Signals in Python: How reaktiv brings Feline Reflexes to Your Backend Code
The Curious Cat in Your Codebase You know how cats seem to have an almost supernatural awareness of their surroundings? A leaf rustles outside, and the cat's ears immediately perk up. The refrigerator door opens from across the house, and suddenly your previously napping feline materializes at your feet. Cats embody the perfect reactive system – they observe, react instantly, and maintain minimal energy expenditure when nothing interesting is happening. What if your Python code could have these same feline reflexes? That's exactly what reaktiv brings to the table! A Brief History of Reactive Programming (With Cats, Naturally) Reactive programming has evolved much like our relationship with cats. In ancient Egypt, cats were revered as divine creatures. Similarly, early reactive programming concepts emerged in the 1970s with dataflow programming languages, but they were somewhat esoteric and specialized. Fast forward to the 2000s – just as cats conquered the internet with cute videos, reactive programming began its mainstream adoption. Libraries like Rx (Reactive Extensions) emerged, bringing observer patterns to a new level of sophistication. Then came the frontend revolution with frameworks like React (2013), Vue, and Angular adopting reactive paradigms. The concept of "Signals" (which reaktiv implements) gained particular traction with solid.js and Angular's signals implementation. These frameworks understood a fundamental truth: manually syncing data with UI is like herding cats – nearly impossible and frustratingly complex. Meet reaktiv: Your Python Backend's New Feline Superpower reaktiv brings the elegance of frontend reactivity to Python backends. It's like having a well-trained cat that knows exactly when to pounce on changes and when to conserve energy. # Install reaktiv now pip install reaktiv The core primitives are simple yet powerful: Signals - Store values and notify dependents when changed (like a cat's keen eyes spotting movement) Computed Signals - Derive values that update automatically (like a cat calculating the perfect jump trajectory) Effects - Run side effects when signals change (like a cat springing into action) Why reaktiv Solves Real Backend Headaches (Better Than Giving Catnip to Kittens) Backend systems face unique challenges that traditional programming approaches struggle with: 1. The Cascading Dependencies Problem Traditional approach: When user_profile changes, you manually update permissions, then access_controls, then ui_state... It's tedious, error-prone, and you'll inevitably forget something. With reaktiv: # Import from the reaktiv library from reaktiv import Signal, ComputeSignal user_profile = Signal({"name": "Whiskers", "role": "admin"}) permissions = ComputeSignal(lambda: calculate_permissions(user_profile.get())) access_controls = ComputeSignal(lambda: determine_access(permissions.get())) # Just update the source - everything else updates automatically! user_profile.set({"name": "Whiskers", "role": "user"}) 2. The Real-Time Data Flow Nightmare Handling real-time data in backends (like stock prices, IoT sensor readings, or live analytics) traditionally requires complex event buses, message queues, and subscription management. reaktiv simplifies this dramatically: # Import from reaktiv from reaktiv import Signal, ComputeSignal, Effect temperature = Signal(72.0) humidity = Signal(45.0) comfort_index = ComputeSignal(lambda: calculate_comfort(temperature.get(), humidity.get())) # Automatically tracks dependencies and updates only when needed alert_system = Effect(lambda: send_alert_if_needed(comfort_index.get())) alert_system.schedule() # When these update, only the affected computations will run temperature.set(78.5) # Triggers comfort_index update, which may trigger alert 3. The Async Coordination Dance Coordinating async operations in Python can be as chaotic as a roomful of kittens with laser pointers. reaktiv brings order with first-class async support: import asyncio from reaktiv import Signal, ComputeSignal, Effect async def monitor_system(): cpu_usage = Signal(0) memory_usage = Signal(0) system_status = ComputeSignal( lambda: "Critical" if cpu_usage.get() > 90 or memory_usage.get() > 85 else "Normal" ) # This effect runs asynchronously when dependencies change status_effect = Effect(lambda: log_status_change(system_status.get())) status_effect.schedule() # Updating signals automatically triggers dependent computations while True: cpu_usage.set(await get_cpu_usage()) memory_usage.set(await get_memory_usage()) await asyncio.sleep(5) Why reaktiv Outpaces Traditional Event Systems Ever tried to get multiple cats to move in a coordinated fashion? That's what traditional event/observer systems feel like in complex applications. Traditional Observer P
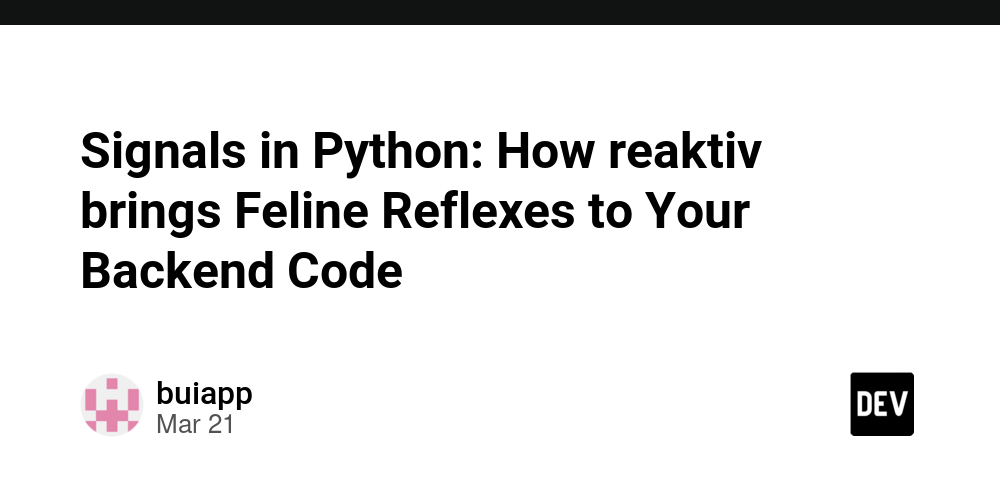
The Curious Cat in Your Codebase
You know how cats seem to have an almost supernatural awareness of their surroundings? A leaf rustles outside, and the cat's ears immediately perk up. The refrigerator door opens from across the house, and suddenly your previously napping feline materializes at your feet. Cats embody the perfect reactive system – they observe, react instantly, and maintain minimal energy expenditure when nothing interesting is happening.
What if your Python code could have these same feline reflexes? That's exactly what reaktiv
brings to the table!
A Brief History of Reactive Programming (With Cats, Naturally)
Reactive programming has evolved much like our relationship with cats. In ancient Egypt, cats were revered as divine creatures. Similarly, early reactive programming concepts emerged in the 1970s with dataflow programming languages, but they were somewhat esoteric and specialized.
Fast forward to the 2000s – just as cats conquered the internet with cute videos, reactive programming began its mainstream adoption. Libraries like Rx (Reactive Extensions) emerged, bringing observer patterns to a new level of sophistication.
Then came the frontend revolution with frameworks like React (2013), Vue, and Angular adopting reactive paradigms. The concept of "Signals" (which reaktiv
implements) gained particular traction with solid.js and Angular's signals implementation. These frameworks understood a fundamental truth: manually syncing data with UI is like herding cats – nearly impossible and frustratingly complex.
Meet reaktiv
: Your Python Backend's New Feline Superpower
reaktiv
brings the elegance of frontend reactivity to Python backends. It's like having a well-trained cat that knows exactly when to pounce on changes and when to conserve energy.
# Install reaktiv now
pip install reaktiv
The core primitives are simple yet powerful:
- Signals - Store values and notify dependents when changed (like a cat's keen eyes spotting movement)
- Computed Signals - Derive values that update automatically (like a cat calculating the perfect jump trajectory)
- Effects - Run side effects when signals change (like a cat springing into action)
Why reaktiv
Solves Real Backend Headaches (Better Than Giving Catnip to Kittens)
Backend systems face unique challenges that traditional programming approaches struggle with:
1. The Cascading Dependencies Problem
Traditional approach: When user_profile
changes, you manually update permissions
, then access_controls
, then ui_state
... It's tedious, error-prone, and you'll inevitably forget something.
With reaktiv
:
# Import from the reaktiv library
from reaktiv import Signal, ComputeSignal
user_profile = Signal({"name": "Whiskers", "role": "admin"})
permissions = ComputeSignal(lambda: calculate_permissions(user_profile.get()))
access_controls = ComputeSignal(lambda: determine_access(permissions.get()))
# Just update the source - everything else updates automatically!
user_profile.set({"name": "Whiskers", "role": "user"})
2. The Real-Time Data Flow Nightmare
Handling real-time data in backends (like stock prices, IoT sensor readings, or live analytics) traditionally requires complex event buses, message queues, and subscription management.
reaktiv
simplifies this dramatically:
# Import from reaktiv
from reaktiv import Signal, ComputeSignal, Effect
temperature = Signal(72.0)
humidity = Signal(45.0)
comfort_index = ComputeSignal(lambda: calculate_comfort(temperature.get(), humidity.get()))
# Automatically tracks dependencies and updates only when needed
alert_system = Effect(lambda: send_alert_if_needed(comfort_index.get()))
alert_system.schedule()
# When these update, only the affected computations will run
temperature.set(78.5) # Triggers comfort_index update, which may trigger alert
3. The Async Coordination Dance
Coordinating async operations in Python can be as chaotic as a roomful of kittens with laser pointers. reaktiv
brings order with first-class async support:
import asyncio
from reaktiv import Signal, ComputeSignal, Effect
async def monitor_system():
cpu_usage = Signal(0)
memory_usage = Signal(0)
system_status = ComputeSignal(
lambda: "Critical" if cpu_usage.get() > 90 or memory_usage.get() > 85 else "Normal"
)
# This effect runs asynchronously when dependencies change
status_effect = Effect(lambda: log_status_change(system_status.get()))
status_effect.schedule()
# Updating signals automatically triggers dependent computations
while True:
cpu_usage.set(await get_cpu_usage())
memory_usage.set(await get_memory_usage())
await asyncio.sleep(5)
Why reaktiv
Outpaces Traditional Event Systems
Ever tried to get multiple cats to move in a coordinated fashion? That's what traditional event/observer systems feel like in complex applications.
Traditional Observer Pattern |
reaktiv Signals |
---|---|
Manual subscription management | Automatic dependency tracking |
Easy to create memory leaks | Clean disposal system |
Execution order can be unpredictable | Predictable update propagation |
Difficult to debug when events misfire | Clear dependency graphs |
"Push" based (data pushed to observer) | "Pull" based (data pulled when needed) |
No built-in efficiency for unchanged values | Updates only when values actually change |
For the Frontend Developers: Yes, It's Those Signals!
If you've worked with Angular, solid.js, or other modern frontend frameworks, you'll feel right at home. reaktiv
brings the same mental model to Python:
# Import from reaktiv
from reaktiv import Signal, ComputeSignal, Effect
# This should look familiar to Angular developers
name = Signal("Mr. Whiskers")
greeting = ComputeSignal(lambda: f"Hello, {name.get()}!")
# Effects work just like in frontend frameworks
effect = Effect(lambda: print(greeting.get()))
effect.schedule()
# Change the input, see the output update automatically
name.set("Captain Meowmeow")
For the Backend Developers: This Isn't Your Grandfather's Observer Pattern
If you've never touched a frontend framework but have dealt with event-based systems, think of reaktiv
as Observer Pattern 2.0 with these key improvements:
-
Automatic tracking - No more manual
observer.subscribe(observable)
- Fine-grained updates - Only what changed gets updated, not everything
- Computation caching - Results are cached until dependencies change
-
Built for Python's async world - First-class support for
asyncio
Real-World Backend Example: The Cat Monitoring System
Let's build a cat-activity monitoring system using reaktiv
:
import asyncio
from reaktiv import Signal, ComputeSignal, Effect
async def cat_monitoring_system():
# Input signals
food_bowl_weight = Signal(100) # grams
water_level = Signal(200) # ml
activity_count = Signal(0) # movements per hour
# Computed signals
food_status = ComputeSignal(
lambda: "Low" if food_bowl_weight.get() < 20 else "OK"
)
water_status = ComputeSignal(
lambda: "Low" if water_level.get() < 50 else "OK"
)
cat_status = ComputeSignal(
lambda: "Lazy" if activity_count.get() < 5 else
"Normal" if activity_count.get() < 20 else "Hyperactive"
)
overall_status = ComputeSignal(
lambda: {
"food": food_status.get(),
"water": water_status.get(),
"activity": cat_status.get()
}
)
# Effect that runs only when relevant data changes
async def notify_owner():
status = overall_status.get()
if status["food"] == "Low" or status["water"] == "Low":
print(f"ALERT: Your cat needs attention! Status: {status}")
# Save reference to prevent garbage collection
notification = Effect(notify_owner)
notification.schedule()
# Simulate data changes
await asyncio.sleep(1)
food_bowl_weight.set(15) # This will trigger the notification
await asyncio.sleep(1)
activity_count.set(25) # This won't trigger notification (food is still low)
await asyncio.sleep(1)
food_bowl_weight.set(100) # Refilled food
water_level.set(30) # Water is now low - will trigger notification
await asyncio.sleep(1)
asyncio.run(cat_monitoring_system())
Try reaktiv
Today!
Like cats who instantly respond to the slightest movement, reaktiv
provides your Python code with that same level of effortless reactivity. It eliminates boilerplate, reduces bugs from forgotten updates, and makes dealing with changing state as natural as a cat's graceful movements.
Whether you're building real-time dashboards, complex data processing pipelines, or systems that respond to external events, reaktiv
offers a fresh approach that's both more elegant and more maintainable.
Get started with reaktiv
today:
-
Install it:
pip install reaktiv
- Star it on GitHub: https://github.com/buiapp/reaktiv
- Try the examples: Check out the examples folder in the repository
- Share your feedback: Open issues or submit PRs for improvements
Give your Python code the feline reflexes it deserves with reaktiv
– zero external dependencies, fully type-safe, and built for Python's async-first world!
P.S. Unlike real cats, reaktiv
will actually do what you tell it to, exactly when you need it to. No random 3 AM zoomies or keyboard walking, guaranteed!