Integrating Supabase with Next.js: A Step-by-Step Guide
Integrating Supabase with Next.js: A Step-by-Step Guide Introduction In this tutorial, we will explore how to integrate Supabase with Next.js, covering essential features such as authentication, database setup, real-time subscriptions, and serverless functions. Supabase is an open-source Firebase alternative that provides a powerful backend for your applications, while Next.js is a popular React framework for building server-rendered applications. By the end of this guide, you will have a fully functional application that leverages the strengths of both technologies. Prerequisites Before we begin, ensure you have the following: Node.js installed on your machine A Supabase account (you can sign up for free) Basic knowledge of React and Next.js Step 1: Setting Up Your Next.js Project First, let's create a new Next.js project. Open your terminal and run: npx create-next-app supabase-nextjs-integration cd supabase-nextjs-integration Step 2: Installing Supabase Client Next, we need to install the Supabase client library. Run the following command: npm install @supabase/supabase-js Step 3: Configuring Supabase Go to your Supabase dashboard, create a new project, and note down your API URL and anon key. Now, create a .env.local file in your project root and add the following: NEXT_PUBLIC_SUPABASE_URL=your_supabase_url NEXT_PUBLIC_SUPABASE_ANON_KEY=your_anon_key Step 4: Setting Up Authentication To implement authentication, create a new file lib/supabase.js to initialize the Supabase client: // lib/supabase.js import { createClient } from '@supabase/supabase-js'; const supabaseUrl = process.env.NEXT_PUBLIC_SUPABASE_URL; const supabaseAnonKey = process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY; export const supabase = createClient(supabaseUrl, supabaseAnonKey); Next, create a simple authentication form in pages/index.js: // pages/index.js import { useState } from 'react'; import { supabase } from '../lib/supabase'; export default function Home() { const [email, setEmail] = useState(''); const handleLogin = async (e) => { e.preventDefault(); const { user, error } = await supabase.auth.signIn({ email }); if (error) console.error('Error logging in:', error); else console.log('User logged in:', user); }; return ( Login setEmail(e.target.value)} required /> Login ); } Step 5: Setting Up the Database In your Supabase dashboard, navigate to the SQL editor and create a new table: create table messages ( id serial primary key, content text, created_at timestamp with time zone default now() ); Step 6: Real-time Subscriptions To listen for real-time updates, we can use Supabase's subscription feature. Update your pages/index.js to include: import { useEffect } from 'react'; useEffect(() => { const subscription = supabase .from('messages') .on('INSERT', payload => { console.log('New message:', payload.new); }) .subscribe(); return () => { supabase.removeSubscription(subscription); }; }, []); Step 7: Implementing Serverless Functions Next.js allows you to create serverless functions easily. Create a new folder pages/api and add a file sendMessage.js: // pages/api/sendMessage.js import { supabase } from '../../lib/supabase'; export default async function handler(req, res) { if (req.method === 'POST') { const { content } = req.body; const { data, error } = await supabase .from('messages') .insert([{ content }]); if (error) return res.status(400).json({ error }); return res.status(200).json(data); } return res.status(405).json({ message: 'Method not allowed' }); } Conclusion In this tutorial, we successfully integrated Supabase with Next.js, covering authentication, database setup, real-time subscriptions, and serverless functions. This powerful combination allows you to build scalable applications with ease. Explore further by adding more features and enhancing your application! Tags nextjs supabase authentication real-time serverless Description Learn how to integrate Supabase with Next.js in this step-by-step tutorial, covering authentication, database setup, real-time subscriptions, and serverless functions.
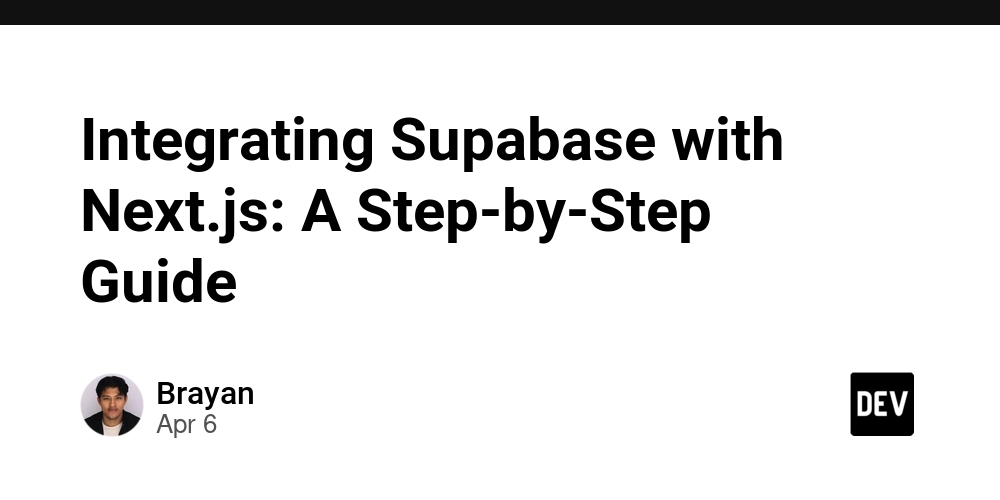
Integrating Supabase with Next.js: A Step-by-Step Guide
Introduction
In this tutorial, we will explore how to integrate Supabase with Next.js, covering essential features such as authentication, database setup, real-time subscriptions, and serverless functions. Supabase is an open-source Firebase alternative that provides a powerful backend for your applications, while Next.js is a popular React framework for building server-rendered applications. By the end of this guide, you will have a fully functional application that leverages the strengths of both technologies.
Prerequisites
Before we begin, ensure you have the following:
- Node.js installed on your machine
- A Supabase account (you can sign up for free)
- Basic knowledge of React and Next.js
Step 1: Setting Up Your Next.js Project
First, let's create a new Next.js project. Open your terminal and run:
npx create-next-app supabase-nextjs-integration
cd supabase-nextjs-integration
Step 2: Installing Supabase Client
Next, we need to install the Supabase client library. Run the following command:
npm install @supabase/supabase-js
Step 3: Configuring Supabase
Go to your Supabase dashboard, create a new project, and note down your API URL and anon key. Now, create a .env.local
file in your project root and add the following:
NEXT_PUBLIC_SUPABASE_URL=your_supabase_url
NEXT_PUBLIC_SUPABASE_ANON_KEY=your_anon_key
Step 4: Setting Up Authentication
To implement authentication, create a new file lib/supabase.js
to initialize the Supabase client:
// lib/supabase.js
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = process.env.NEXT_PUBLIC_SUPABASE_URL;
const supabaseAnonKey = process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl, supabaseAnonKey);
Next, create a simple authentication form in pages/index.js
:
// pages/index.js
import { useState } from 'react';
import { supabase } from '../lib/supabase';
export default function Home() {
const [email, setEmail] = useState('');
const handleLogin = async (e) => {
e.preventDefault();
const { user, error } = await supabase.auth.signIn({ email });
if (error) console.error('Error logging in:', error);
else console.log('User logged in:', user);
};
return (
<div>
<h1>Login</h1>
<form onSubmit={handleLogin}>
<input type="email" value={email} onChange={(e) => setEmail(e.target.value)} required />
<button type="submit">Login</button>
</form>
</div>
);
}
Step 5: Setting Up the Database
In your Supabase dashboard, navigate to the SQL editor and create a new table:
create table messages (
id serial primary key,
content text,
created_at timestamp with time zone default now()
);
Step 6: Real-time Subscriptions
To listen for real-time updates, we can use Supabase's subscription feature. Update your pages/index.js
to include:
import { useEffect } from 'react';
useEffect(() => {
const subscription = supabase
.from('messages')
.on('INSERT', payload => {
console.log('New message:', payload.new);
})
.subscribe();
return () => {
supabase.removeSubscription(subscription);
};
}, []);
Step 7: Implementing Serverless Functions
Next.js allows you to create serverless functions easily. Create a new folder pages/api
and add a file sendMessage.js
:
// pages/api/sendMessage.js
import { supabase } from '../../lib/supabase';
export default async function handler(req, res) {
if (req.method === 'POST') {
const { content } = req.body;
const { data, error } = await supabase
.from('messages')
.insert([{ content }]);
if (error) return res.status(400).json({ error });
return res.status(200).json(data);
}
return res.status(405).json({ message: 'Method not allowed' });
}
Conclusion
In this tutorial, we successfully integrated Supabase with Next.js, covering authentication, database setup, real-time subscriptions, and serverless functions. This powerful combination allows you to build scalable applications with ease. Explore further by adding more features and enhancing your application!
Tags
- nextjs
- supabase
- authentication
- real-time
- serverless
Description
Learn how to integrate Supabase with Next.js in this step-by-step tutorial, covering authentication, database setup, real-time subscriptions, and serverless functions.