How to Automate Print Design Workflows with Python and APIs
In the world of print design, efficiency is key. Whether you're creating business cards, brochures, or large-scale banners, streamlining your workflow can save time and reduce errors. For developers and designers alike, automating repetitive tasks can be a game-changer. In this article, we’ll explore how you can use Python and APIs to automate print design workflows, making your process faster and more efficient. Why Automate Print Design Workflows? Print design often involves repetitive tasks like resizing images, generating PDFs, or sending files to a printing service. Automating these tasks not only saves time but also ensures consistency across projects. By leveraging Python and APIs, you can create scripts that handle these tasks seamlessly. Getting Started with Python for Print Automation Python is a versatile programming language that’s perfect for automation. Here’s a simple example of how you can use Python to resize images for print: from PIL import Image def resize_image(input_path, output_path, size): with Image.open(input_path) as img: resized_img = img.resize(size) resized_img.save(output_path) print(f"Image saved to {output_path}") # Example usage resize_image("input.jpg", "output.jpg", (1200, 800)) This script uses the Python Imaging Library (PIL) to resize an image to the desired dimensions, which is a common task in print design. Integrating with Printing Services via APIs Many modern printing services, like Print in London, offer APIs that allow you to automate the process of uploading designs, checking print specifications, and placing orders. By integrating these APIs into your workflow, you can create a seamless pipeline from design to production. Here’s an example of how you might use an API to upload a design file: import requests def upload_design(api_url, file_path, api_key): headers = {"Authorization": f"Bearer {api_key}"} with open(file_path, "rb") as file: response = requests.post(api_url, files={"file": file}, headers=headers) if response.status_code == 200: print("Design uploaded successfully!") else: print(f"Error: {response.status_code} - {response.text}") # Example usage upload_design("https://api.printinlondon.co.uk/upload", "design.pdf", "your_api_key_here") This script sends a design file to a printing service’s API, allowing you to automate the upload process. Benefits of Automating Your Print Workflow Time Savings: Automating repetitive tasks frees up time for more creative work. Consistency: Automated workflows reduce the risk of human error. Scalability: Easily handle larger volumes of work without additional effort. Integration: Connect your design tools with printing services for a seamless process. Conclusion Automating print design workflows with Python and APIs can significantly enhance your productivity and ensure high-quality results. Whether you’re resizing images, generating print-ready files, or integrating with a printing service like Print in London, automation is a powerful tool for modern designers and developers. Start exploring Python and APIs today to take your print design workflow to the next level! By sharing this article on dev.to, you can engage with a community of developers and designers who are always looking for ways to optimize their workflows. Plus, it’s a great opportunity to introduce them to the services offered by Print in London. Happy coding!
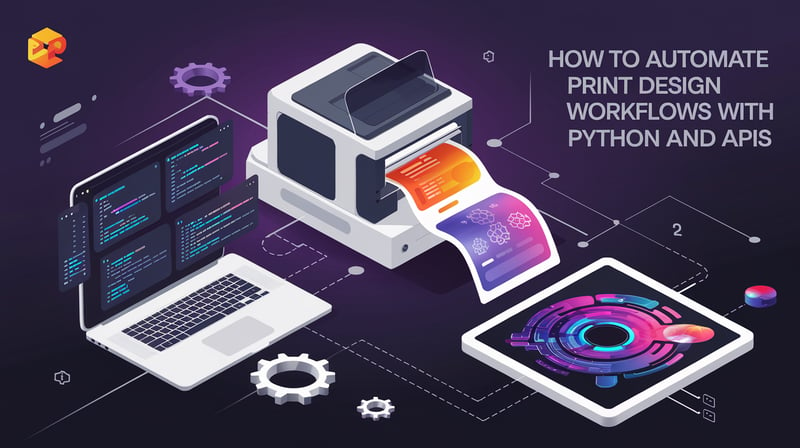
In the world of print design, efficiency is key. Whether you're creating business cards, brochures, or large-scale banners, streamlining your workflow can save time and reduce errors. For developers and designers alike, automating repetitive tasks can be a game-changer. In this article, we’ll explore how you can use Python and APIs to automate print design workflows, making your process faster and more efficient.
Why Automate Print Design Workflows?
Print design often involves repetitive tasks like resizing images, generating PDFs, or sending files to a printing service. Automating these tasks not only saves time but also ensures consistency across projects. By leveraging Python and APIs, you can create scripts that handle these tasks seamlessly.
Getting Started with Python for Print Automation
Python is a versatile programming language that’s perfect for automation. Here’s a simple example of how you can use Python to resize images for print:
from PIL import Image
def resize_image(input_path, output_path, size):
with Image.open(input_path) as img:
resized_img = img.resize(size)
resized_img.save(output_path)
print(f"Image saved to {output_path}")
# Example usage
resize_image("input.jpg", "output.jpg", (1200, 800))
This script uses the Python Imaging Library (PIL) to resize an image to the desired dimensions, which is a common task in print design.
Integrating with Printing Services via APIs
Many modern printing services, like Print in London, offer APIs that allow you to automate the process of uploading designs, checking print specifications, and placing orders. By integrating these APIs into your workflow, you can create a seamless pipeline from design to production.
Here’s an example of how you might use an API to upload a design file:
import requests
def upload_design(api_url, file_path, api_key):
headers = {"Authorization": f"Bearer {api_key}"}
with open(file_path, "rb") as file:
response = requests.post(api_url, files={"file": file}, headers=headers)
if response.status_code == 200:
print("Design uploaded successfully!")
else:
print(f"Error: {response.status_code} - {response.text}")
# Example usage
upload_design("https://api.printinlondon.co.uk/upload", "design.pdf", "your_api_key_here")
This script sends a design file to a printing service’s API, allowing you to automate the upload process.
Benefits of Automating Your Print Workflow
- Time Savings: Automating repetitive tasks frees up time for more creative work.
- Consistency: Automated workflows reduce the risk of human error.
- Scalability: Easily handle larger volumes of work without additional effort.
- Integration: Connect your design tools with printing services for a seamless process.
Conclusion
Automating print design workflows with Python and APIs can significantly enhance your productivity and ensure high-quality results. Whether you’re resizing images, generating print-ready files, or integrating with a printing service like Print in London, automation is a powerful tool for modern designers and developers.
Start exploring Python and APIs today to take your print design workflow to the next level!
By sharing this article on dev.to, you can engage with a community of developers and designers who are always looking for ways to optimize their workflows. Plus, it’s a great opportunity to introduce them to the services offered by Print in London. Happy coding!