Interactive Task Manager
This is a submission for the KendoReact Free Components Challenge. What I Built I built an Interactive Task Manager application. This app allows users to create, view, and manage their tasks with features like setting due dates and filtering by status. It utilizes several KendoReact Free components to provide a polished and user-friendly interface. Demo import React, { useState, useEffect } from 'react'; import { Grid, GridColumn } from '@progress/kendo-react-grid'; import { Input } from '@progress/kendo-react-inputs'; import { DatePicker } from '@progress/kendo-react-dateinputs'; import { Button } from '@progress/kendo-react-buttons'; import { Checkbox } from '@progress/kendo-react-inputs'; import { DropDownList } from '@progress/kendo-react-dropdowns'; import { Card, CardBody, CardTitle } from '@progress/kendo-react-layout'; import { Tooltip } from '@progress/kendo-react-tooltip'; import { ProgressBar } from '@progress/kendo-react-progressbar'; import { Badge } from '@progress/kendo-react-indicators'; const App = () => { const [tasks, setTasks] = useState([ { id: 1, name: 'Grocery Shopping', dueDate: new Date(2025, 2, 20), status: 'Incomplete' }, { id: 2, name: 'Book Appointment', dueDate: new Date(2025, 2, 22), status: 'Complete' }, { id: 3, name: 'Pay Bills', dueDate: new Date(2025, 2, 25), status: 'Incomplete' }, ]); const [newTaskName, setNewTaskName] = useState(''); const [newTaskDueDate, setNewTaskDueDate] = useState(new Date()); const [filterStatus, setFilterStatus] = useState('All'); const taskStatuses = ['All', 'Complete', 'Incomplete']; const handleAddTask = () => { if (newTaskName.trim()) { setTasks([...tasks, { id: Date.now(), name: newTaskName, dueDate: newTaskDueDate, status: 'Incomplete' }]); setNewTaskName(''); } }; const handleStatusChange = (taskId, newStatus) => { setTasks(tasks.map(task => task.id === taskId ? { ...task, status: newStatus } : task )); }; const filteredTasks = filterStatus === 'All' ? tasks : tasks.filter(task => task.status === filterStatus); const completedTasksCount = tasks.filter(task => task.status === 'Complete').length; const totalTasksCount = tasks.length; const completionPercentage = totalTasksCount > 0 ? (completedTasksCount / totalTasksCount) * 100 : 0; return ( Interactive Task Manager setNewTaskName(e.target.value)} /> setNewTaskDueDate(e.target.value)} /> Add Task Filter by Status: setFilterStatus(e.target.value)} /> ( {props.dataItem.name} )} /> ( handleStatusChange(props.dataItem.id, e.target.value)} /> )} /> ); }; export default App; KendoReact Experience I leveraged the following KendoReact Free Components to build the Task Manager: 1️⃣. Grid: The Grid component is used to display the list of tasks in a structured and organized manner, with columns for "Task Name", "Due Date", and "Status". 2️⃣. Input: The Input component allows users to type in the name of a new task they want to add. 3️⃣. DatePicker: The DatePicker component enables users to easily select a due date for their new tasks. 4️⃣. Button: The Button component triggers the action of adding a new task to the list when clicked. 5️⃣. DropDownList: Two DropDownList components are used: One at the top to allow users to filter tasks by their status ("All", "Complete", "Incomplete"). Another within each row of the `Grid` in the "Status" column, allowing users to update the status of individual tasks. 6️⃣. Card: The Card component from @progress/kendo-react-layout is used as a container to wrap the entire Task Manager application, providing a visually distinct section on the page. 7️⃣. Tooltip: The Tooltip component is used to display the full due date of a task when the user hovers over the task name in the Grid. 8️⃣. ProgressBar: The ProgressBar component visually represents the overall completion progress of all tasks. 9️⃣. Badge: While not explicitly used in this basic code for visual representation in the Grid, the Badge component could be easily integrated within the status column to display "Complete" or "Incomplete" with different visual styles. (For sim
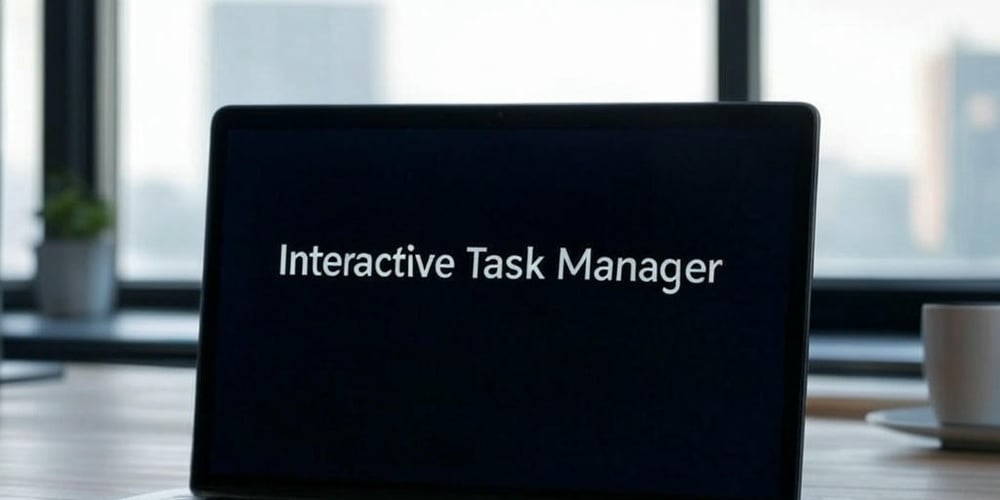
This is a submission for the KendoReact Free Components Challenge.
What I Built
I built an Interactive Task Manager application. This app allows users to create, view, and manage their tasks with features like setting due dates and filtering by status. It utilizes several KendoReact Free components to provide a polished and user-friendly interface.
Demo
import React, { useState, useEffect } from 'react';
import { Grid, GridColumn } from '@progress/kendo-react-grid';
import { Input } from '@progress/kendo-react-inputs';
import { DatePicker } from '@progress/kendo-react-dateinputs';
import { Button } from '@progress/kendo-react-buttons';
import { Checkbox } from '@progress/kendo-react-inputs';
import { DropDownList } from '@progress/kendo-react-dropdowns';
import { Card, CardBody, CardTitle } from '@progress/kendo-react-layout';
import { Tooltip } from '@progress/kendo-react-tooltip';
import { ProgressBar } from '@progress/kendo-react-progressbar';
import { Badge } from '@progress/kendo-react-indicators';
const App = () => {
const [tasks, setTasks] = useState([
{ id: 1, name: 'Grocery Shopping', dueDate: new Date(2025, 2, 20), status: 'Incomplete' },
{ id: 2, name: 'Book Appointment', dueDate: new Date(2025, 2, 22), status: 'Complete' },
{ id: 3, name: 'Pay Bills', dueDate: new Date(2025, 2, 25), status: 'Incomplete' },
]);
const [newTaskName, setNewTaskName] = useState('');
const [newTaskDueDate, setNewTaskDueDate] = useState(new Date());
const [filterStatus, setFilterStatus] = useState('All');
const taskStatuses = ['All', 'Complete', 'Incomplete'];
const handleAddTask = () => {
if (newTaskName.trim()) {
setTasks([...tasks, { id: Date.now(), name: newTaskName, dueDate: newTaskDueDate, status: 'Incomplete' }]);
setNewTaskName('');
}
};
const handleStatusChange = (taskId, newStatus) => {
setTasks(tasks.map(task =>
task.id === taskId ? { ...task, status: newStatus } : task
));
};
const filteredTasks = filterStatus === 'All'
? tasks
: tasks.filter(task => task.status === filterStatus);
const completedTasksCount = tasks.filter(task => task.status === 'Complete').length;
const totalTasksCount = tasks.length;
const completionPercentage = totalTasksCount > 0 ? (completedTasksCount / totalTasksCount) * 100 : 0;
return (
Interactive Task Manager
setNewTaskName(e.target.value)}
/>
setNewTaskDueDate(e.target.value)} />
setFilterStatus(e.target.value)}
/>
(
{props.dataItem.name}
)} />
(
handleStatusChange(props.dataItem.id, e.target.value)}
/>
)}
/>
);
};
export default App;
KendoReact Experience
I leveraged the following KendoReact Free Components to build the Task Manager:
1️⃣. Grid: The Grid
component is used to display the list of tasks in a structured and organized manner, with columns for "Task Name", "Due Date", and "Status".
2️⃣. Input: The Input
component allows users to type in the name of a new task they want to add.
3️⃣. DatePicker: The DatePicker
component enables users to easily select a due date for their new tasks.
4️⃣. Button: The Button
component triggers the action of adding a new task to the list when clicked.
5️⃣. DropDownList: Two DropDownList
components are used:
One at the top to allow users to filter tasks by their status ("All", "Complete", "Incomplete").
Another within each row of the `Grid` in the "Status" column, allowing users to update the status of individual tasks.
6️⃣. Card: The Card
component from @progress/kendo-react-layout
is used as a container to wrap the entire Task Manager application, providing a visually distinct section on the page.
7️⃣. Tooltip: The Tooltip
component is used to display the full due date of a task when the user hovers over the task name in the Grid
.
8️⃣. ProgressBar: The ProgressBar
component visually represents the overall completion progress of all tasks.
9️⃣. Badge: While not explicitly used in this basic code for visual representation in the Grid, the Badge
component could be easily integrated within the status column to display "Complete" or "Incomplete" with different visual styles. (For simplicity in this basic example, a DropDownList
is used for status update).