Traits in Rust Explained: From Usage to Internal Mechanics
In Rust’s design goals, zero-cost abstractions are one of the most important principles. They allow Rust to have the expressive power of a high-level language without sacrificing performance. The foundation of these zero-cost abstractions lies in generics and traits, which allow high-level syntax to be compiled into efficient low-level code during compilation, achieving runtime efficiency. This article introduces traits, including how to use them and analyzes three common problems, explaining their underlying mechanisms through the exploration of these issues. Usage Basic Usage The main purpose of traits is to abstract behaviors, similar to "interfaces" in other programming languages. Here's an example to illustrate the basic usage of traits: trait Greeting { fn greeting(&self) -> &str; } struct Cat; impl Greeting for Cat { fn greeting(&self) -> &str { "Meow!" } } struct Dog; impl Greeting for Dog { fn greeting(&self) -> &str { "Woof!" } } In the code above, a trait Greeting is defined and implemented by two structs. Depending on how the function is called, there are two primary ways to use it: Static dispatch based on generics Dynamic dispatch based on trait objects The concept of generics is more commonly known, so we’ll focus here on trait objects: A trait object is an opaque value of another type that implements a set of traits. The set of traits is made up of an object safe base trait plus any number of auto traits. An important detail is that trait objects belong to Dynamically Sized Types (DST), meaning their size cannot be determined at compile time. They must be accessed indirectly through pointers. Common forms include Box, &dyn Trait, etc. fn print_greeting_static(g: G) { println!("{}", g.greeting()); } fn print_greeting_dynamic(g: Box) { println!("{}", g.greeting()); } print_greeting_static(Cat); print_greeting_static(Dog); print_greeting_dynamic(Box::new(Cat)); print_greeting_dynamic(Box::new(Dog)); Static Dispatch In Rust, generics are implemented using monomorphization, which generates different versions of a function at compile time for different types. Therefore, generics are also known as type parameters. The advantage is that there's no overhead from virtual function calls, but the downside is increased binary size. In the example above, print_greeting_static would be compiled into two versions: print_greeting_static_cat(Cat); print_greeting_static_dog(Dog); Dynamic Dispatch Not all function calls can have their caller type determined at compile time. A common scenario is callbacks for events in GUI programming. Typically, one event may correspond to multiple callback functions, which are not known at compile time. Therefore, generics are not suitable in such cases, and dynamic dispatch is needed: trait ClickCallback { fn on_click(&self, x: i64, y: i64); } struct Button { listeners: Vec, } impl Trait In Rust 1.26, a new way of using traits was introduced: impl Trait, which can be used in two places—function parameters and return values. This is mainly to simplify the use of complex traits and can be regarded as a special case of generics. When using impl Trait, it's still static dispatch. However, when used as a return type, the data type must be the same across all return paths—this is a critical point! fn print_greeting_impl(g: impl Greeting) { println!("{}", g.greeting()); } print_greeting_impl(Cat); print_greeting_impl(Dog); // The following code will result in a compilation error fn return_greeting_impl(i: i32) -> impl Greeting { if i > 10 { return Cat; } Dog } // | fn return_greeting_impl(i: i32) -> impl Greeting { // | ------------- expected because this return type... // | if i > 10 { // | return Cat; // | --- ...is found to be `Cat` here // | } // | Dog // | ^^^ expected struct `Cat`, found struct `Dog` Advanced Usage Associated Types In the basic usage section above, the parameter or return types in trait methods are fixed. Rust provides a mechanism called lazy binding of types, namely associated types, which allows the concrete type to be specified when implementing the trait. A common example is the standard library’s Iterator trait, where the return value of next is Self::Item: trait Iterator { type Item; fn next(&mut self) -> Option; } /// A sample iterator that outputs only even numbers struct EvenNumbers { count: usize, limit: usize, } impl Iterator for EvenNumbers { type Item = usize; fn next(&mut self) -> Option { if self.count > self.limit { return None; } let ret = self.count * 2; self.count += 1; Some(ret) } } fn main() { let nums = EvenNumbers { count: 1, limit: 5 }; for n in nums { printl
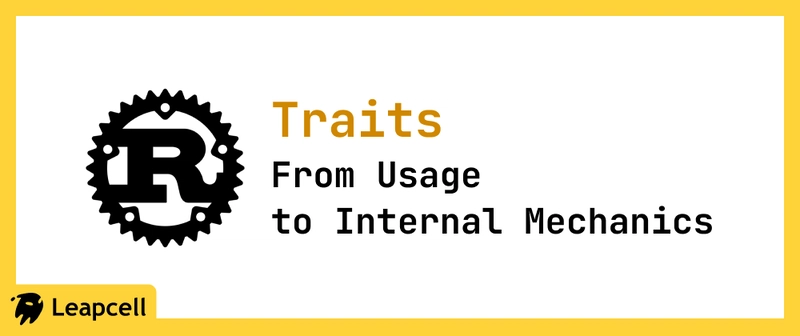
In Rust’s design goals, zero-cost abstractions are one of the most important principles. They allow Rust to have the expressive power of a high-level language without sacrificing performance. The foundation of these zero-cost abstractions lies in generics and traits, which allow high-level syntax to be compiled into efficient low-level code during compilation, achieving runtime efficiency. This article introduces traits, including how to use them and analyzes three common problems, explaining their underlying mechanisms through the exploration of these issues.
Usage
Basic Usage
The main purpose of traits is to abstract behaviors, similar to "interfaces" in other programming languages. Here's an example to illustrate the basic usage of traits:
trait Greeting {
fn greeting(&self) -> &str;
}
struct Cat;
impl Greeting for Cat {
fn greeting(&self) -> &str {
"Meow!"
}
}
struct Dog;
impl Greeting for Dog {
fn greeting(&self) -> &str {
"Woof!"
}
}
In the code above, a trait Greeting
is defined and implemented by two structs. Depending on how the function is called, there are two primary ways to use it:
- Static dispatch based on generics
- Dynamic dispatch based on trait objects
The concept of generics is more commonly known, so we’ll focus here on trait objects:
A trait object is an opaque value of another type that implements a set of traits. The set of traits is made up of an object safe base trait plus any number of auto traits.
An important detail is that trait objects belong to Dynamically Sized Types (DST), meaning their size cannot be determined at compile time. They must be accessed indirectly through pointers. Common forms include Box
, &dyn Trait
, etc.
fn print_greeting_static<G: Greeting>(g: G) {
println!("{}", g.greeting());
}
fn print_greeting_dynamic(g: Box<dyn Greeting>) {
println!("{}", g.greeting());
}
print_greeting_static(Cat);
print_greeting_static(Dog);
print_greeting_dynamic(Box::new(Cat));
print_greeting_dynamic(Box::new(Dog));
Static Dispatch
In Rust, generics are implemented using monomorphization, which generates different versions of a function at compile time for different types. Therefore, generics are also known as type parameters. The advantage is that there's no overhead from virtual function calls, but the downside is increased binary size. In the example above, print_greeting_static
would be compiled into two versions:
print_greeting_static_cat(Cat);
print_greeting_static_dog(Dog);
Dynamic Dispatch
Not all function calls can have their caller type determined at compile time. A common scenario is callbacks for events in GUI programming. Typically, one event may correspond to multiple callback functions, which are not known at compile time. Therefore, generics are not suitable in such cases, and dynamic dispatch is needed:
trait ClickCallback {
fn on_click(&self, x: i64, y: i64);
}
struct Button {
listeners: Vec<Box<dyn ClickCallback>>,
}
impl Trait
In Rust 1.26, a new way of using traits was introduced: impl Trait
, which can be used in two places—function parameters and return values. This is mainly to simplify the use of complex traits and can be regarded as a special case of generics. When using impl Trait
, it's still static dispatch. However, when used as a return type, the data type must be the same across all return paths—this is a critical point!
fn print_greeting_impl(g: impl Greeting) {
println!("{}", g.greeting());
}
print_greeting_impl(Cat);
print_greeting_impl(Dog);
// The following code will result in a compilation error
fn return_greeting_impl(i: i32) -> impl Greeting {
if i > 10 {
return Cat;
}
Dog
}
// | fn return_greeting_impl(i: i32) -> impl Greeting {
// | ------------- expected because this return type...
// | if i > 10 {
// | return Cat;
// | --- ...is found to be `Cat` here
// | }
// | Dog
// | ^^^ expected struct `Cat`, found struct `Dog`
Advanced Usage
Associated Types
In the basic usage section above, the parameter or return types in trait methods are fixed. Rust provides a mechanism called lazy binding of types, namely associated types, which allows the concrete type to be specified when implementing the trait. A common example is the standard library’s Iterator
trait, where the return value of next
is Self::Item
:
trait Iterator {
type Item;
fn next(&mut self) -> Option<Self::Item>;
}
/// A sample iterator that outputs only even numbers
struct EvenNumbers {
count: usize,
limit: usize,
}
impl Iterator for EvenNumbers {
type Item = usize;
fn next(&mut self) -> Option<Self::Item> {
if self.count > self.limit {
return None;
}
let ret = self.count * 2;
self.count += 1;
Some(ret)
}
}
fn main() {
let nums = EvenNumbers { count: 1, limit: 5 };
for n in nums {
println!("{}", n);
}
}
// Outputs: 2 4 6 8 10
The use of associated types is similar to generics. The Iterator
trait can also be defined using generics:
pub trait Iterator<T> {
fn next(&mut self) -> Option<T>;
}
The main differences between the two approaches are:
- A specific type (such as the
Cat
struct above) can implement a generic trait multiple times. For example, with theFrom
trait, you can have bothimpl From<&str> for Cat
andimpl From
.for Cat - However, a trait with an associated type can only be implemented once. For instance, with
FromStr
, you can only have oneimpl FromStr for Cat
. Traits likeIterator
andDeref
follow this pattern.
Derive Macros
In Rust, the derive
attribute can be used to automatically implement some common traits, such as Debug
or Clone
. For user-defined traits, it is also possible to implement procedural macros to support derive
. For more details, see: How to write a custom derive macro?. We won't go into further detail here.
Common Problems
Upcasting
For traits where SubTrait: Base
, in the current version of Rust, it is not possible to convert a &dyn SubTrait
into a &dyn Base
. This limitation is related to the memory layout of trait objects.
In the article Exploring Rust fat pointers, the author used transmute
to convert a trait object reference into two usize
values and verified that they point to the data and the vtable, respectively:
use std::mem::transmute;
use std::fmt::Debug;
fn main() {
let v = vec![1, 2, 3, 4];
let a: &Vec<u64> = &v;
// Convert to trait object
let b: &dyn Debug = &v;
println!("a: {}", a as *const _ as usize);
println!("b: {:?}", unsafe { transmute::<_, (usize, usize)>(b) });
}
// a: 140735227204568
// b: (140735227204568, 94484672107880)
This demonstrates that Rust uses fat pointers (i.e., two pointers) to represent trait object references: one pointing to the data, and the other to the vtable. This is very similar to how interfaces are handled in Go.
+---------------------+
| fat object pointer |
+---------+-----------+
| data | vtable |
+----|----+----|------+
| |
v v
+---------+ +-----------+
| object | | vtable |
+---------+ +-----+-----+
| ... | | S | S |
+---------+ +-----+-----+
pub struct TraitObjectReference {
pub data: *mut (),
pub vtable: *mut (),
}
struct Vtable {
destructor: fn(*mut ()),
size: usize,
align: usize,
method: fn(*const ()) -> String,
}
Although fat pointers increase the size of pointers (which makes them unusable with atomic operations), the benefits are significant:
- Traits can be implemented for existing types (e.g., blanket implementations)
- Calling a method from the vtable only requires one level of indirection. In contrast, in C++, the vtable resides inside the object, so each function call involves two levels of indirection, like this:
object pointer --> object contents --> vtable --> DynamicType::method() implementation
When a trait has an inheritance relationship, how does the vtable store methods from multiple traits? In the current implementation, all methods are stored sequentially in a single vtable, like this:
Trait Object
+---------------+ +------------------+
| data | <------------ | data |
+---------------+ +------------------+
| vtable | ------------> +---------------------+
+------------------+ | destructor |
+---------------------+
| size |
+---------------------+
| align |
+---------------------+
| base.fn1 |
+---------------------+
| base.fn2 |
+---------------------+
| subtrait.fn1 |
+---------------------+
| ...... |
+---------------------+
As you can see, all trait methods are stored in sequence without any distinction between which method belongs to which trait. This is why upcasting is not possible. There's an ongoing RFC—RFC 2765—tracking this issue. Instead of discussing the solution proposed by the RFC here, we’ll introduce a more general workaround by adding an AsBase
trait:
trait Base {
fn base(&self) {
println!("base...");
}
}
trait AsBase {
fn as_base(&self) -> &dyn Base;
}
// Blanket implementation
impl<T: Base> AsBase for T {
fn as_base(&self) -> &dyn Base {
self
}
}
trait Foo: AsBase {
fn foo(&self) {
println!("foo..");
}
}
#[derive(Debug)]
struct MyStruct;
impl Foo for MyStruct {}
impl Base for MyStruct {}
fn main() {
let s = MyStruct;
let foo: &dyn Foo = &s;
foo.foo();
let base: &dyn Base = foo.as_base();
base.base();
}
Downcasting
Downcasting refers to converting a trait object back to its original concrete type. Rust provides the Any
trait to achieve this.
pub trait Any: 'static {
fn type_id(&self) -> TypeId;
}
Most types implement Any
, except those that contain non-'static
references. Using type_id
, we can determine the type at runtime. Here's an example:
use std::any::Any;
trait Greeting {
fn greeting(&self) -> &str;
fn as_any(&self) -> &dyn Any;
}
struct Cat;
impl Greeting for Cat {
fn greeting(&self) -> &str {
"Meow!"
}
fn as_any(&self) -> &dyn Any {
self
}
}
fn main() {
let cat = Cat;
let g: &dyn Greeting = &cat;
println!("greeting {}", g.greeting());
// Convert to &Cat
let downcast_cat = g.as_any().downcast_ref::<Cat>().unwrap();
println!("greeting {}", downcast_cat.greeting());
}
The key here is downcast_ref
, whose implementation is:
pub fn downcast_ref<T: Any>(&self) -> Option<&T> {
if self.is::<T>() {
unsafe { Some(&*(self as *const dyn Any as *const T)) }
} else {
None
}
}
As shown, if the type matches, the trait object’s data pointer (the first pointer) is safely cast to a reference of the concrete type using unsafe
code.
Object Safety
In Rust, not all traits can be used as trait objects. To be eligible, a trait must satisfy certain conditions—this is referred to as object safety. The main rules are:
Trait methods cannot return
Self
(i.e., the implementing type).
This is because once an object is converted into a trait object, the original type information is lost, soSelf
becomes indeterminate.Trait methods cannot have generic parameters.
The reason is that monomorphization would generate a large number of function implementations, which could lead to method bloat inside the trait. For example:
trait Trait {
fn foo<T>(&self, on: T);
// more methods
}
// 10 implementations
fn call_foo(thing: Box<Trait>) {
thing.foo(true); // this could be any one of the 10 types above
thing.foo(1);
thing.foo("hello");
}
// Would result in 10 * 3 = 30 different implementations
-
Traits used as trait objects must not inherit (have a trait bound)
Sized
. Rust assumes that a trait object implements its trait and generates code like:
trait Foo {
fn method1(&self);
fn method2(&mut self, x: i32, y: String) -> usize;
}
// Autogenerated impl
impl Foo for TraitObject {
fn method1(&self) {
// `self` is a `&Foo` trait object.
// Load the correct function pointer and call it with the opaque data pointer
(self.vtable.method1)(self.data)
}
fn method2(&mut self, x: i32, y: String) -> usize {
// `self` is an `&mut Foo` trait object
// Same as above, passing along the other arguments
(self.vtable.method2)(self.data, x, y)
}
}
If Foo
were to inherit Sized
, then it would require the trait object to also be Sized
. But trait objects are DST (Dynamically Sized Types), meaning they are ?Sized
, and thus the constraint would fail.
For unsafe traits that violate object safety, the best approach is to refactor them into object-safe forms. If that’s not possible, using generics is an alternative workaround.
Conclusion
At the beginning of this article, we introduced that traits are the foundation of zero-cost abstractions. Traits allow you to add new methods to existing types, solving the expression problem, enabling operator overloading, and allowing for interface-oriented programming. It is our hope that this article provides readers with a solid understanding of how to use traits effectively and gives them the confidence to handle compiler errors with ease when working with traits in Rust.
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ