➡️ 7 Effective JavaScript Debugging Techniques Every Developer Should Know
Debugging JavaScript effectively can save countless hours and significantly reduce frustration. Here are some proven techniques and tools to debug your JavaScript efficiently and effectively. 1. Console Logging The simplest yet powerful way to debug JavaScript is by logging messages, objects, or variables to the console. console.log("Value:", value); console.error("Error occurred", error); console.table(arrayOfObjects); 2. Using Breakpoints in Developer Tools Breakpoints allow you to pause code execution at specific points and inspect variables or step through the code line-by-line. Chrome DevTools: Go to Sources → Click on a line number to set a breakpoint. VSCode: Click in the gutter next to the code line to toggle breakpoints. 3. Debugger Statement Insert a debugger statement directly into your JavaScript code to automatically trigger a breakpoint when the developer tools are open. function problematicFunction() { debugger; // Code to debug let result = complexCalculation(); console.log(result); } 4. Source Maps for Debugging Minified Code Use source maps to debug compressed or transpiled JavaScript effectively. Source maps connect minified code back to the original source files. Webpack Example: // webpack.config.js module.exports = { devtool: 'source-map' }; 5. Network Tab for Debugging API Calls Inspect network requests to verify API responses, check request parameters, and debug network issues effectively. Open DevTools → Network Tab. Inspect headers, payloads, response status codes, and timings. 6. Call Stack and Scope Inspection The call stack lets you track the sequence of function calls, while inspecting scope helps you understand the current state of variables. Example: function first() { second(); } function second() { third(); } function third() { console.trace(); // prints the call stack } first(); 7. Conditional Breakpoints Set breakpoints that activate only under specific conditions, making your debugging more precise. Chrome DevTools: Right-click on breakpoint → Edit breakpoint → Set condition (e.g., i === 10). Example: for(let i = 0; i
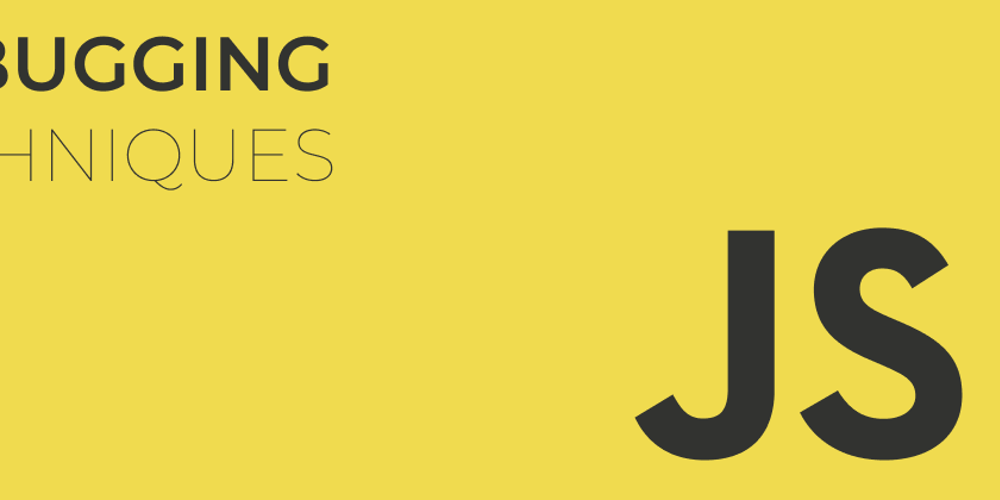
Debugging JavaScript effectively can save countless hours and significantly reduce frustration. Here are some proven techniques and tools to debug your JavaScript efficiently and effectively.
1. Console Logging
The simplest yet powerful way to debug JavaScript is by logging messages, objects, or variables to the console.
console.log("Value:", value);
console.error("Error occurred", error);
console.table(arrayOfObjects);
2. Using Breakpoints in Developer Tools
Breakpoints allow you to pause code execution at specific points and inspect variables or step through the code line-by-line.
- Chrome DevTools: Go to Sources → Click on a line number to set a breakpoint.
- VSCode: Click in the gutter next to the code line to toggle breakpoints.
3. Debugger Statement
Insert a debugger
statement directly into your JavaScript code to automatically trigger a breakpoint when the developer tools are open.
function problematicFunction() {
debugger;
// Code to debug
let result = complexCalculation();
console.log(result);
}
4. Source Maps for Debugging Minified Code
Use source maps to debug compressed or transpiled JavaScript effectively. Source maps connect minified code back to the original source files.
Webpack Example:
// webpack.config.js
module.exports = {
devtool: 'source-map'
};
5. Network Tab for Debugging API Calls
Inspect network requests to verify API responses, check request parameters, and debug network issues effectively.
- Open DevTools → Network Tab.
- Inspect headers, payloads, response status codes, and timings.
6. Call Stack and Scope Inspection
The call stack lets you track the sequence of function calls, while inspecting scope helps you understand the current state of variables.
Example:
function first() {
second();
}
function second() {
third();
}
function third() {
console.trace(); // prints the call stack
}
first();
7. Conditional Breakpoints
Set breakpoints that activate only under specific conditions, making your debugging more precise.
-
Chrome DevTools: Right-click on breakpoint → Edit breakpoint → Set condition (e.g.,
i === 10
).
Example:
for(let i = 0; i < 100; i++) {
// Breakpoint triggers only when i equals 10
}
Final Thoughts
Mastering debugging techniques and tools greatly improves your productivity and coding efficiency. Which debugging techniques do you find most useful?
Share your best practices and experiences in the comments below!