Terraform Modules: Organize Your Infra as Code in Scalable Way
If you're just getting started with Terraform, you’ve probably created all your .tf files in the same folder, mixing all your resources together. But as your project grows, this setup can get hard to manage. That’s where Terraform Modules come in — a powerful way to organize, reuse, and standardize your infrastructure. What Is a Terraform Module ? A module is simply a folder with terraform files. You can reuse it in different parts of your project - or even in other projects. Any folder with .tf files can be considered a module. Basic Structure of a Module Let's say you want to create a VPC on AWS. You can create a module called vpc with this structure: modules/ └── vpc/ ├── main.tf ├── variables.tf ├── outputs.tf main.tf resource "aws_vpc" "main" { cidr_block = var.cidr_block tags = { Name = var.name } } variables.tf variable "cidr_block" { type = string } variable "name" { type = string } outputs.tf output "vpc_id" { value = aws_vpc.main.id } How to Use a Module Now that your module is ready, you can call it in your main Terraform file like this. module "vpc" { source = "./modules/vpc" cidr_block = "10.0.0.0/16" name = "my-vpc" } Why Use Modules ? Reusability: Write code once and use it many times. Organization: Easier to read and maintain. Standardization: Helps teams follow the same patterns. Using Community Modules Terraform has a Terraform Registry with lots of ready-to-use modules. Here's an example using an official AWS VPC module: module "vpc" { source = "terraform-aws-modules/vpc/aws" version = "5.1.0" name = "my-vpc" cidr = "10.0.0.0/16" azs = ["us-east-1a", "us-east-1b"] public_subnets = ["10.0.1.0/24", "10.0.2.0/24"] } Best Practices Use clear and short names for your modules. Always document your variables and outputs. Use versioning (especially for remote modules). Keep your modules simple. Avoid too much logic inside them. Conclusion Terraform modules are a key part of building clean, scalable, and maintainable infrastructure as code. Whether you're working solo or in a team, modules help you avoid repetition, improve readability, and speed up your development process. Start small: move a few resources into a module, test it, and refactor as needed. With time, you'll build a library of reusable modules that will save you hours on every new project. The more your infrastructure grows, the more you'll thank yourself for using modules from the beginning.
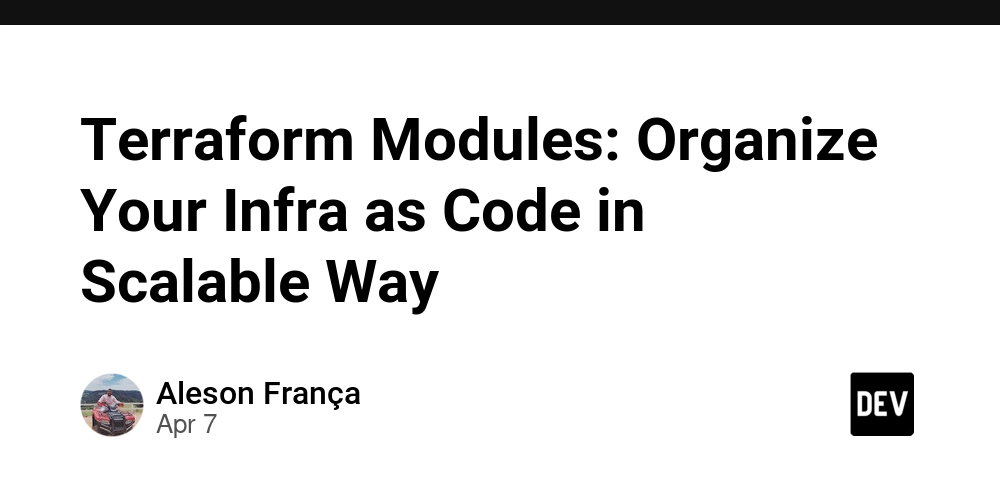
If you're just getting started with Terraform, you’ve probably created all your .tf files in the same folder, mixing all your resources together. But as your project grows, this setup can get hard to manage.
That’s where Terraform Modules come in — a powerful way to organize, reuse, and standardize your infrastructure.
What Is a Terraform Module ?
A module is simply a folder with terraform files. You can reuse it in different parts of your project - or even in other projects.
Any folder with .tf
files can be considered a module.
Basic Structure of a Module
Let's say you want to create a VPC on AWS. You can create a module called vpc
with this structure:
modules/
└── vpc/
├── main.tf
├── variables.tf
├── outputs.tf
main.tf
resource "aws_vpc" "main" {
cidr_block = var.cidr_block
tags = {
Name = var.name
}
}
variables.tf
variable "cidr_block" {
type = string
}
variable "name" {
type = string
}
outputs.tf
output "vpc_id" {
value = aws_vpc.main.id
}
How to Use a Module
Now that your module is ready, you can call it in your main Terraform file like this.
module "vpc" {
source = "./modules/vpc"
cidr_block = "10.0.0.0/16"
name = "my-vpc"
}
Why Use Modules ?
Reusability: Write code once and use it many times.
Organization: Easier to read and maintain.
Standardization: Helps teams follow the same patterns.
Using Community Modules
Terraform has a Terraform Registry with lots of ready-to-use modules. Here's an example using an official AWS VPC module:
module "vpc" {
source = "terraform-aws-modules/vpc/aws"
version = "5.1.0"
name = "my-vpc"
cidr = "10.0.0.0/16"
azs = ["us-east-1a", "us-east-1b"]
public_subnets = ["10.0.1.0/24", "10.0.2.0/24"]
}
Best Practices
Use clear and short names for your modules.
Always document your variables and outputs.
Use versioning (especially for remote modules).
Keep your modules simple. Avoid too much logic inside them.
Conclusion
Terraform modules are a key part of building clean, scalable, and maintainable infrastructure as code. Whether you're working solo or in a team, modules help you avoid repetition, improve readability, and speed up your development process.
Start small: move a few resources into a module, test it, and refactor as needed. With time, you'll build a library of reusable modules that will save you hours on every new project.
The more your infrastructure grows, the more you'll thank yourself for using modules from the beginning.