HTTP Requests
HTTP Requests have some common request types: /GET: request.get(): is used to retrieve data from a server. GET https://api.user.org/users/?id=AAA /POST: request.post(): is used when sending data to the server to create new resources. POST /api/users Content-Type: application/json { "id": "AAA", "name": "John Doe", "email": "john@testmail.com" } /PUT: request.put(): is used to update or replace a resource on the server. PUT /api/users/AAA Content-Type: application/json { "name": "AAA Smith", "email": "aaa@testmail.com" } /DELETE: request.delete(): is used to remove or delete a resource on the server. DELETE /api/users/AAA Sample Python code: Remember to create a .env file with TOKE, USERNAME, and ENDPOINT. import os import dotenv import requests PIXELA_TOKEN = os.getenv("PIXELA_TOKEN") PIXELA_USERNAME = os.getenv("PIXELA_USERNAME") PIXELA_ENDPOINT = os.getenv("PIXELA_ENDPOINT") headers = { "X-USER-TOKEN": PIXELA_TOKEN } user_param = { "token": PIXELA_TOKEN, "username": PIXELA_USERNAME, "agreeTermsOfService": "yes", "notMinor": "yes" } response = requests.post(url=PIXELA_ENDPOINT, json=user_param) print(response.text) ### {"message":"Success. Let's visit ...
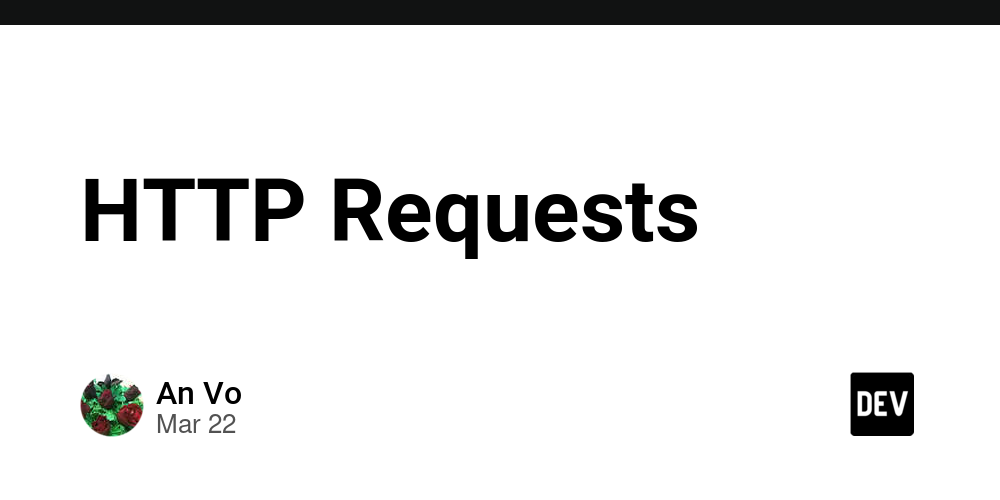
HTTP Requests have some common request types:
- /GET: request.get(): is used to retrieve data from a server.
GET https://api.user.org/users/?id=AAA
- /POST: request.post(): is used when sending data to the server to create new resources.
POST /api/users
Content-Type: application/json
{
"id": "AAA",
"name": "John Doe",
"email": "john@testmail.com"
}
- /PUT: request.put(): is used to update or replace a resource on the server.
PUT /api/users/AAA
Content-Type: application/json
{
"name": "AAA Smith",
"email": "aaa@testmail.com"
}
- /DELETE: request.delete(): is used to remove or delete a resource on the server.
DELETE /api/users/AAA
- Sample Python code: Remember to create a .env file with TOKE, USERNAME, and ENDPOINT.
import os
import dotenv
import requests
PIXELA_TOKEN = os.getenv("PIXELA_TOKEN")
PIXELA_USERNAME = os.getenv("PIXELA_USERNAME")
PIXELA_ENDPOINT = os.getenv("PIXELA_ENDPOINT")
headers = {
"X-USER-TOKEN": PIXELA_TOKEN
}
user_param = {
"token": PIXELA_TOKEN,
"username": PIXELA_USERNAME,
"agreeTermsOfService": "yes",
"notMinor": "yes"
}
response = requests.post(url=PIXELA_ENDPOINT, json=user_param)
print(response.text)
### {"message":"Success. Let's visit ...