.5h", or "2h45m". No spaces are allowed between the number and unit.
Error handling: Always check the error return value. The function returns an error for any invalid input.
Fractional units: You can use decimal points with any unit (e.g., "1.5h").
Negative durations: Prepending a minus sign creates a negative duration, useful for time calculations involving the past.
A common pattern is to provide default values when parsing potentially invalid duration strings:
func parseDurationWithDefault(input string, defaultDuration time.Duration) time.Duration {
duration, err := time.ParseDuration(input)
if err != nil {
return defaultDuration
}
return duration
}
func configExample() {
// Simulating config values from environment or file
timeoutStr := "30s"
retryIntervalStr := "invalid"
// Parse with defaults
timeout := parseDurationWithDefault(timeoutStr, 10*time.Second)
retryInterval := parseDurationWithDefault(retryIntervalStr, 5*time.Second)
fmt.Printf("Using timeout: %v\n", timeout)
fmt.Printf("Using retry interval: %v\n", retryInterval)
}
For more complex use cases, you might need to build your own parser for non-standard formats or units. Here's a simple example that handles days:
func parseDurationWithDays(input string) (time.Duration, error) {
// Check if the string contains a day component
if len(input) > 1 && input[len(input)-1] == 'd' {
// Try to parse the day value
dayValue, err := strconv.ParseFloat(input[:len(input)-1], 64)
if err != nil {
return 0, fmt.Errorf("invalid day value: %w", err)
}
// Convert days to hours and parse
hourStr := fmt.Sprintf("%.2fh", dayValue*24)
return time.ParseDuration(hourStr)
}
// No day component, use standard parsing
return time.ParseDuration(input)
}
The ParseDuration()
function, combined with the other features of time.Duration
, provides a robust foundation for working with time intervals in Go applications. Whether you're implementing timeouts, rate limiters, caching, or scheduling logic, these tools make it easier to write clear, correct, and maintainable time-based code.