Go Learning Journey: Part 1 - Setting Up and First Impressions
Go's, like, everywhere—cloud computing, backend services... you name it! It's known for being simple, super fast, and handling lots of things at once. Tons of developers swear by it. But, is it really that easy for beginners? Well, instead of just following some boring tutorial, I'm getting my hands dirty—learning Go and sharing what I find, the good and the bad, as I go. Setting Up Go (Easier Than I Thought!) Setting up Go is pretty straightforward, and here's how you can do it on different operating systems. Installing on Linux Download Go: Grab the latest Go archive from the official downloads page. You can use wgetin your terminal: wget https://go.dev/dl/go1.21.6.linux-amd64.tar.gz # Replace with the actual version Extract the archive: Extract the downloaded archive to /usr/local: sudo tar -C /usr/local -xzf go1.21.6.linux-amd64.tar.gz Set up environment variables: Add Go's binary directory to your PATH by adding this line to your ~/.profile or ~/.bashrc file export PATH=$PATH:/usr/local/go/bin Then, apply the changes: source ~/.profile or source ~/.bashrc Verify installation: Check if Go is installed correctly: go version Installing on macOS Download Go: Download the .pkg installer from the official Go downloads page. Install Go: Run the .pkg file and follow the instructions. Go will be installed to /usr/local/go. Set up environment variables: Add Go to your PATH by adding this line to your ~/.bash_profile or ~/.zshrc file: echo "export PATH=$PATH:/usr/local/go/bin" >> ~/.bash_profile source ~/.bash_profile Verify Installation: Run the following command go version Installing on Windows Download Go: Download the .msi installer from the official Go downloads page. Install Go: Run the .msi installer and follow the instructions. Set up Environment Variables: The installer usually sets the necessary environment variables. If not, add C:\Program Files\Go\bin to the Path variable in your system environment settings. Verify Installation: Open a command prompt and type: go version Writing My First Go Program Now that Go is set up, let's write a simple program to make sure everything's working and to get a feel for the Go language. It's tradition to start with a "Hello, World" program, so that's what we'll do! Create the Go file: Now, we'll create a file to hold our Go code. We'll name it main.go. You can use any text editor you like (VS Code, Notepad, Nano, Vim, etc.). Inside your text editor, type the following Go code package main import "fmt" func main() { fmt.Println("Hello, World!") } package main: This line declares the package that this code belongs to. Every Go program must belong to a package. The main package is special; it tells the Go compiler that this code is the entry point of an executable program. import "fmt": This line imports the fmt package, which provides functions for formatted input and output. In this case, we're using the fmt.Println function. func main() { ... }: This is the main function. It's the function that gets executed when you run the program. The code inside the curly braces {} is what the function does. fmt.Println("Hello, World!"): This line is the heart of the program. It calls the Println function from the fmt package to print the text "Hello, World!" to the console. Println adds a newline character to the end of the output. Save the file: Save the main.go file in your text editor. If you're using Nano, you can press Ctrl+O to save, and then Ctrl+X to exit. Run the program: Now, let's run the program! In your terminal or command prompt type the following command: go run main.go This command does two things: go run: This is the Go command that compiles and runs the Go program. main.go: This specifies the Go file that you want to run. When you press Enter, you should see the output: Hello, World! Congratulations! You've just written and run your first Go program.
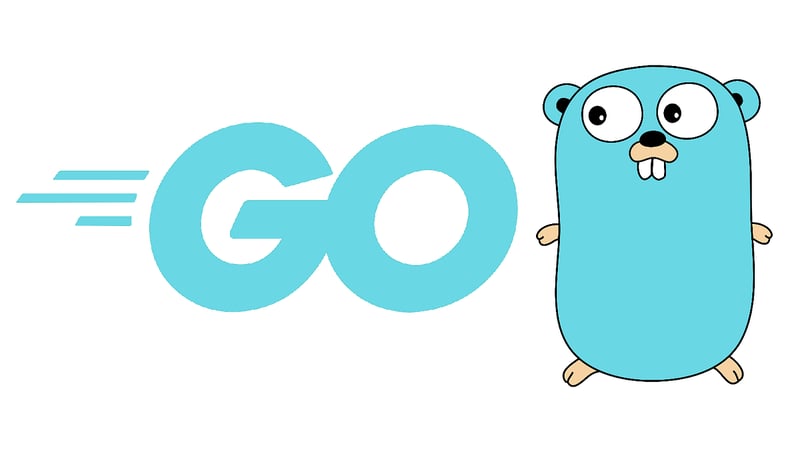
Go's, like, everywhere—cloud computing, backend services... you name it! It's known for being simple, super fast, and handling lots of things at once. Tons of developers swear by it. But, is it really that easy for beginners? Well, instead of just following some boring tutorial, I'm getting my hands dirty—learning Go and sharing what I find, the good and the bad, as I go.
Setting Up Go (Easier Than I Thought!)
Setting up Go is pretty straightforward, and here's how you can do it on different operating systems.
Installing on Linux
Download Go: Grab the latest Go archive from the official downloads page. You can use wget
in your terminal:
wget https://go.dev/dl/go1.21.6.linux-amd64.tar.gz # Replace with the actual version
Extract the archive: Extract the downloaded archive to /usr/local
:
sudo tar -C /usr/local -xzf go1.21.6.linux-amd64.tar.gz
Set up environment variables: Add Go's binary directory to your PATH by adding this line to your ~/.profile
or ~/.bashrc
file
export PATH=$PATH:/usr/local/go/bin
Then, apply the changes:
source ~/.profile
or source ~/.bashrc
Verify installation: Check if Go is installed correctly:
go version
Installing on macOS
Download Go: Download the .pkg
installer from the official Go downloads page.
Install Go: Run the .pkg
file and follow the instructions. Go will be installed to /usr/local/go
.
Set up environment variables: Add Go to your PATH by adding this line to your ~/.bash_profile
or ~/.zshrc
file:
echo "export PATH=$PATH:/usr/local/go/bin" >> ~/.bash_profile source ~/.bash_profile
Verify Installation: Run the following command
go version
Installing on Windows
Download Go: Download the .msi
installer from the official Go downloads page.
Install Go: Run the .msi
installer and follow the instructions.
Set up Environment Variables: The installer usually sets the necessary environment variables. If not, add C:\Program Files\Go\bin
to the Path variable in your system environment settings.
Verify Installation: Open a command prompt and type:
go version
Writing My First Go Program
Now that Go is set up, let's write a simple program to make sure everything's working and to get a feel for the Go language. It's tradition to start with a "Hello, World" program, so that's what we'll do!
Create the Go file: Now, we'll create a file to hold our Go code. We'll name it main.go
. You can use any text editor you like (VS Code, Notepad, Nano, Vim, etc.). Inside your text editor, type the following Go code
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
package main: This line declares the package that this code belongs to. Every Go program must belong to a package. The main package is special; it tells the Go compiler that this code is the entry point of an executable program.
import "fmt": This line imports the fmt package, which provides functions for formatted input and output. In this case, we're using the fmt.Println function.
func main() { ... }: This is the main function. It's the function that gets executed when you run the program. The code inside the curly braces {} is what the function does.
fmt.Println("Hello, World!"): This line is the heart of the program. It calls the Println function from the fmt package to print the text "Hello, World!" to the console. Println adds a newline character to the end of the output.
Save the file: Save the main.go file in your text editor. If you're using Nano, you can press Ctrl+O to save, and then Ctrl+X to exit.
Run the program: Now, let's run the program! In your terminal or command prompt type the following command:
go run main.go
This command does two things:
go run: This is the Go command that compiles and runs the Go program.
main.go: This specifies the Go file that you want to run.
When you press Enter, you should see the output:
Hello, World!
Congratulations! You've just written and run your first Go program.