Understanding Condition Coverage in Software Testing
Condition coverage is a crucial metric in software testing that helps ensure every logical condition in your code has been evaluated both to true and false. In this post, we’ll explore what condition coverage means, how it differs from other types of test coverage, and why it’s vital for reliable software. What is Condition Coverage? Condition coverage is a type of code coverage that checks whether each boolean sub-expression in a decision has been tested for both true and false outcomes. For example, in a condition like if (A && B), condition coverage ensures that both A and B are independently evaluated as true and false during test execution, even if the overall condition's result remains the same. Why is Condition Coverage Important? Condition coverage ensures that individual logical conditions are tested independently, reducing the chances of hidden bugs. Many defects in software originate from overlooked logical branches or improperly evaluated boolean conditions. By focusing on these individual parts, condition coverage helps detect bugs that could otherwise go unnoticed with broader metrics like statement or decision coverage. How Condition Coverage Works In a conditional expression like if (A || B), condition coverage checks if both A and B can independently be true or false. This is done regardless of the final result of the condition. For instance, if A is true and the condition short-circuits, a traditional decision coverage metric may miss testing B entirely. Condition coverage avoids this by requiring that each operand be tested both ways. Condition Coverage vs. Decision Coverage While decision coverage ensures that the entire decision evaluates to true and false, it does not guarantee that every condition within that decision is independently tested. Condition coverage provides deeper insight by verifying that each boolean expression within a decision behaves correctly. For example: java CopyEdit if (A && B) Decision Coverage: Ensures the entire if condition is true and false. Condition Coverage: Ensures A is true/false and B is true/false — independently. This makes condition coverage more thorough in catching logic-related errors. Benefits of Using Condition Coverage Condition coverage provides several key benefits: Enhanced logic validation: Tests the smallest parts of decision logic. Improved defect detection: Helps identify bugs hiding in compound conditions. Increased code confidence: Gives developers a clearer view of what’s been thoroughly tested. Better maintainability: Encourages writing modular, testable code by focusing on logic clarity. Limitations of Condition Coverage Although condition coverage is powerful, it doesn’t test all possible combinations of condition outcomes. For that, you would need multiple condition coverage or MC/DC (Modified Condition/Decision Coverage). These offer deeper granularity but also introduce complexity and increased testing effort. Condition coverage is a practical middle ground between minimal and exhaustive testing. How to Measure Condition Coverage You can measure condition coverage using automated testing tools that support this metric. These tools analyze test executions and determine whether each condition was evaluated to both true and false. Tools like JaCoCo (Java), Istanbul (JavaScript), and gcov (C/C++) provide condition coverage metrics in their reports. Developers can integrate these tools with CI pipelines to continuously monitor and improve coverage. Tools that Support Condition Coverage Here are some popular tools that provide condition coverage capabilities: JaCoCo (Java) – Supports condition and branch coverage. Istanbul/nyc (JavaScript) – Offers branch and condition coverage. gcov (C/C++) – Offers detailed condition and branch analysis. Coverage.py (Python) – Tracks branch conditions with add-ons. Cobertura (Java) – Provides condition coverage at a basic level. Each tool varies in how it defines and reports condition coverage, so it’s important to choose one that fits your language and build environment. Best Practices for Implementing Condition Coverage To effectively implement condition coverage: Write focused unit tests that isolate individual conditions. Avoid overly complex conditions; break them into smaller logical units. Use mocks and stubs to control test inputs and simulate edge cases. Automate coverage reports within your CI pipeline to enforce coverage goals. Review untested conditions regularly to ensure critical logic is fully validated. Conclusion Condition coverage plays a key role in writing high-quality, testable code by focusing on individual logical conditions. It helps identify hidden logic flaws, improve test completeness, and boost confidence in your codebase. When combined with other coverage metrics like statement and decision coverage, it forms a strong foundation for a robust testing strategy.
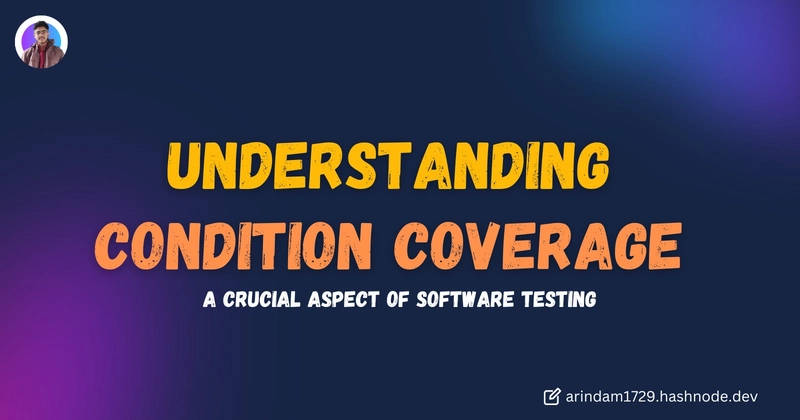
Condition coverage is a crucial metric in software testing that helps ensure every logical condition in your code has been evaluated both to true and false. In this post, we’ll explore what condition coverage means, how it differs from other types of test coverage, and why it’s vital for reliable software.
What is Condition Coverage?
Condition coverage is a type of code coverage that checks whether each boolean sub-expression in a decision has been tested for both true and false outcomes. For example, in a condition like if (A && B), condition coverage ensures that both A and B are independently evaluated as true and false during test execution, even if the overall condition's result remains the same.
Why is Condition Coverage Important?
Condition coverage ensures that individual logical conditions are tested independently, reducing the chances of hidden bugs. Many defects in software originate from overlooked logical branches or improperly evaluated boolean conditions. By focusing on these individual parts, condition coverage helps detect bugs that could otherwise go unnoticed with broader metrics like statement or decision coverage.
How Condition Coverage Works
In a conditional expression like if (A || B), condition coverage checks if both A and B can independently be true or false. This is done regardless of the final result of the condition. For instance, if A is true and the condition short-circuits, a traditional decision coverage metric may miss testing B entirely. Condition coverage avoids this by requiring that each operand be tested both ways.
Condition Coverage vs. Decision Coverage
While decision coverage ensures that the entire decision evaluates to true and false, it does not guarantee that every condition within that decision is independently tested. Condition coverage provides deeper insight by verifying that each boolean expression within a decision behaves correctly. For example:
java
CopyEdit
if (A && B)
- Decision Coverage: Ensures the entire if condition is true and false.
- Condition Coverage: Ensures A is true/false and B is true/false — independently.
This makes condition coverage more thorough in catching logic-related errors.
Benefits of Using Condition Coverage
Condition coverage provides several key benefits:
- Enhanced logic validation: Tests the smallest parts of decision logic.
- Improved defect detection: Helps identify bugs hiding in compound conditions.
- Increased code confidence: Gives developers a clearer view of what’s been thoroughly tested.
- Better maintainability: Encourages writing modular, testable code by focusing on logic clarity.
Limitations of Condition Coverage
Although condition coverage is powerful, it doesn’t test all possible combinations of condition outcomes. For that, you would need multiple condition coverage or MC/DC (Modified Condition/Decision Coverage). These offer deeper granularity but also introduce complexity and increased testing effort. Condition coverage is a practical middle ground between minimal and exhaustive testing.
How to Measure Condition Coverage
You can measure condition coverage using automated testing tools that support this metric. These tools analyze test executions and determine whether each condition was evaluated to both true and false. Tools like JaCoCo (Java), Istanbul (JavaScript), and gcov (C/C++) provide condition coverage metrics in their reports. Developers can integrate these tools with CI pipelines to continuously monitor and improve coverage.
Tools that Support Condition Coverage
Here are some popular tools that provide condition coverage capabilities:
- JaCoCo (Java) – Supports condition and branch coverage.
- Istanbul/nyc (JavaScript) – Offers branch and condition coverage.
- gcov (C/C++) – Offers detailed condition and branch analysis.
- Coverage.py (Python) – Tracks branch conditions with add-ons.
- Cobertura (Java) – Provides condition coverage at a basic level.
Each tool varies in how it defines and reports condition coverage, so it’s important to choose one that fits your language and build environment.
Best Practices for Implementing Condition Coverage
To effectively implement condition coverage:
- Write focused unit tests that isolate individual conditions.
- Avoid overly complex conditions; break them into smaller logical units.
- Use mocks and stubs to control test inputs and simulate edge cases.
- Automate coverage reports within your CI pipeline to enforce coverage goals.
- Review untested conditions regularly to ensure critical logic is fully validated.
Conclusion
Condition coverage plays a key role in writing high-quality, testable code by focusing on individual logical conditions. It helps identify hidden logic flaws, improve test completeness, and boost confidence in your codebase. When combined with other coverage metrics like statement and decision coverage, it forms a strong foundation for a robust testing strategy.