Mastering lxml in Python: Parse XML and HTML Like a Pro
Introduction XML and HTML are everywhere—from APIs to scraped websites. In this post, I’ll show you how to use lxml, a powerful and fast Python library for parsing and manipulating XML/HTML. Installation pip install lxml Parsing XML from lxml import etree xml_data = '''OneTwo''' root = etree.fromstring(xml_data) for item in root.findall('item'): print(item.text) Parsing HTML from lxml import html html_content = 'Hello' tree = html.fromstring(html_content) heading = tree.xpath('//h1/text()') print(heading[0]) # Output: Hello XPath Basics Explain how XPath is used to select nodes: links = tree.xpath('//a/@href') Error Handling & Best Practices Use try/except Validate structure before parsing Real-world Use Cases Scraping Working with config files Parsing API responses Conclusion lxml gives you superpowers for XML and HTML parsing. Whether you're a beginner or advanced dev, it’s worth mastering!
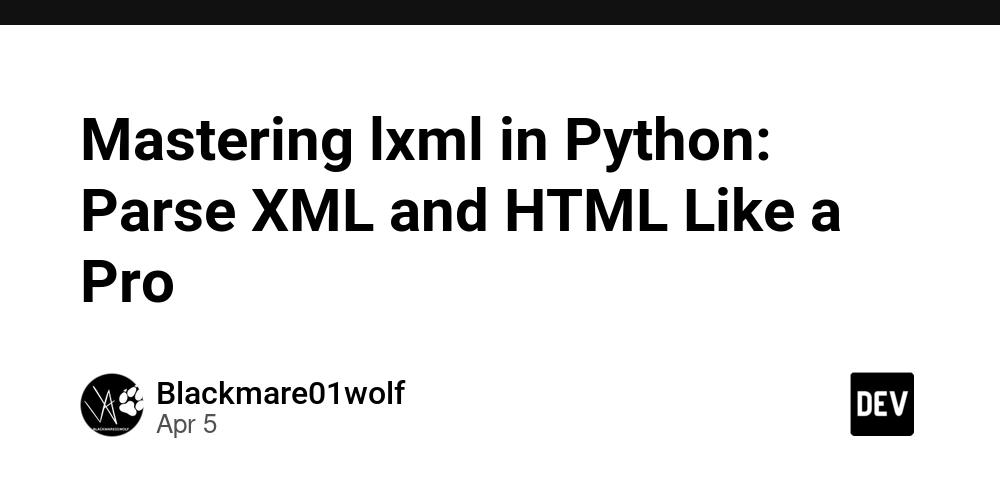
Introduction
XML and HTML are everywhere—from APIs to scraped websites. In this post, I’ll show you how to use lxml, a powerful and fast Python library for parsing and manipulating XML/HTML.
Installation
pip install lxml
Parsing XML
from lxml import etree
xml_data = '''- One
- Two
'''
root = etree.fromstring(xml_data)
for item in root.findall('item'):
print(item.text)
Parsing HTML
from lxml import html
html_content = 'Hello
'
tree = html.fromstring(html_content)
heading = tree.xpath('//h1/text()')
print(heading[0]) # Output: Hello
XPath Basics
Explain how XPath is used to select nodes:
links = tree.xpath('//a/@href')
Error Handling & Best Practices
- Use try/except
- Validate structure before parsing
Real-world Use Cases
- Scraping
- Working with config files
- Parsing API responses
Conclusion
lxml gives you superpowers for XML and HTML parsing. Whether you're a beginner or advanced dev, it’s worth mastering!