Human-Readable IDs in PrismaJS with a few lines of code
TL;DR Use prisma-prefixed-ids to automatically generate prefixed, human-readable IDs (like usr_abc123) in your Prisma models. Inspired by Stripe’s article on designing APIs for humans, this drop-in extension improves clarity and structure with just one line of set up. No schema changes needed. Why Use Prefixed IDs? Ever debugged an API and thought “What even is this ID?” Stripe solves this by prefixing object IDs: cus_, pi_, sub_, etc. That little prefix gives context instantly. I wanted that in my Prisma projects—so I built prisma-prefixed-ids. Now all my Prisma models get smart, readable IDs like usr_xyz123. Install & Use Install it: npm install prisma-prefixed-ids Add one line to your Prisma setup: import { PrismaClient } from "@prisma/client"; import { extendPrismaClient } from "prisma-prefixed-ids"; const prisma = new PrismaClient(); const db = extendPrismaClient(prisma, { prefixes: { User: "usr", Organization: "org", }, }); Then use your extended client as usual: const user = await db.user.create({ data: { name: "Alice", email: "alice@example.com" } }); console.log(user.id); // "usr_xxxxxxxx" No need to manually set IDs—they’re generated automatically with the correct prefix. Why Use nanoid? Instead of UUIDs, this plugin uses nanoid for shorter, URL-safe, high-entropy IDs. ✅ ~128-bit randomness ✅ Shorter than UUID (24 vs. 36 chars) ✅ Customizable alphabet/length ✅ Great performance Bonus: Custom ID Generator Want hex IDs? Shorter strings? You can plug in your own generator: import { customAlphabet } from 'nanoid'; const generator = (prefix: string) => { const nanoid = customAlphabet("1234567890abcdef", 10); return `${prefix}_${nanoid()}`; }; extendPrismaClient(prisma, { prefixes: { User: "usr" }, idGenerator: generator, }); Deep Dive (For the Curious) Works only on models with String IDs. Uses Prisma’s native extension API (clean, no hacks). You can override the ID manually if needed. I use it in production on Verex.ai — it’s rock solid. Try It Out Give prisma-prefixed-ids a spin in your next project. It’s open source, easy to use, and makes your data more readable for devs, support, and even end users. Feedback and contributions are welcome on GitHub.
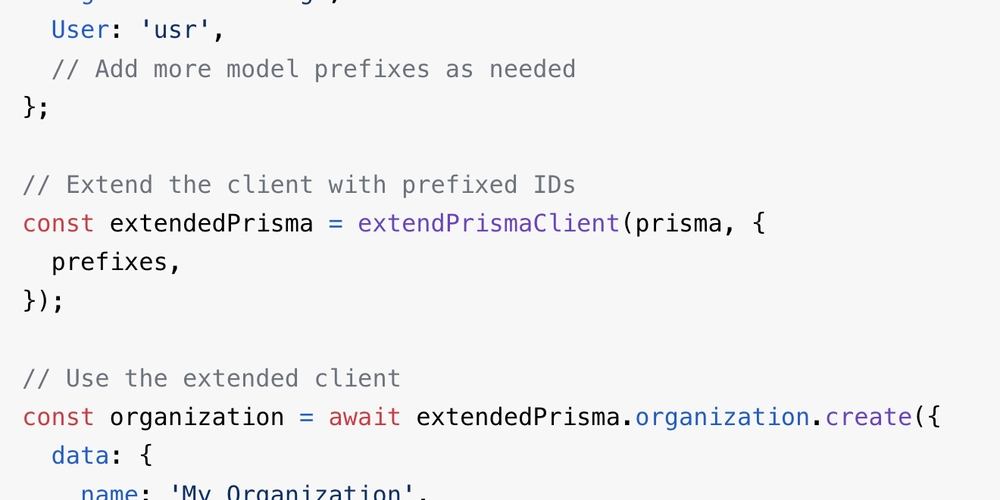
TL;DR
Use
prisma-prefixed-ids
to automatically generate prefixed, human-readable IDs (likeusr_abc123
) in your Prisma models. Inspired by Stripe’s article on designing APIs for humans, this drop-in extension improves clarity and structure with just one line of set up. No schema changes needed.
Why Use Prefixed IDs?
Ever debugged an API and thought “What even is this ID?”
Stripe solves this by prefixing object IDs: cus_
, pi_
, sub_
, etc. That little prefix gives context instantly. I wanted that in my Prisma projects—so I built prisma-prefixed-ids
. Now all my Prisma models get smart, readable IDs like usr_xyz123
.
Install & Use
Install it:
npm install prisma-prefixed-ids
Add one line to your Prisma setup:
import { PrismaClient } from "@prisma/client";
import { extendPrismaClient } from "prisma-prefixed-ids";
const prisma = new PrismaClient();
const db = extendPrismaClient(prisma, {
prefixes: {
User: "usr",
Organization: "org",
},
});
Then use your extended client as usual:
const user = await db.user.create({
data: { name: "Alice", email: "alice@example.com" }
});
console.log(user.id); // "usr_xxxxxxxx"
No need to manually set IDs—they’re generated automatically with the correct prefix.
Why Use nanoid
?
Instead of UUIDs, this plugin uses nanoid for shorter, URL-safe, high-entropy IDs.
✅ ~128-bit randomness
✅ Shorter than UUID (24 vs. 36 chars)
✅ Customizable alphabet/length
✅ Great performance
Bonus: Custom ID Generator
Want hex IDs? Shorter strings? You can plug in your own generator:
import { customAlphabet } from 'nanoid';
const generator = (prefix: string) => {
const nanoid = customAlphabet("1234567890abcdef", 10);
return `${prefix}_${nanoid()}`;
};
extendPrismaClient(prisma, {
prefixes: { User: "usr" },
idGenerator: generator,
});
Deep Dive (For the Curious)
- Works only on models with String IDs.
- Uses Prisma’s native extension API (clean, no hacks).
- You can override the ID manually if needed.
- I use it in production on Verex.ai — it’s rock solid.
Try It Out
Give prisma-prefixed-ids
a spin in your next project.
It’s open source, easy to use, and makes your data more readable for devs, support, and even end users.
Feedback and contributions are welcome on GitHub.