Day-12: Data Types
EXAMPLE: int myNum = 5; // Integer (whole number) float myFloatNum = 5.99f; // Floating point number char myLetter = 'D'; // Character boolean myBool = true; // Boolean String myText = "Hello"; // String Primitive DataTypes: Data Type Description byte -Stores whole numbers from -128 to 127 short -Stores whole numbers from -32,768 to 32,767 int -Stores whole numbers from -2,147,483,648 to 2,147,483,647 long -Stores whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 float -Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits double -Stores fractional numbers. Sufficient for storing 15 to 16 decimal digits boolean -Stores true or false values char -Stores a single character/letter or ASCII values Assignment operator:("=") assigning right side value to left side variable. Data type: int num = 50. Here, int is the data type for the variable num. what is reference variable? Some of the data types are primitive and the rest are non-primitive. All the non-primitive data types are derived from primitive data types and are also known as reference types. The variable declared with non-primitive data types is known as reference variables. String:string is non primitive data type .string is a class. IQ:(Interview question) Tell me the primitive datatypes in java? In Java, the eight primitive data types are byte, short, int, long, float, double, boolean, and char. How many primitive datatype are there in java? The eight primitive data types supported by the Java programming language are: byte: The byte data type is an 8-bit signed two's complement integer. How do you create object in java? use the new keyword followed by the class name and optional constructor arguments if the class has a constructor. How do you assign value to variable in java? The = symbol is known as the assignment operator
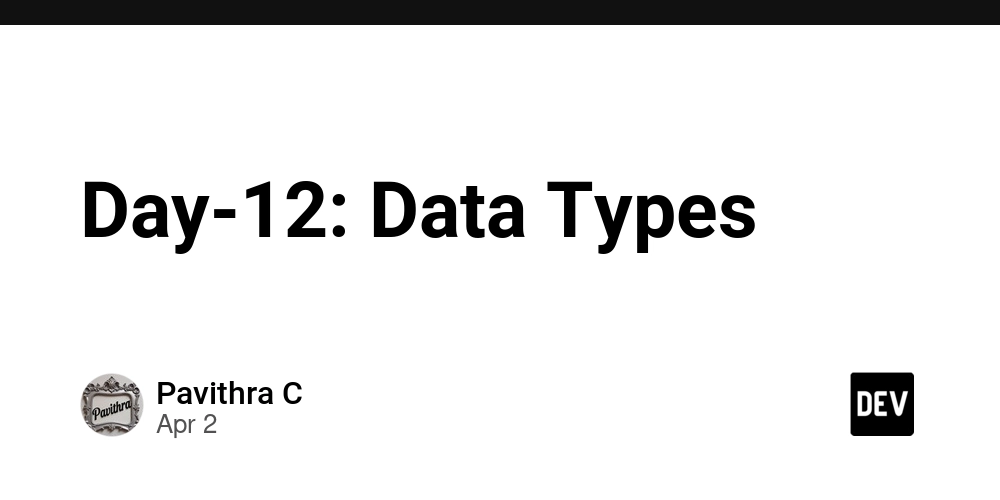
EXAMPLE:
int myNum = 5; // Integer (whole number)
float myFloatNum = 5.99f; // Floating point number
char myLetter = 'D'; // Character
boolean myBool = true; // Boolean
String myText = "Hello"; // String
Primitive DataTypes:
Data Type Description
- byte -Stores whole numbers from -128 to 127
- short -Stores whole numbers from -32,768 to 32,767
- int -Stores whole numbers from -2,147,483,648 to 2,147,483,647
- long -Stores whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
- float -Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits
- double -Stores fractional numbers. Sufficient for storing 15 to 16 decimal digits
- boolean -Stores true or false values
- char -Stores a single character/letter or ASCII values
Assignment operator:("=")
assigning right side value to left side variable.
Data type:
int num = 50. Here, int is the data type for the variable num.
what is reference variable?
Some of the data types are primitive and the rest are non-primitive. All the non-primitive data types are derived from primitive data types and are also known as reference types. The variable declared with non-primitive data types is known as reference variables.
String:string is non primitive data type .string is a class.
IQ:(Interview question)
Tell me the primitive datatypes in java?
In Java, the eight primitive data types are byte, short, int, long, float, double, boolean, and char.How many primitive datatype are there in java?
The eight primitive data types supported by the Java programming language are: byte: The byte data type is an 8-bit signed two's complement integer.How do you create object in java?
use the new keyword followed by the class name and optional constructor arguments if the class has a constructor.How do you assign value to variable in java?
The = symbol is known as the assignment operator