Lifetimes in Rust: Preventing Dangling References
Introduction In a rust program the main aim of lifetimes is to prevent dangling references, which can cause a program to reference data other than the data it intends to reference. Dangling reference is a situation where a reference points to invalid memory. Tho Rust enforces strict borrowing rules, ensuring references are always vaid. However, there are instances where Rust can't infer reference lifetimes, and that's where lifetimes come in. This article is going to explain: ✅ What lifetimes are and why they matter ✅ How dangling references occur and how Rust prevents them. ✅ How to use lifetime annotations correctly. ✅ Common pitfalls and best practices. By the end of reading this article, you should understand what lifetimes are and how to use them in Rust. The Problem: Dangling References Using languages like C/C++, it's easy to dereference a pointer to an invalid memory location, which leads to segmentation faults or undefined behaviour. Example of a dangling reference in C++ #include int* getPointer() { int x = 10; // x is local to this function return &x; // Returning a reference to a local variable } int main() { int* ptr = getPointer(); // ptr now points to a freed memory location std::cout (x: &'a i32) { } struct Example &str { if x.len() > y.len() { x } else { y } } Now looking at this function, ordinarily, the function is supposed to compile right? Well, the borrow checker says a big NO!. When we compile this code, we get this error below: error[E0106]: missing lifetime specifier --> example.rs:1:33 | 1 | fn longest(x: &str, y: &str) -> &str { | ---- ---- ^ expected named lifetime parameter | = help: this function's return type contains a borrowed value, but the signature does not say whether it is borrowed from `x` or `y` help: consider introducing a named lifetime parameter | 1 | fn longest y.len() { x } else { y } } Now we introduce 'a which is a generic lifetime parameter, and have x and y parameters tied to this same lifetime. Finally we added the generic lifetime parameter to the return value, as such the returned reference is valid for at least as long as both inputs remain valid. Rust now ensures that what is being returned won't outlive x or y, prevent dangling references. Lifetimes in Structs In the case of a struct, if it holds reference(s), it must specify lifetimes to ensure that it doesn't outlive the referenced data. Let us look at this example below: struct Person &str { /* ... */ } The 'static Lifetime 'static is a special lifetime that means the reference lives for the entire program duration. Although it is advisable not to make use of the 'static lifetime except where is it absolutely necessary. By default, string literals have 'static lifetimes. let s: &`static str = "Hello, Bene!"; Summary Lifetimes in Rust ensures references remain valid, preventing memory safety issues at compile time. While most lifetimes are inferred, explicit annotations help resolve ambiguity in functions and structs.
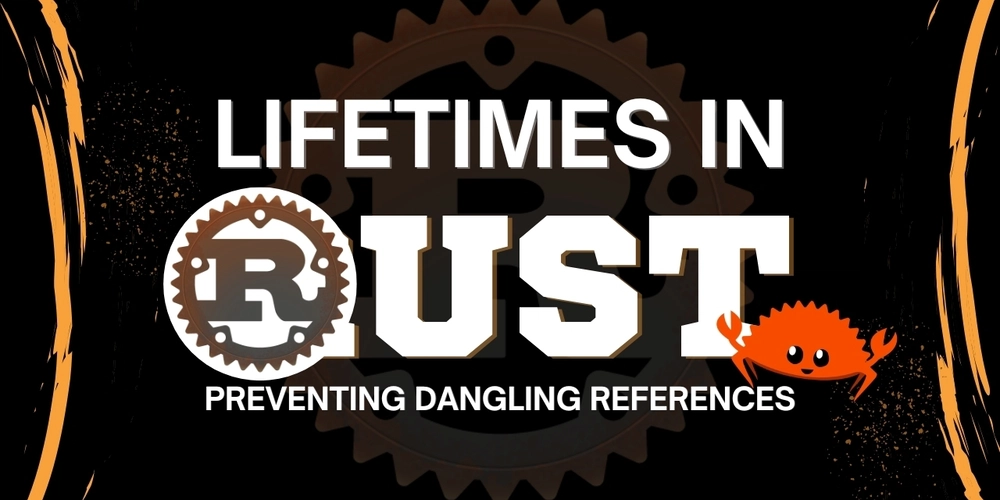
Introduction
In a rust program the main aim of lifetimes is to prevent dangling references, which can cause a program to reference data other than the data it intends to reference.
Dangling reference is a situation where a reference points to invalid memory. Tho Rust enforces strict borrowing rules, ensuring references are always vaid.
However, there are instances where Rust can't infer reference lifetimes, and that's where lifetimes come in.
This article is going to explain:
✅ What lifetimes are and why they matter
✅ How dangling references occur and how Rust prevents them.
✅ How to use lifetime annotations correctly.
✅ Common pitfalls and best practices.
By the end of reading this article, you should understand what lifetimes are and how to use them in Rust.
The Problem: Dangling References
Using languages like C/C++, it's easy to dereference a pointer to an invalid memory location, which leads to segmentation faults or undefined behaviour.
Example of a dangling reference in C++
#include
int* getPointer() {
int x = 10; // x is local to this function
return &x; // Returning a reference to a local variable
}
int main() {
int* ptr = getPointer(); // ptr now points to a freed memory location
std::cout << *ptr; // Undefined behavior!
}
The code above shows how ptr
points to memory that is no longer valid. It is a classic example of a dangling reference.
Rust's Prevention Mechanism
Rust using one of the components of it's compiler called the borrow checker strictly prevents such. For example:
fn main() {
let r;
{
let x = 10;
r = &x; // ❌ Error: `x` does not live long enough
}
println!("{}", r); // ❌ Borrow checker prevents use-after-free
}
Looking at the rust code above, we can say the code failed because:
-
x
is declared inside of the inner scope{}
. -
r
is assigned to a reference tox
, butx
goes out of scope after the block ends. -
r
now points to invalid memory. Rust stops this at compile time!
This is where lifetimes comes into play. So let us see what these lifetimes are...
What are Lifetimes in Rust?
Lifetimes are a way to tell Rust how long reference(s) should be valid. They don't change how long a value actually lives - they just help the compiler how long a reference should remain valid.
Some key facts about lifetimes:
✅ Every reference in Rust has a lifetime.
✅ The borrow checker compares lifetimes to ensure valid references.
✅ Most lifetimes are inferred (just like types), so you don't always need to specify them.
✅ Explicit lifetimes are needed when Rust can't infer them unambiguously (e.g., in function signatures or structs.)
Understanding Lifetime Annotations
The lifetime annotation syntax starts with an apostrophe '
. followed immediately with/by a letter or word (depends on what you would want to make use of), but conventionally a
or b
is being used. Lastly, for functions/structs the apostrophe '
, character a
or b
is enclosed in an angle bracket. For example:
fn example<'a>(x: &'a i32) { }
struct Example<'a> { part: &'a str }
Function Signatures and Lifetimes
Let us look at a situation where we have a function that find the longer of two string slices:
fn longest(x: &str, y: &str) -> &str {
if x.len() > y.len() {
x
} else {
y
}
}
Now looking at this function, ordinarily, the function is supposed to compile right? Well, the borrow checker says a big NO!.
When we compile this code, we get this error below:
error[E0106]: missing lifetime specifier
--> example.rs:1:33
|
1 | fn longest(x: &str, y: &str) -> &str {
| ---- ---- ^ expected named lifetime parameter
|
= help: this function's return type contains a borrowed value, but the signature does not say whether it is borrowed from `x` or `y`
help: consider introducing a named lifetime parameter
|
1 | fn longest<'a>(x: &'a str, y: &'a str) -> &'a str {
| ++++ ++ ++ ++
We get the error[E0106]: missing lifetime specifier
error. This error is trying to say the borrow check doesn't know if the returned reference will be coming from x
or y
since based on what the function is doing, it could go two ways, simply put as it can't determine its lifetime.
In fixing this error, we will have to include the lifetime parameter to our function:
fn longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
Now we introduce 'a
which is a generic lifetime parameter, and have x
and y
parameters tied to this same lifetime.
Finally we added the generic lifetime parameter to the return value, as such the returned reference is valid for at least as long as both inputs remain valid. Rust now ensures that what is being returned won't outlive x
or y
, prevent dangling references.
Lifetimes in Structs
In the case of a struct, if it holds reference(s), it must specify lifetimes to ensure that it doesn't outlive the referenced data. Let us look at this example below:
struct Person<'a> {
name: &'a str,
}
fn main() {
let name = String::from("Alice");
let person = Person { name: &name };
println!("{}", person.name); // ✅ Works fine
}
In this struct, 'a
is needed because without it, Rust wouldn't know how long Person
should be valid. The borrow checker ensures that the Person
struct never outlives name
.
Lifetime Elision (When You Can Skip It)
There are cases where Rust automatically infers lifetimes: this is what is referred as lifetime elision.
For example for a function with only one parameter reference, s
so the borrow checker assumes that the '/ reference lives as long as it.
This follows the Rust's lifetime elision rules.
fn first_word(s: &str) -> &str { /* ... */ }
The 'static
Lifetime
'static
is a special lifetime that means the reference lives for the entire program duration. Although it is advisable not to make use of the 'static
lifetime except where is it absolutely necessary. By default, string literals have 'static
lifetimes.
let s: &`static str = "Hello, Bene!";
Summary
Lifetimes in Rust ensures references remain valid, preventing memory safety issues at compile time. While most lifetimes are inferred, explicit annotations help resolve ambiguity in functions and structs.