Using Trigonometry to create a circle with C++ and OpenGL | Android Game dev
Table of contents Show me the code The Math My app on the Google play store The app My app's GitHub code The app's GitHub code Basic set up My code is based on THIS hello world triangle tutorial. If you can get that working, you can create the circle Show me the code const int NUM_SEGMENTS = 12; // add more segments to get cleaner circle const float RADIUS = 0.2f; GLfloat circleVertices[(NUM_SEGMENTS + 2) * 2]; // (x, y) pairs void generateCircleVerticesAspectRatioAdjusted(float radius, int numSegments, float aspectRatio) { circleVertices[0] = 0.0f; // Center X circleVertices[1] = 0.0f; // Center Y for (int i = 0; i
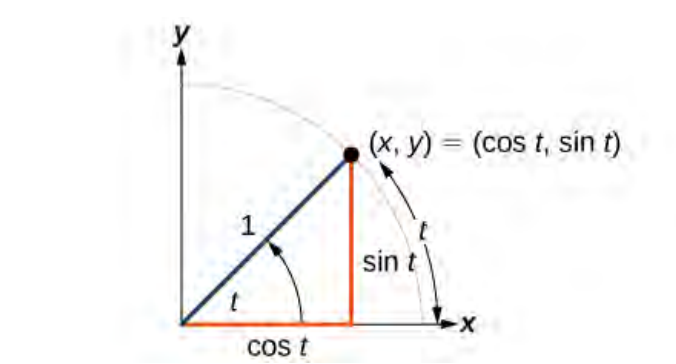
Table of contents
- Show me the code
- The Math
My app on the Google play store
My app's GitHub code
Basic set up
- My code is based on THIS hello world triangle tutorial. If you can get that working, you can create the circle
Show me the code
const int NUM_SEGMENTS = 12; // add more segments to get cleaner circle
const float RADIUS = 0.2f;
GLfloat circleVertices[(NUM_SEGMENTS + 2) * 2]; // (x, y) pairs
void generateCircleVerticesAspectRatioAdjusted(float radius, int numSegments, float aspectRatio) {
circleVertices[0] = 0.0f; // Center X
circleVertices[1] = 0.0f; // Center Y
for (int i = 0; i <= numSegments; i++) {
float theta = (2.0f * M_PI * i) / numSegments;
float x = radius * cosf(theta) / aspectRatio; // Adjust x by aspect ratio
float y = radius * sinf(theta); // y remains unchanged
circleVertices[(i + 1) * 2] = x;
circleVertices[(i + 1) * 2 + 1] = y;
}
}
//CALLED BY THE JNI RENDERER's onSurfaceChanged() method
extern "C"
JNIEXPORT void JNICALL
Java_com_example_clicker_presentation_minigames_dinoRun_TriangleStripJNI_init(JNIEnv *env,
jobject thiz,
jint width,
jint height) {
setupGraphics(width, height);
float aspectRatio = (float)width / (float)height;
LOGI("APSECTrATIOtESTINGaGAIN", "ratio -->%f",aspectRatio);
generateCircleVerticesAspectRatioAdjusted(RADIUS, NUM_SEGMENTS,aspectRatio);
}
- Then to use to code and get it setup you still need all the setup that is described in THIS tutorial
Understanding the code
- We will look at this section of code:
const int NUM_SEGMENTS = 12;
const float RADIUS = 0.2f;
GLfloat circleVertices[(NUM_SEGMENTS + 2) * 2];
So technically what we are doing with this entire code base is using a little trigonometry to create a circle and it all starts with
NUM_SEGMENTS = 12
. Which is how many sides our circle is going to have. If you want a smoother circle you should increase the number.RADIUS
is what we use to define the size of our circle.GLfloat circleVertices[(NUM_SEGMENTS + 2) * 2]
declares our array that will hold our vertices and create the triangles. The+2
is what we use to account for the center and the end of the circle and the*2
to represent the (x,y) valuesNext we will look at:
circleVertices[0] = 0.0f; // Center X
circleVertices[1] = 0.0f; // Center Y
- With the two indexes of 0 and 1 we are defining the center position of our circle
The Maths:
for (int i = 0; i <= numSegments; i++) {
float theta = (2.0f * M_PI * i) / numSegments;
// aspectRatio used to readjust during orientation change
float x = radius * cosf(theta) / aspectRatio;
float y = radius * sinf(theta); // y remains unchanged
circleVertices[(i + 1) * 2] = x;
circleVertices[(i + 1) * 2 + 1] = y;
}
- essentially, we are looping around in a circle and every change in theta we are defining the x and y values for each vertices that will be used to create our circle.
- If you are more of a visual learner, we are essentially doing this for the entire for loop:
- Where the
t
is our theta and our radius is 0.2f. Why does all this math work? Because its based on the Trigonometric properties of a right angle triangle. If the there was no right angle triangle it would not work
Conclusion
- Thank you for taking the time out of your day to read this blog post of mine. If you have any questions or concerns please comment below or reach out to me on Twitter.