How to Be a Senior PHP Developer (Without Faking It)
So, you’ve been coding in PHP for a while, and you’re wondering, “What does it take to be a senior PHP developer?” Sure, you could just wait until you have enough years under your belt, but let’s be real—years don’t equal skill. A senior developer isn’t just someone with experience; it’s someone who knows how to use that experience wisely. Here’s what makes the difference. 1. Master the Art of Debugging (Not Just Googling Errors) A junior dev sees an error and pastes it into Google. A senior dev knows how to truly debug. This means: Understanding stack traces and following them logically. Using breakpoints instead of dumping variables everywhere. Knowing how to isolate the issue rather than randomly tweaking code. Want to level up? Try solving issues without searching for them immediately. Dig into the PHP documentation. Challenge yourself. 2. Think Architecture, Not Just Code Anyone can write working PHP code. But does your code scale? Does it follow SOLID principles? Are you thinking about maintainability? Senior devs don’t just focus on “does it work?” but also “will it still work a year from now?” Learn: Design patterns (Factory, Singleton, Repository, etc.). How to write modular code. Why bad architecture leads to technical debt and how to avoid it. 3. Become a Database Whisperer A senior PHP dev doesn’t just write queries; they optimize them. Know when to use indexes and why they matter. Avoid N+1 query problems like the plague. Learn about database caching, transactions, and normalization. Get comfortable with both SQL and NoSQL (sometimes MongoDB is a better fit!). Bonus tip: Run EXPLAIN on your queries and see how MySQL processes them. You’ll learn a lot! 4. Automate Yourself Out of Repetitive Work A good developer writes code. A great developer writes code that writes code. Use Composer for dependency management. Automate testing (unit, integration, functional) with PHPUnit and Pest. Learn bash scripts or tools like Ansible to automate deployments. When you find yourself repeating the same task over and over, stop and think: “Can I automate this?” 5. Understand Security Beyond the Basics “Don’t trust user input” isn’t enough. A senior dev knows security vulnerabilities inside and out: SQL Injection (and why prepared statements are a must!) XSS (Cross-Site Scripting) and how to sanitize inputs correctly. CSRF (Cross-Site Request Forgery) and how to prevent it. Why exposing stack traces in production is a bad idea. Get into the mindset of a hacker—because if you don’t, someone else will. 6. Code Reviews: Give and Take Like a Pro A senior dev isn’t just good at writing code, but also at reviewing it. Instead of just pointing out what’s wrong, explain why. Learn to spot performance issues before they happen. Encourage best practices without being a code dictator. Accept feedback with an open mind (you’re not perfect, and that’s okay!). 7. Know When Not to Use PHP It sounds weird, but hear me out: just because you can do something in PHP doesn’t mean you should. If you need real-time processing, maybe WebSockets with Node.js is better. If you’re building a static site, why not use a headless CMS or a Jamstack approach? Sometimes microservices with Go, Rust, or Python make more sense. A senior developer knows when PHP is the right tool—and when it’s not. 8. Be the Dev Others Want to Work With Technical skills will get you the title, but soft skills will make you a real senior dev. Mentor juniors instead of gatekeeping knowledge. Communicate clearly—whether in meetings, comments, or documentation. Take ownership of problems instead of blaming others. Wrapping Up Being a senior PHP developer isn’t just about experience or writing complex code. It’s about solving problems effectively, thinking long-term, and making life easier for your future self and your team. So, don’t just count your years of experience—make them count. What’s one skill that helped YOU grow as a developer? Drop it in the comments!
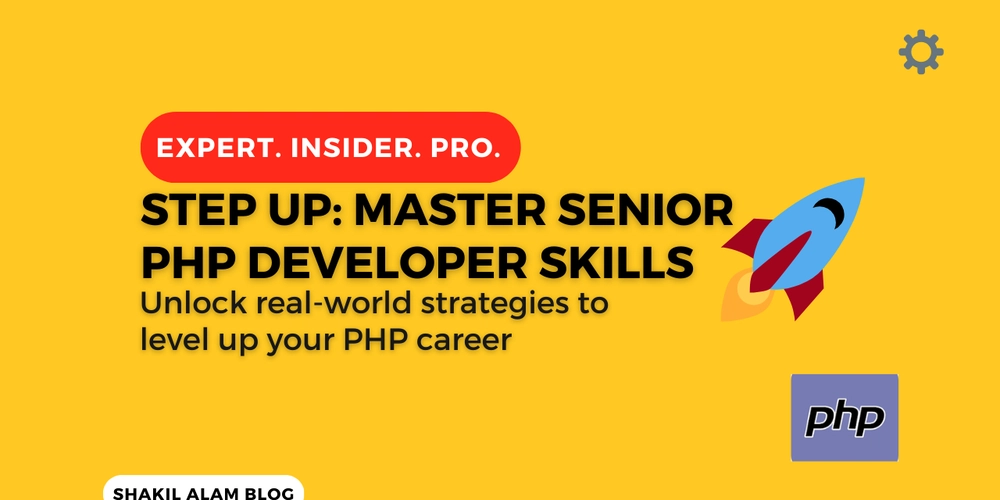
So, you’ve been coding in PHP for a while, and you’re wondering, “What does it take to be a senior PHP developer?”
Sure, you could just wait until you have enough years under your belt, but let’s be real—years don’t equal skill. A senior developer isn’t just someone with experience; it’s someone who knows how to use that experience wisely.
Here’s what makes the difference.
1. Master the Art of Debugging (Not Just Googling Errors)
A junior dev sees an error and pastes it into Google. A senior dev knows how to truly debug. This means:
- Understanding stack traces and following them logically.
- Using breakpoints instead of dumping variables everywhere.
- Knowing how to isolate the issue rather than randomly tweaking code.
Want to level up? Try solving issues without searching for them immediately. Dig into the PHP documentation. Challenge yourself.
2. Think Architecture, Not Just Code
Anyone can write working PHP code. But does your code scale? Does it follow SOLID principles? Are you thinking about maintainability?
Senior devs don’t just focus on “does it work?” but also “will it still work a year from now?” Learn:
- Design patterns (Factory, Singleton, Repository, etc.).
- How to write modular code.
- Why bad architecture leads to technical debt and how to avoid it.
3. Become a Database Whisperer
A senior PHP dev doesn’t just write queries; they optimize them.
- Know when to use indexes and why they matter.
- Avoid N+1 query problems like the plague.
- Learn about database caching, transactions, and normalization.
- Get comfortable with both SQL and NoSQL (sometimes MongoDB is a better fit!).
Bonus tip: Run EXPLAIN
on your queries and see how MySQL processes them. You’ll learn a lot!
4. Automate Yourself Out of Repetitive Work
A good developer writes code. A great developer writes code that writes code.
- Use Composer for dependency management.
- Automate testing (unit, integration, functional) with PHPUnit and Pest.
- Learn bash scripts or tools like Ansible to automate deployments.
When you find yourself repeating the same task over and over, stop and think: “Can I automate this?”
5. Understand Security Beyond the Basics
“Don’t trust user input” isn’t enough. A senior dev knows security vulnerabilities inside and out:
- SQL Injection (and why prepared statements are a must!)
- XSS (Cross-Site Scripting) and how to sanitize inputs correctly.
- CSRF (Cross-Site Request Forgery) and how to prevent it.
- Why exposing stack traces in production is a bad idea.
Get into the mindset of a hacker—because if you don’t, someone else will.
6. Code Reviews: Give and Take Like a Pro
A senior dev isn’t just good at writing code, but also at reviewing it.
- Instead of just pointing out what’s wrong, explain why.
- Learn to spot performance issues before they happen.
- Encourage best practices without being a code dictator.
- Accept feedback with an open mind (you’re not perfect, and that’s okay!).
7. Know When Not to Use PHP
It sounds weird, but hear me out: just because you can do something in PHP doesn’t mean you should.
- If you need real-time processing, maybe WebSockets with Node.js is better.
- If you’re building a static site, why not use a headless CMS or a Jamstack approach?
- Sometimes microservices with Go, Rust, or Python make more sense.
A senior developer knows when PHP is the right tool—and when it’s not.
8. Be the Dev Others Want to Work With
Technical skills will get you the title, but soft skills will make you a real senior dev.
- Mentor juniors instead of gatekeeping knowledge.
- Communicate clearly—whether in meetings, comments, or documentation.
- Take ownership of problems instead of blaming others.
Wrapping Up
Being a senior PHP developer isn’t just about experience or writing complex code. It’s about solving problems effectively, thinking long-term, and making life easier for your future self and your team.
So, don’t just count your years of experience—make them count.
What’s one skill that helped YOU grow as a developer? Drop it in the comments!