Day 4: Methods,static & Non static.
How to Call an Object in Java? In Java, we call or access an object by: Creating an object of a class using the new keyword. Calling methods and variables using the object. Syntax to Create an Object $ ClassName objectName = new ClassName(); $ ClassName → Name of the class. $ objectName → Name of the object. $ new → Allocates memory and creates the object. $ ClassName() → Calls the constructor of the class. Example: Object Creation and Method Calling class Student { void display() { // Method System.out.println("Hello, I am a Student!"); } public static void main(String[] args) { Student obj = new Student(); // Object creation obj.display(); // Calling method using object } } Output: Hello, I am a Student! Example: Calling Instance Variables and Methods class Car { String brand = "Toyota"; // Instance variable void showBrand() { // Method System.out.println("Car Brand: " + brand); } public static void main(String[] args) { Car myCar = new Car(); // Creating object System.out.println(myCar.brand); // Accessing variable myCar.showBrand(); // Calling method } } Output: Toyota Car Brand: Toyota Why Use Methods? $ Code Reusability – Write once, use multiple times. $ Improves Readability – Organizes code into small, logical parts. $ Reduces Redundancy – Avoids writing the same code repeatedly. $ Easier Debugging – Makes it easy to find and fix errors. Syntax of a Method returnType methodName(parameters) { // method body return value; // If returnType is not void } returnType– Specifies the type of value the method returns (int, String, void, etc.). methodName – The name of the method. parameters – Input values (optional). return– Used to return a value (optional for void methods). Example 1: Method Without Parameters and Return Type class Example { void greet() { // No parameters, no return System.out.println("Hello, Welcome!"); } public static void main(String[] args) { Example obj = new Example(); obj.greet(); // Calling the method } } Output: Hello, Welcome! Calling Static and Non-Static Methods 1. Static $ Declared using the static keyword. $ Belongs to the class rather than a specific instance. $ Shared among all objects of the class. $ Can be accessed without creating an object. $ Cannot access non-static members directly. Calling Static Methods (Without Object) class Example { static void show() { // Static method System.out.println("Static Method Called"); } public static void main(String[] args) { Example.show(); // Calling static method } } Output: Static Method Called 2. Non-Static (Instance) $ Does not use the static keyword. $ Belongs to a specific object of the class. $ Each object has its own copy of instance variables. $ Can access both static and non-static members. Calling Non-Static Methods (Using Object) class Example { void show() { // Non-static method System.out.println("Non-Static Method Called"); } public static void main(String[] args) { Example obj = new Example(); // Creating object obj.show(); // Calling non-static method } } Output: Non-Static Method Called Key Points ✅ Static methods can be called without an object. ✅ Non-static methods and variables require an object to be accessed. ✅ new keyword is used to create an object in Java. Global and Local Variables in Java In Java, variables can have different scopes based on where they are declared. The two main types are: Global Variables (Instance/Class Variables) Local Variables 1. Global Variables (Instance or Class Variables) Declared outside of methods, inside the class. Can be accessed by all methods in the class. Can be instance variables (different for each object) or static variables (shared among all objects). Example: Global (Instance) Variable class Example { int num = 10; // Global variable (Instance variable) void display() { System.out.println("Number: " + num); // Accessible in all methods } public static void main(String[] args) { Example obj = new Example(); obj.display(); } } Output: Number: 10 Example: Global (Static) Variable class Example { static int count = 5; // Global static variable public static void main(String[] args) { System.out.println("Count: " + count); // No need to create an object } } Output: Count: 5 2. Local Variables $ Declared inside a method, constructor, or block. $ Can be accessed only within the method where they are declared. $ Cannot have static keyword. Example: Local Variable class Example { void show() { int x = 20; // Local variable System.out.println("Value of x: " + x);
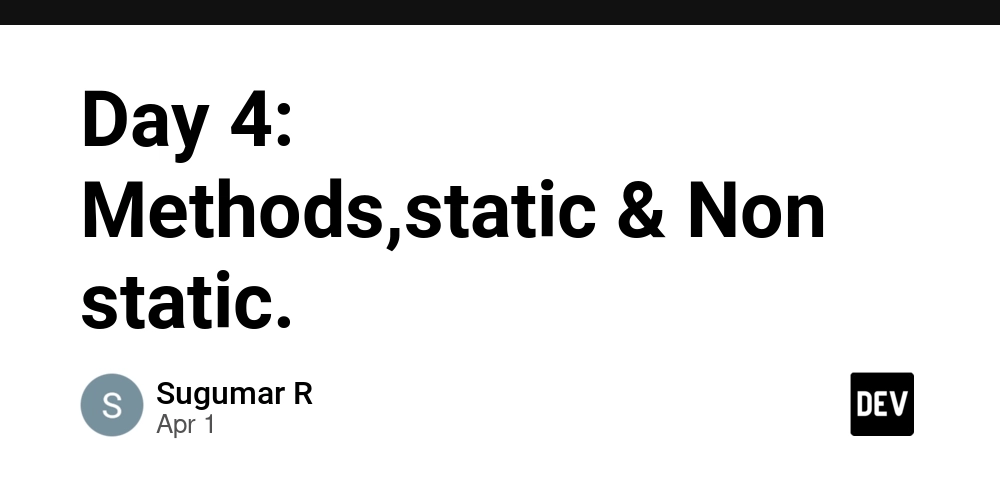
How to Call an Object in Java?
In Java, we call or access an object by:
Creating an object of a class using the new keyword.
Calling methods and variables using the object.
Syntax to Create an Object
$ ClassName objectName = new ClassName();
$ ClassName → Name of the class.
$ objectName → Name of the object.
$ new → Allocates memory and creates the object.
$ ClassName() → Calls the constructor of the class.
Example: Object Creation and Method Calling
class Student {
void display() { // Method
System.out.println("Hello, I am a Student!");
}
public static void main(String[] args) {
Student obj = new Student(); // Object creation
obj.display(); // Calling method using object
}
}
Output:
Hello, I am a Student!
Example: Calling Instance Variables and Methods
class Car {
String brand = "Toyota"; // Instance variable
void showBrand() { // Method
System.out.println("Car Brand: " + brand);
}
public static void main(String[] args) {
Car myCar = new Car(); // Creating object
System.out.println(myCar.brand); // Accessing variable
myCar.showBrand(); // Calling method
}
}
Output:
Toyota
Car Brand: Toyota
Why Use Methods?
$ Code Reusability – Write once, use multiple times.
$ Improves Readability – Organizes code into small, logical parts.
$ Reduces Redundancy – Avoids writing the same code repeatedly.
$ Easier Debugging – Makes it easy to find and fix errors.
Syntax of a Method
returnType methodName(parameters) {
// method body
return value; // If returnType is not void
}
returnType– Specifies the type of value the method returns (int, String, void, etc.).
methodName – The name of the method.
parameters – Input values (optional).
return– Used to return a value (optional for void methods).
Example 1: Method Without Parameters and Return Type
class Example {
void greet() { // No parameters, no return
System.out.println("Hello, Welcome!");
}
public static void main(String[] args) {
Example obj = new Example();
obj.greet(); // Calling the method
}
}
Output:
Hello, Welcome!
Calling Static and Non-Static Methods
1. Static
$ Declared using the static keyword.
$ Belongs to the class rather than a specific instance.
$ Shared among all objects of the class.
$ Can be accessed without creating an object.
$ Cannot access non-static members directly.
Calling Static Methods (Without Object)
class Example {
static void show() { // Static method
System.out.println("Static Method Called");
}
public static void main(String[] args) {
Example.show(); // Calling static method
}
}
Output:
Static Method Called
2. Non-Static (Instance)
$ Does not use the static keyword.
$ Belongs to a specific object of the class.
$ Each object has its own copy of instance variables.
$ Can access both static and non-static members.
Calling Non-Static Methods (Using Object)
class Example {
void show() { // Non-static method
System.out.println("Non-Static Method Called");
}
public static void main(String[] args) {
Example obj = new Example(); // Creating object
obj.show(); // Calling non-static method
}
}
Output:
Non-Static Method Called
Key Points
✅ Static methods can be called without an object.
✅ Non-static methods and variables require an object to be accessed.
✅ new keyword is used to create an object in Java.
Global and Local Variables in Java
In Java, variables can have different scopes based on where they are declared. The two main types are:
Global Variables (Instance/Class Variables)
Local Variables
1. Global Variables (Instance or Class Variables)
Declared outside of methods, inside the class.
Can be accessed by all methods in the class.
Can be instance variables (different for each object) or static variables (shared among all objects).
Example: Global (Instance) Variable
class Example {
int num = 10; // Global variable (Instance variable)
void display() {
System.out.println("Number: " + num); // Accessible in all methods
}
public static void main(String[] args) {
Example obj = new Example();
obj.display();
}
}
Output:
Number: 10
Example: Global (Static) Variable
class Example {
static int count = 5; // Global static variable
public static void main(String[] args) {
System.out.println("Count: " + count); // No need to create an object
}
}
Output:
Count: 5
2. Local Variables
$ Declared inside a method, constructor, or block.
$ Can be accessed only within the method where they are declared.
$ Cannot have static keyword.
Example: Local Variable
class Example {
void show() {
int x = 20; // Local variable
System.out.println("Value of x: " + x);
}
public static void main(String[] args) {
Example obj = new Example();
obj.show();
// System.out.println(x); // Error! 'x' is not accessible here
}
}
Output:
Value of x: 20
Key Differences Between Global and Local Variables.