Calculating Days Between Dates with Python: A Simple Guide
Have you ever needed to calculate how many days have passed since a specific date? Whether you're tracking project timelines, counting down to an important event, or just curious about time intervals, Python makes this calculation remarkably easy. In this article, I'll show you how to use Python's built-in datetime module to calculate the number of days between any date and today. Let's dive in! The Power of Python's datetime Module Python's datetime module is a powerful tool for handling dates, times, and time intervals. It provides classes for manipulating dates and times in both simple and complex ways. Basic Calculation: Days Between a Date and Today Here's a simple function to calculate how many days have passed since a specific date: from datetime import datetime, date def days_since(year, month, day): """Calculate days between a specific date and today.""" past_date = date(year, month, day) today = date.today() delta = today - past_date return delta.days # Example usage days = days_since(2023, 1, 1) print(f"Days since January 1, 2023: {days}") This function takes a year, month, and day as input, creates a date object, and calculates the difference between that date and today. Working with Date Strings Often, you'll have dates in string format. Here's how to handle those: from datetime import datetime def days_since_date_string(date_string, format="%Y-%m-%d"): """Calculate days between a date string and today.""" past_date = datetime.strptime(date_string, format).date() today = date.today() delta = today - past_date return delta.days # Example usage days = days_since_date_string("2023-01-01") print(f"Days since January 1, 2023: {days}") The strptime() method parses a string according to the format you specify, converting it to a datetime object. Handling Future Dates What if the date you're calculating is in the future? Let's modify our function to handle this case: def days_between(target_date_str, format="%Y-%m-%d"): """Calculate absolute days between a date string and today.""" target_date = datetime.strptime(target_date_str, format).date() today = date.today() delta = abs((today - target_date).days) if today > target_date: return f"{delta} days have passed since {target_date_str}" elif today
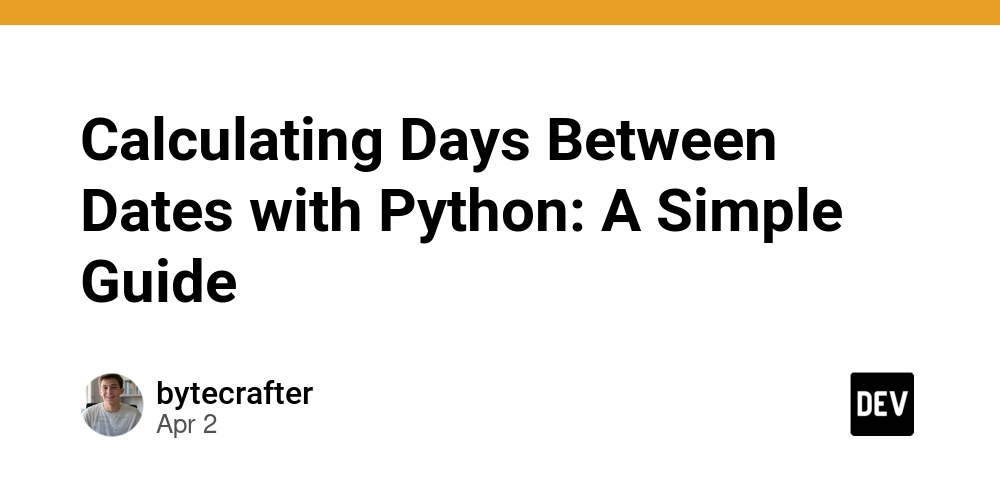
Have you ever needed to calculate how many days have passed since a specific date? Whether you're tracking project timelines, counting down to an important event, or just curious about time intervals, Python makes this calculation remarkably easy.
In this article, I'll show you how to use Python's built-in datetime
module to calculate the number of days between any date and today. Let's dive in!
The Power of Python's datetime
Module
Python's datetime
module is a powerful tool for handling dates, times, and time intervals. It provides classes for manipulating dates and times in both simple and complex ways.
Basic Calculation: Days Between a Date and Today
Here's a simple function to calculate how many days have passed since a specific date:
from datetime import datetime, date
def days_since(year, month, day):
"""Calculate days between a specific date and today."""
past_date = date(year, month, day)
today = date.today()
delta = today - past_date
return delta.days
# Example usage
days = days_since(2023, 1, 1)
print(f"Days since January 1, 2023: {days}")
This function takes a year, month, and day as input, creates a date
object, and calculates the difference between that date and today.
Working with Date Strings
Often, you'll have dates in string format. Here's how to handle those:
from datetime import datetime
def days_since_date_string(date_string, format="%Y-%m-%d"):
"""Calculate days between a date string and today."""
past_date = datetime.strptime(date_string, format).date()
today = date.today()
delta = today - past_date
return delta.days
# Example usage
days = days_since_date_string("2023-01-01")
print(f"Days since January 1, 2023: {days}")
The strptime()
method parses a string according to the format you specify, converting it to a datetime
object.
Handling Future Dates
What if the date you're calculating is in the future? Let's modify our function to handle this case:
def days_between(target_date_str, format="%Y-%m-%d"):
"""Calculate absolute days between a date string and today."""
target_date = datetime.strptime(target_date_str, format).date()
today = date.today()
delta = abs((today - target_date).days)
if today > target_date:
return f"{delta} days have passed since {target_date_str}"
elif today < target_date:
return f"{delta} days until {target_date_str}"
else:
return "That's today!"
# Examples
print(days_between("2023-01-01")) # Past date
print(days_between("2025-02-14")) # Future date
Real-World Application
This type of calculation is incredibly useful for:
- Project management (days since project start/days until deadline)
- Personal events (anniversaries, days until birthdays)
- Habit tracking (days since quitting smoking, days exercising consecutively)
- Financial applications (days until loan payoff, days since last payment)
Online Tools for Date Calculations
While Python gives us the flexibility to calculate these intervals programmatically, sometimes you just need a quick answer. There are many online tools that can help with this.
For instance, if you're curious about how many days remain until Valentine's Day 2025, you could use a tool like How many days since February 14, 2025 instead of writing a Python script.
These online calculators can be convenient for one-off calculations, but when you need to process multiple dates or integrate date calculations into a larger application, Python remains the superior choice.
Advanced Techniques
For more complex date handling, Python offers additional functionality:
# Working with time zones
from datetime import datetime
import pytz
def days_since_with_timezone(date_str, timezone="UTC"):
"""Calculate days since a date with timezone awareness."""
tz = pytz.timezone(timezone)
past_date = tz.localize(datetime.strptime(date_str, "%Y-%m-%d")).date()
today = datetime.now(tz).date()
return (today - past_date).days
# Example
days = days_since_with_timezone("2023-01-01", "America/New_York")
print(f"Days since January 1, 2023 in New York: {days}")
Conclusion
Python's datetime
module provides elegant solutions for date calculations. Whether you're building a complex application or just need to satisfy your curiosity about a time interval, these simple techniques will serve you well.
Remember that while functions like these calculate calendar days, you might sometimes want to calculate business days or customize the calculation in other ways. Python's flexibility makes these customizations straightforward.
Happy coding!