Day 2 JAVA OOps, Sample Program
Java Object-Oriented Programming (OOPs) Concepts Object-Oriented Programming (OOP) is a programming paradigm that organizes code into objects, making it more modular, reusable, and scalable. Java is one of the most popular OOP-based languages. Let's explore its core concepts! 1. Class and Object Class A class is a blueprint for creating objects. It defines properties (variables) and behaviors (methods) that objects of that class will have. class Car { String brand; int speed; void display() { System.out.println("Brand: " + brand + ", Speed: " + speed); } } Object An object is an instance of a class. It holds actual values for the properties defined in the class. public class Main { public static void main(String[] args) { Car myCar = new Car(); // Creating an object myCar.brand = "Toyota"; myCar.speed = 120; myCar.display(); } } 2. Encapsulation Encapsulation is the principle of wrapping data (variables) and code (methods) into a single unit and restricting direct access to them. class BankAccount { private double balance; public void deposit(double amount) { balance += amount; } public double getBalance() { return balance; } } Benefits of Encapsulation: Protects data from unauthorized access Maintains data integrity Improves code reusability 3. Inheritance Inheritance allows one class to acquire the properties and behaviors of another class, promoting reusability. class Animal { void eat() { System.out.println("This animal eats food."); } } class Dog extends Animal { void bark() { System.out.println("Dog barks"); } } public class Main { public static void main(String[] args) { Dog myDog = new Dog(); myDog.eat(); // Inherited from Animal myDog.bark(); // Defined in Dog } } Types of Inheritance in Java: Single Inheritance: One class inherits from another. Multilevel Inheritance: A derived class acts as a base class for another class. Hierarchical Inheritance: Multiple classes inherit from the same base class. Java does not support multiple inheritance (using classes) to avoid ambiguity, but it can be achieved with interfaces. 4. Polymorphism Polymorphism allows methods to perform different behaviors based on the object calling them. Method Overloading (Compile-time Polymorphism) Same method name, different parameters. class MathOperations { int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; } } Method Overriding (Runtime Polymorphism) Child class provides a specific implementation of a method from the parent class. class Parent { void show() { System.out.println("Parent's show method"); } } class Child extends Parent { @Override void show() { System.out.println("Child's show method"); } } 5. Abstraction Abstraction hides implementation details and only exposes necessary functionality. Abstract Class abstract class Vehicle { abstract void start(); // Abstract method } class Bike extends Vehicle { void start() { System.out.println("Bike is starting..."); } } Interface An interface defines a contract that classes must follow. interface Animal { void makeSound(); // Abstract method } class Cat implements Animal { public void makeSound() { System.out.println("Meow!"); } } Key Differences: Abstract Class vs Interface Feature Abstract Class Interface Methods Can have both abstract and concrete methods Only abstract methods (until Java 8) Variables Can have instance variables Only constants (final, static) Inheritance Single inheritance Multiple inheritance Conclusion Java's Object-Oriented Programming (OOP) principles help in designing scalable, reusable, and maintainable applications Here the sample java program Common Mistakes and Fixes 1. Wrong Class Name If your file is named Hello.java, the class name must be Hello. ❌ Wrong: public class HelloWorld { // Class name does not match the filename public static void main(String[] args) { System.out.println("Hello, Java!"); } } ✅ Fix: Rename the class to match the filename OR rename the file to HelloWorld.java. 2. Missing main Method The Java Virtual Machine (JVM) needs a main method to run the program. ❌ Wrong: public class Hello { // No main method } ✅ Fix: Add the main method: public class Hello { public static void main(String[] args) { System.out.println("Hello, Java!"); } } 3. Incorrect main Method Signature The main method must be public, static, and have String
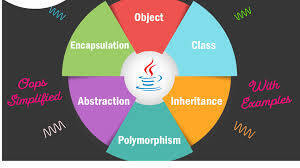
Java Object-Oriented Programming (OOPs) Concepts
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into objects, making it more modular, reusable, and scalable. Java is one of the most popular OOP-based languages. Let's explore its core concepts!
1. Class and Object
Class
A class is a blueprint for creating objects. It defines properties (variables) and behaviors (methods) that objects of that class will have.
class Car {
String brand;
int speed;
void display() {
System.out.println("Brand: " + brand + ", Speed: " + speed);
}
}
Object
An object is an instance of a class. It holds actual values for the properties defined in the class.
public class Main {
public static void main(String[] args) {
Car myCar = new Car(); // Creating an object
myCar.brand = "Toyota";
myCar.speed = 120;
myCar.display();
}
}
2. Encapsulation
Encapsulation is the principle of wrapping data (variables) and code (methods) into a single unit and restricting direct access to them.
class BankAccount {
private double balance;
public void deposit(double amount) {
balance += amount;
}
public double getBalance() {
return balance;
}
}
Benefits of Encapsulation:
- Protects data from unauthorized access
- Maintains data integrity
- Improves code reusability
3. Inheritance
Inheritance allows one class to acquire the properties and behaviors of another class, promoting reusability.
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat(); // Inherited from Animal
myDog.bark(); // Defined in Dog
}
}
Types of Inheritance in Java:
- Single Inheritance: One class inherits from another.
- Multilevel Inheritance: A derived class acts as a base class for another class.
- Hierarchical Inheritance: Multiple classes inherit from the same base class.
- Java does not support multiple inheritance (using classes) to avoid ambiguity, but it can be achieved with interfaces.
4. Polymorphism
Polymorphism allows methods to perform different behaviors based on the object calling them.
Method Overloading (Compile-time Polymorphism)
Same method name, different parameters.
class MathOperations {
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
}
Method Overriding (Runtime Polymorphism)
Child class provides a specific implementation of a method from the parent class.
class Parent {
void show() {
System.out.println("Parent's show method");
}
}
class Child extends Parent {
@Override
void show() {
System.out.println("Child's show method");
}
}
5. Abstraction
Abstraction hides implementation details and only exposes necessary functionality.
Abstract Class
abstract class Vehicle {
abstract void start(); // Abstract method
}
class Bike extends Vehicle {
void start() {
System.out.println("Bike is starting...");
}
}
Interface
An interface defines a contract that classes must follow.
interface Animal {
void makeSound(); // Abstract method
}
class Cat implements Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
Key Differences: Abstract Class vs Interface
Feature | Abstract Class | Interface |
---|---|---|
Methods | Can have both abstract and concrete methods | Only abstract methods (until Java 8) |
Variables | Can have instance variables | Only constants (final, static) |
Inheritance | Single inheritance | Multiple inheritance |
Conclusion
Java's Object-Oriented Programming (OOP) principles help in designing scalable, reusable, and maintainable applications
Here the sample java program
Common Mistakes and Fixes
1. Wrong Class Name
If your file is named Hello.java
, the class name must be Hello
.
❌ Wrong:
public class HelloWorld { // Class name does not match the filename
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
✅ Fix:
Rename the class to match the filename OR rename the file to HelloWorld.java
.
2. Missing main
Method
The Java Virtual Machine (JVM) needs a main
method to run the program.
❌ Wrong:
public class Hello {
// No main method
}
✅ Fix:
Add the main
method:
public class Hello {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
3. Incorrect main
Method Signature
The main
method must be public
, static
, and have String[] args
.
❌ Wrong:
public class Hello {
void main() { // Missing 'public static' and incorrect parameters
System.out.println("Hello, Java!");
}
}
✅ Fix:
public class Hello {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
4. Missing Semicolon (;
)
Java statements must end with a semicolon.
❌ Wrong:
public class Hello {
public static void main(String[] args) {
System.out.println("Hello, Java!") // Missing semicolon
}
}
✅ Fix:
public class Hello {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
5. Compilation and Execution Mistakes
If your file is Hello.java
, compile and run it properly:
javac Hello.java # Compile
java Hello # Run (without .class or .java)
If you run:
java Hello.java # ❌ Wrong
You'll get an error like:
Error: Could not find or load main class Hello.java