Bridging Java and AI Systems with Model Integration Protocol
Overview The Model Integration Protocol (MIP) is a powerful framework designed to create seamless integration between Java services and AI systems. It functions by converting Java methods, classes, and services into AI-consumable tools using a modified JSON-RPC format. The core strength of MIP lies in its ability to automatically handle the conversion of existing Java objects—including fields, arrays, maps, and nested objects—into a standardized format that AI systems can understand and utilize. Key Features Automatic Conversion of Java Services Leverages reflection and annotations to expose Java methods and classes as tools for AI Transforms Java classes, methods, and objects into JSON-RPC compliant tools Makes Java functionality directly accessible to LLMs (Large Language Models) Annotation-Based Mapping Uses specialized annotations like @Action, @Prompt, @ListType, and @MapKeyType These annotations define field types, array handling, and date formatting behaviors Simplifies the integration process through declarative programming Complex Structure Support Handles nested objects, arrays, maps, and custom date formats Ensures full compatibility with AI systems regardless of data complexity Eliminates the need for manual serialization/deserialization Cross-Platform Compatibility Uses modified JSON-RPC format that works across multiple platforms Enables integration with various Java-based backends How MIP Works Input Annotations: Java classes and methods are annotated with MIP-specific annotations Reflection: MIP inspects the Java class and its annotations to extract necessary details JSON-RPC Conversion: The class or method is converted into a modified JSON-RPC format AI Consumption: AI systems can directly call these methods using JSON-RPC requests Example Java Class: @Service @Log @Agent public class CompareMiniVanService { public CompareMiniVanService() { log.info("created compare car service"); } @Action(description = "compare two minivan") public String compareMiniVan(String car1, String car2) { log.info(car2); log.info(car1); // implement the comparison logic here return " this is better - "+car2; } } Converted JSON-RPC: { "actionType": "JAVAMETHOD", "actionParameters": { "methodName": "compareMiniVan", "parameters": [ { "name": "car1", "type": "String", "fieldValue": "" }, { "name": "car2", "type": "String", "fieldValue": "" } ], "returnType": "String" }, "actionClass": "io.github.vishalmysore.service.CompareMiniVanService", "description": "compare two minivan", "actionGroup": "No Group", "actionName": "compareMiniVan", "expanded": true } Key Advantages Simplified Integration Eliminates the need to build separate API layers or new REST servers Directly exposes existing Spring services, HTTP calls, and shell scripts Reduced Boilerplate Uses reflection and annotations to auto-generate schemas Makes maintenance, expansion, and scaling significantly easier Unlike alternatives that require more extensive coding and separate servers Cross-LLM Compatibility Works with any LLM that supports JSON-RPC (OpenAI, Claude, Gemini, etc.) Platform-agnostic design ensures broad applicability Implementation Server Neurocaster-Server serves as the reference implementation of MIP Exposes existing Java classes as JSON-RPC tools with minimal configuration Available at http://localhost:8081/actuator/tools4ai-tools when included in a Spring Boot project Client Neurocaster-Client built in Angular Allows chatting and invoking MIP tools via a WebSocket interface WebSocket Configuration To enable tool invocation in a Spring Boot project: @Configuration @EnableWebSocket public class NeuroCasterWebSocketConfig implements WebSocketConfigurer { @Override public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) { registry.addHandler(webSocketHandler(), "/chat") .addInterceptors(new HttpSessionHandshakeInterceptor()) .setAllowedOrigins("*"); // Allow all origins or specific ones } @Bean public WebSocketHandler webSocketHandler() { return new NeuroCasterChatEndpoint(); // Use the ChatEndpoint class as WebSocketHandler } @Bean public ServerEndpointExporter serverEndpointExporter() { return new ServerEndpointExporter(); } } Conclusion The Model Integration Protocol represents a significant advancement in connecting Java applications with AI systems. By automating the conversion process through annotations and reflection, MIP dramatically reduces the development overhead traditionally associated with A
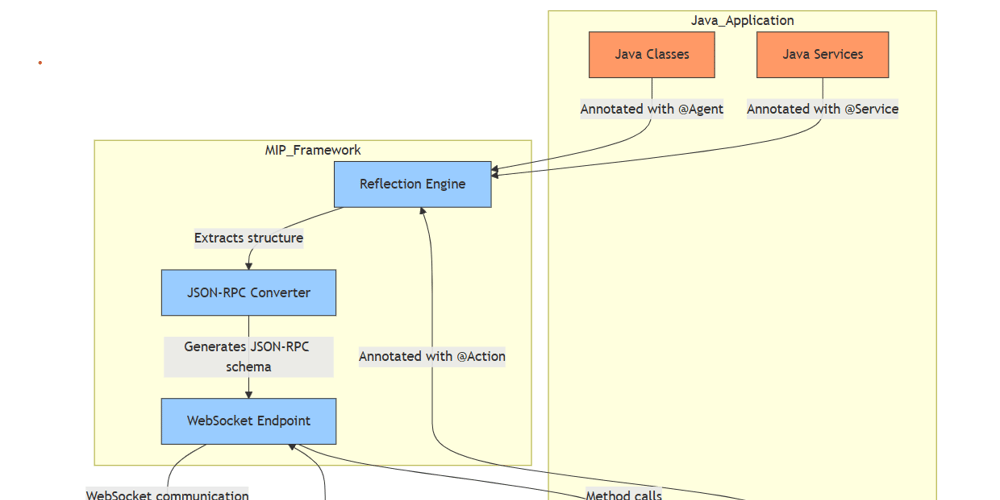
Overview
The Model Integration Protocol (MIP) is a powerful framework designed to create seamless integration between Java services and AI systems. It functions by converting Java methods, classes, and services into AI-consumable tools using a modified JSON-RPC format. The core strength of MIP lies in its ability to automatically handle the conversion of existing Java objects—including fields, arrays, maps, and nested objects—into a standardized format that AI systems can understand and utilize.
Key Features
Automatic Conversion of Java Services
- Leverages reflection and annotations to expose Java methods and classes as tools for AI
- Transforms Java classes, methods, and objects into JSON-RPC compliant tools
- Makes Java functionality directly accessible to LLMs (Large Language Models)
Annotation-Based Mapping
- Uses specialized annotations like
@Action
,@Prompt
,@ListType
, and@MapKeyType
- These annotations define field types, array handling, and date formatting behaviors
- Simplifies the integration process through declarative programming
Complex Structure Support
- Handles nested objects, arrays, maps, and custom date formats
- Ensures full compatibility with AI systems regardless of data complexity
- Eliminates the need for manual serialization/deserialization
Cross-Platform Compatibility
- Uses modified JSON-RPC format that works across multiple platforms
- Enables integration with various Java-based backends
How MIP Works
- Input Annotations: Java classes and methods are annotated with MIP-specific annotations
- Reflection: MIP inspects the Java class and its annotations to extract necessary details
- JSON-RPC Conversion: The class or method is converted into a modified JSON-RPC format
- AI Consumption: AI systems can directly call these methods using JSON-RPC requests
Example
Java Class:
@Service
@Log
@Agent
public class CompareMiniVanService {
public CompareMiniVanService() {
log.info("created compare car service");
}
@Action(description = "compare two minivan")
public String compareMiniVan(String car1, String car2) {
log.info(car2);
log.info(car1);
// implement the comparison logic here
return " this is better - "+car2;
}
}
Converted JSON-RPC:
{
"actionType": "JAVAMETHOD",
"actionParameters": {
"methodName": "compareMiniVan",
"parameters": [
{
"name": "car1",
"type": "String",
"fieldValue": ""
},
{
"name": "car2",
"type": "String",
"fieldValue": ""
}
],
"returnType": "String"
},
"actionClass": "io.github.vishalmysore.service.CompareMiniVanService",
"description": "compare two minivan",
"actionGroup": "No Group",
"actionName": "compareMiniVan",
"expanded": true
}
Key Advantages
Simplified Integration
- Eliminates the need to build separate API layers or new REST servers
- Directly exposes existing Spring services, HTTP calls, and shell scripts
Reduced Boilerplate
- Uses reflection and annotations to auto-generate schemas
- Makes maintenance, expansion, and scaling significantly easier
- Unlike alternatives that require more extensive coding and separate servers
Cross-LLM Compatibility
- Works with any LLM that supports JSON-RPC (OpenAI, Claude, Gemini, etc.)
- Platform-agnostic design ensures broad applicability
Implementation
Server
- Neurocaster-Server serves as the reference implementation of MIP
- Exposes existing Java classes as JSON-RPC tools with minimal configuration
- Available at
http://localhost:8081/actuator/tools4ai-tools
when included in a Spring Boot project
Client
- Neurocaster-Client built in Angular
- Allows chatting and invoking MIP tools via a WebSocket interface
WebSocket Configuration
To enable tool invocation in a Spring Boot project:
@Configuration
@EnableWebSocket
public class NeuroCasterWebSocketConfig implements WebSocketConfigurer {
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(webSocketHandler(), "/chat")
.addInterceptors(new HttpSessionHandshakeInterceptor())
.setAllowedOrigins("*"); // Allow all origins or specific ones
}
@Bean
public WebSocketHandler webSocketHandler() {
return new NeuroCasterChatEndpoint(); // Use the ChatEndpoint class as WebSocketHandler
}
@Bean
public ServerEndpointExporter serverEndpointExporter() {
return new ServerEndpointExporter();
}
}
Conclusion
The Model Integration Protocol represents a significant advancement in connecting Java applications with AI systems. By automating the conversion process through annotations and reflection, MIP dramatically reduces the development overhead traditionally associated with AI integration. This enables developers to focus on core functionality while ensuring their Java services can be effortlessly consumed by modern AI systems.