If You Can Answer These 7 Questions Correctly You’re Decent at JavaScript
This article was originally published here JavaScript can be a little tricky sometimes, even when you’re dealing with simple-looking problems. Here are seven questions that test different parts of JavaScript. They look easy, but they can catch you off guard! If you can answer these, you’ve got a good handle on JavaScript! JOIN MY FREE WEEKLY WEBDEV NEWSLETTER! Question 1: What’s the Result of 0.1 + 0.2 === 0.3? console.log(0.1 + 0.2 === 0.3); Answer: The result is false. Explanation: In JavaScript, numbers with decimals (called floating-point numbers) don’t always add up the way we expect. Due to how floating-point numbers are represented in JavaScript, 0.1 + 0.2 doesn’t exactly equal 0.3. Instead, it results in 0.30000000000000004, leading to the comparison being false. This issue comes from the binary approximation of decimal numbers in JavaScript. Question 2: What’s the Result of "5" + 3 and "5" - 3? console.log("5" + 3); console.log("5" - 3); Answer: "5" + 3 results in "53". "5" - 3 results in 2. Explanation: "5" + 3: When you use the + sign with a string and a number, JavaScript treats the number like a part of the string. So instead of adding 5 and 3 as numbers, it sticks them together as text, resulting in "53". "5" - 3: The - operator doesn’t work with strings, so JavaScript converts "5" to a number and subtracts 3, resulting in 2. Question 3: What’s the Value of typeof null? console.log(typeof null); Answer: The result is "object". Explanation: This is a weird part of JavaScript. The typeof operator should tell you what kind of value you have. But when you check typeof null, JavaScript mistakenly says it’s an object, even though null is actually a special value that means "nothing." This is a bug that has been around for a long time and hasn’t been changed to keep old code from breaking. Question 4: How Does a Closure Work? function outerFunction() { let count = 0; return function() { count++; console.log(count); }; } const closure = outerFunction(); closure(); // ? closure(); // ? Answer: The output will be: 1 2 Explanation: A closure happens when a function remembers the variables around it, even after the outer function has finished running. In this example, the inner function still has access to the count variable inside outerFunction. Each time you call closure(), it increases count and shows it. Question 5: What’s the Result of true + false and [] + {}? console.log(true + false); console.log([] + {}); Answer: true + false gives 1. [] + {} gives "[object Object]". Explanation: true + false: In JavaScript, true is treated as 1 and false as 0. Adding 1 + 0 gives 1. [] + {}: When you add an empty array [] to an empty object {}, JavaScript changes them to strings. The empty array becomes an empty string "", and the empty object becomes "[object Object]". So, adding them gives "[object Object]". Question 6: What Does [] == ![] Mean? console.log([] == ![]); Answer: The result is true. Explanation: This is tricky! Here’s what happens: ![] means "not an empty array." An empty array is a "truthy" value, so ![] is false. Now, the expression is [] == false. JavaScript tries to compare [] and false. It changes [] into an empty string "" and false into 0. Then, "" == 0 is true because JavaScript changes the empty string to 0 when comparing. Question 7: What’s the Output of console.log(a) in the Following Code? console.log(a); var a = 5; Answer: The output is undefined. Explanation: This happens because of something called hoisting. When JavaScript reads your code, it moves all variable declarations to the top of their scope. So, in this case, JavaScript sees var a; at the top, but the value 5 hasn’t been assigned yet. So when you try to log a, it’s declared but still undefined. Conclusion How did you do? These questions mix JavaScript’s odd behavior with some important concepts like closures and hoisting. Knowing these will help you avoid common mistakes and better understand how JavaScript handles different operations. Happy coding!
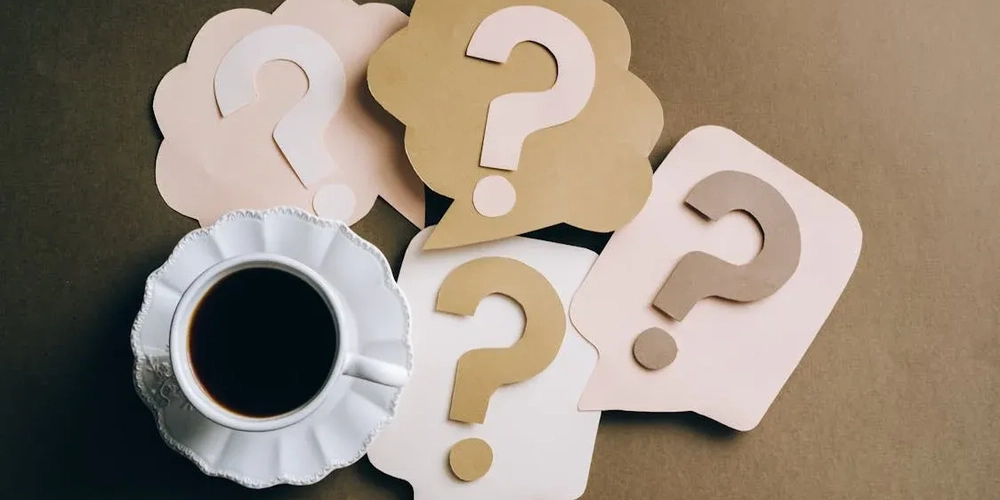
This article was originally published here
JavaScript can be a little tricky sometimes, even when you’re dealing with simple-looking problems.
Here are seven questions that test different parts of JavaScript.
They look easy, but they can catch you off guard! If you can answer these, you’ve got a good handle on JavaScript!
JOIN MY FREE WEEKLY WEBDEV NEWSLETTER!
Question 1: What’s the Result of 0.1 + 0.2 === 0.3?
console.log(0.1 + 0.2 === 0.3);
Answer:
The result is false.
Explanation:
In JavaScript, numbers with decimals (called floating-point numbers) don’t always add up the way we expect.
Due to how floating-point numbers are represented in JavaScript, 0.1 + 0.2 doesn’t exactly equal 0.3. Instead, it results in 0.30000000000000004, leading to the comparison being false. This issue comes from the binary approximation of decimal numbers in JavaScript.
Question 2: What’s the Result of "5" + 3 and "5" - 3?
console.log("5" + 3);
console.log("5" - 3);
Answer:
"5" + 3 results in "53".
"5" - 3 results in 2.
Explanation:
"5" + 3: When you use the + sign with a string and a number, JavaScript treats the number like a part of the string. So instead of adding 5 and 3 as numbers, it sticks them together as text, resulting in "53".
"5" - 3: The - operator doesn’t work with strings, so JavaScript converts "5" to a number and subtracts 3, resulting in 2.
Question 3: What’s the Value of typeof null?
console.log(typeof null);
Answer:
The result is "object".
Explanation:
This is a weird part of JavaScript. The typeof operator should tell you what kind of value you have.
But when you check typeof null, JavaScript mistakenly says it’s an object, even though null is actually a special value that means "nothing."
This is a bug that has been around for a long time and hasn’t been changed to keep old code from breaking.
Question 4: How Does a Closure Work?
function outerFunction() {
let count = 0;
return function() {
count++;
console.log(count);
};
}
const closure = outerFunction();
closure(); // ?
closure(); // ?
Answer:
The output will be:
1
2
Explanation:
A closure happens when a function remembers the variables around it, even after the outer function has finished running.
In this example, the inner function still has access to the count variable inside outerFunction. Each time you call closure(), it increases count and shows it.
Question 5: What’s the Result of true + false and [] + {}?
console.log(true + false);
console.log([] + {});
Answer:
true + false gives 1.
[] + {} gives "[object Object]".
Explanation:
true + false: In JavaScript, true is treated as 1 and false as 0. Adding 1 + 0 gives 1.
[] + {}: When you add an empty array [] to an empty object {}, JavaScript changes them to strings. The empty array becomes an empty string "", and the empty object becomes "[object Object]". So, adding them gives "[object Object]".
Question 6: What Does [] == ![] Mean?
console.log([] == ![]);
Answer:
The result is true.
Explanation:
This is tricky! Here’s what happens:
![] means "not an empty array." An empty array is a "truthy" value, so ![] is false.
Now, the expression is [] == false.
JavaScript tries to compare [] and false. It changes [] into an empty string "" and false into 0.
Then, "" == 0 is true because JavaScript changes the empty string to 0 when comparing.
Question 7: What’s the Output of console.log(a) in the Following Code?
console.log(a);
var a = 5;
Answer:
The output is undefined.
Explanation:
This happens because of something called hoisting. When JavaScript reads your code, it moves all variable declarations to the top of their scope.
So, in this case, JavaScript sees var a; at the top, but the value 5 hasn’t been assigned yet. So when you try to log a, it’s declared but still undefined.
Conclusion
How did you do?
These questions mix JavaScript’s odd behavior with some important concepts like closures and hoisting.
Knowing these will help you avoid common mistakes and better understand how JavaScript handles different operations.
Happy coding!