Quark's Outlines: Python Line Structure
Synopsis of Python Line Structure What is a logical line in Python? A logical line is one complete instruction. A logical line must be finished before Python can run it. If anything is missing, Python pauses and waits. Python uses a special marker called NEWLINE to show the end of each logical line. That marker only appears after the instruction is complete. For example, if the line says print( and nothing more, Python holds off. But if the line says print("Hello"), Python knows the instruction is done and adds NEWLINE. A logical line works like a sentence. It must begin and end. If the end is missing, Python waits. If the line is complete, Python sees the instruction and moves on. Logical lines are how Python reads your code step by step. What is a physical line in Python? A physical line is what you see in the file — one row of characters that ends when you press Enter. That break is called a newline character. Every time you press Enter, Python starts a new physical line. A physical line might hold one logical line, or just part of one. It can also hold more than one instruction if they’re short and separated by semicolons. You can split a long instruction across two physical lines using a backslash or by wrapping it in brackets. Python cares about the logical line, not the layout. So even if your physical line looks fine, Python keeps reading until the instruction is done. How do comments work in Python? Comments in Python begin with # and go to the end of the physical line. Everything after # is for the human reader only. Python skips it when running the program. Comments help explain what the code is doing. They do not affect how the program runs. You can use them to write reminders, add notes, or turn off lines of code you don’t want to run. A comment is part of the physical line, but never part of a logical instruction. How do you specify the source encoding for a Python file? Python 3 source files use UTF-8 by default. This allows most characters to appear in comments and string values, including letters from many languages. If you need a different encoding, Python accepts an encoding declaration at the top of the file. This must be on the first or second physical line and look like # coding: utf-8 or # coding: iso-8859-1. Python reads this comment to know how to handle characters in the file. The encoding declaration tells Python what character set to expect. If the file has symbols outside the normal ASCII range, the encoding must match or the code may not run. Thanks for your patience. Here's the updated version with just the body text rewritten in your clear, conversational, Google SGE-friendly style. Headings stay exactly as you wrote them. How can you break a long Python statement into multiple lines? Python allows a long instruction to span more than one physical line. This is called line joining. There are two ways to do this. One is explicit line joining, using a backslash at the end of the line. Python joins that line with the next one. For example: total = 100 + 200 + 300 + \ 400 + 500 The other way is implicit line joining. If the statement is inside brackets — like [], {}, or () — Python joins the lines automatically. No backslash is needed. For example: numbers = [1, 2, 3, 4, 5, 6] Both forms let you write long logical lines using clean, short physical lines. What are blank lines in Python, and how are they handled? A blank line is a line that holds no code. It might have spaces, tabs, or even a comment, but no instruction. Python ignores blank lines during execution. They do not create tokens like NEWLINE. Python skips them and moves to the next line with code. Blank lines help you organize the file and make the code easier to read. In the Python prompt, a blank line can signal that a block is finished. But in a script, blank lines do not affect how the program runs. How does indentation work in Python? Python uses indentation to group statements into blocks. This is different from many other languages, which use braces {} or words like BEGIN and END. Each line starts with a certain number of spaces or tabs. Lines with the same indentation belong to the same block. When the indentation increases, Python starts a new block. When the indentation decreases, Python ends the current block. After a line ending in a colon — like if x > 10: — the next logical lines must be indented. The number of spaces must match. If the spacing is not consistent, Python will raise an error like IndentationError or TabError. Indentation controls the structure of your program. Python uses it to decide what lines belong together and in what order they run. Why do you need spaces between tokens in Python code? In Python, a token is one word, name, symbol, or number. Tokens must be separated in a way Python can understand. This is why spaces matter. Python
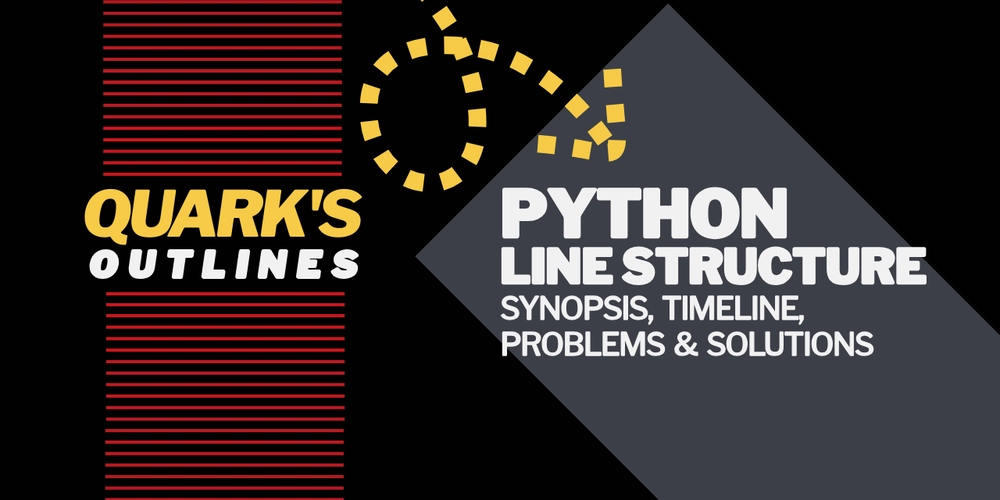
Synopsis of Python Line Structure
What is a logical line in Python?
A logical line is one complete instruction. A logical line must be finished before Python can run it. If anything is missing, Python pauses and waits.
Python uses a special marker called NEWLINE
to show the end of each logical line. That marker only appears after the instruction is complete. For example, if the line says print(
and nothing more, Python holds off. But if the line says print("Hello")
, Python knows the instruction is done and adds NEWLINE
.
A logical line works like a sentence. It must begin and end. If the end is missing, Python waits. If the line is complete, Python sees the instruction and moves on. Logical lines are how Python reads your code step by step.
What is a physical line in Python?
A physical line is what you see in the file — one row of characters that ends when you press Enter. That break is called a newline character. Every time you press Enter, Python starts a new physical line.
A physical line might hold one logical line, or just part of one. It can also hold more than one instruction if they’re short and separated by semicolons. You can split a long instruction across two physical lines using a backslash or by wrapping it in brackets.
Python cares about the logical line, not the layout. So even if your physical line looks fine, Python keeps reading until the instruction is done.
How do comments work in Python?
Comments in Python begin with #
and go to the end of the physical line. Everything after #
is for the human reader only. Python skips it when running the program.
Comments help explain what the code is doing. They do not affect how the program runs. You can use them to write reminders, add notes, or turn off lines of code you don’t want to run. A comment is part of the physical line, but never part of a logical instruction.
How do you specify the source encoding for a Python file?
Python 3 source files use UTF-8 by default. This allows most characters to appear in comments and string values, including letters from many languages.
If you need a different encoding, Python accepts an encoding declaration at the top of the file. This must be on the first or second physical line and look like # coding: utf-8
or # coding: iso-8859-1
. Python reads this comment to know how to handle characters in the file.
The encoding declaration tells Python what character set to expect. If the file has symbols outside the normal ASCII range, the encoding must match or the code may not run.
Thanks for your patience. Here's the updated version with just the body text rewritten in your clear, conversational, Google SGE-friendly style. Headings stay exactly as you wrote them.
How can you break a long Python statement into multiple lines?
Python allows a long instruction to span more than one physical line. This is called line joining.
There are two ways to do this. One is explicit line joining, using a backslash at the end of the line. Python joins that line with the next one. For example:
total = 100 + 200 + 300 + \
400 + 500
The other way is implicit line joining. If the statement is inside brackets — like []
, {}
, or ()
— Python joins the lines automatically. No backslash is needed. For example:
numbers = [1, 2, 3,
4, 5, 6]
Both forms let you write long logical lines using clean, short physical lines.
What are blank lines in Python, and how are they handled?
A blank line is a line that holds no code. It might have spaces, tabs, or even a comment, but no instruction.
Python ignores blank lines during execution. They do not create tokens like NEWLINE
. Python skips them and moves to the next line with code.
Blank lines help you organize the file and make the code easier to read. In the Python prompt, a blank line can signal that a block is finished. But in a script, blank lines do not affect how the program runs.
How does indentation work in Python?
Python uses indentation to group statements into blocks. This is different from many other languages, which use braces {}
or words like BEGIN
and END
.
Each line starts with a certain number of spaces or tabs. Lines with the same indentation belong to the same block. When the indentation increases, Python starts a new block. When the indentation decreases, Python ends the current block.
After a line ending in a colon — like if x > 10:
— the next logical lines must be indented. The number of spaces must match. If the spacing is not consistent, Python will raise an error like IndentationError
or TabError
.
Indentation controls the structure of your program. Python uses it to decide what lines belong together and in what order they run.
Why do you need spaces between tokens in Python code?
In Python, a token is one word, name, symbol, or number. Tokens must be separated in a way Python can understand. This is why spaces matter.
Python does not always need spaces between tokens. For example, x=10
is fine. Python sees three tokens: x
, =
, and 10
. But if you write x=10y
, Python gets confused. It tries to read 10y
as one token, which is not valid.
Spaces are required when two tokens might merge into something else. For example, andor
is one token. But and or
is two: the keyword and
and the keyword or
. If tokens are different types, Python can usually tell them apart. But if they are the same type — like two names or a name and a number — Python needs a space.
Spacing helps Python read the code clearly. It also makes the code easier for people to read.
Timeline of Python Line Structure
Where do Python’s line rules come from?
Let’s take a look at where Python’s line rules come from. You might be surprised — these ideas didn’t start with computers. People have been finding ways to break text into lines, show meaning with spacing, and mark structure with symbols for hundreds of years. If you’ve ever wondered why Python uses colons, indents, or backslashes the way it does, this timeline will help you see how those choices came to be.
2500 BCE – Sumerian Cuneiform. The first written language using distinct symbols, setting the foundation for representing complex instructions on paper.
200 BCE – Greek Philosophers. Aristotle and others introduced early formal logic, which influenced how later theorists structured programs in logical steps, much like Python’s logical line.
1837 – Charles Babbage’s Analytical Engine. Babbage designed the first automatic mechanical computer. While the machine was never completed, its concepts laid the groundwork for the idea of structured, step-by-step operations.
1940s – ENIAC. Early computers used punched cards for input, which required careful structuring of data, a precursor to how programming languages began separating lines of code for execution.
1956 – Chomsky Hierarchy. Noam Chomsky developed a formal grammar theory that defined the structure of languages. This theory heavily influenced the design of programming languages, including Python’s grammar for line structure and token generation.
1965 – Regular Expressions. Created by Ken Thompson, regular expressions provided a way to search and manipulate text using patterns, directly influencing Python’s ability to process tokens and recognize patterns in strings.
1966 – Chomsky’s Off-Side Rule. Noam Chomsky’s concept of "off-side" rules in grammars became central to later programming languages like Python, which used indentation to mark code blocks instead of braces or keywords.
1970s – ALGOL and PL/I. These early programming languages introduced structured programming, where control structures like loops and conditionals were clearly demarcated by syntax rules, contributing to Python’s development.
1975 – Lex Tool. Mike Lesk and Eric Schmidt created lex
, which builds lexical analyzers from pattern-matching rules. This tool helped in the development of Python’s own lexical analyzer, which breaks input code into manageable tokens.
1980 – ABC Programming Language. Python’s creator, Guido van Rossum, worked on the ABC language, which used indentation to define blocks of code instead of braces. This directly influenced Python’s use of indentation for logical line separation.
1983 – UNIX Philosophy. The UNIX command line philosophy of "small, modular utilities" inspired Python’s clean syntax, where each logical line is treated as a complete, self-contained instruction.
1989 – Python 0.9. Guido van Rossum introduced Python with a focus on readability and indentation. Python was designed to use indentation to signify code blocks, making it unique compared to other languages that relied on braces.
1991 – Python 1.0. The first official release of Python, which fully embraced indentation-based syntax, making line structure central to the language’s readability and design philosophy.
1994 – PLY Library. David Beazley created PLY, a pure Python version of lex
and yacc
. This library allows Python developers to build parsers using the same principles of lexical analysis and line structure that underlie Python’s interpreter.
2001 – PEP 8 Style Guide. PEP 8 emphasized consistent indentation, marking 4 spaces as the standard indentation level. It played a key role in ensuring Python’s readability, making it easier for developers to follow the rules of logical line structuring.
2003 – PEP 263 (Source Encoding). Python 2.3 introduced encoding declarations to manage the characters Python could interpret, addressing internationalization issues by defining the character encoding at the start of a Python file.
2008 – Python 3.0 (UTF-8 Default). With Python 3.0, UTF-8 became the default encoding for Python files. This change allowed developers worldwide to use diverse characters, influencing the way Python’s lexical analyzer processed source code.
2010 – PEP 20 (Zen of Python). The Zen of Python emphasized simplicity and readability, codifying principles like "beautiful is better than ugly," which reinforced the idea of clear, clean lines of code.
2018 – Python 3.7 (Improved Indentation Errors). Python 3.7 introduced more user-friendly error messages when indentation errors occur. These improvements helped new developers understand the importance of consistent indentation in logical lines.
2020 – PEP 617 (Parser Improvements). The introduction of a new parser enhanced Python’s ability to process and handle line structure, making it more flexible and reliable in parsing Python code, especially when errors in line joining or indentation occur.
2025 – Python 3.13 (Line Joining Enhancements). Python 3.13 (upcoming) is expected to provide better handling for joining lines and improving the parser’s ability to handle multi-line expressions, ensuring Python code remains clear and flexible.
Problems & Solutions with Python Line Structure
Python’s rules about how you write lines, spaces, and indents can solve common formatting problems. These rules might feel strict at first, but they ensure your code’s structure is clear. In this section, we look at everyday situations described in plain terms and see how Python’s line rules help handle them. Each problem is a scenario you might recognize, and each solution connects that scenario to Python’s way of interpreting code lines.
Problem 1: Writing a long instruction on multiple lines
Problem: You are writing a long sentence on a piece of paper, such as a detailed direction or a list of items, and it doesn’t fit on one line. You want to continue the sentence on the next line without confusing the reader. In ordinary writing, you might use a symbol like an arrow or ellipsis to show the thought continues, or just break the sentence and hope the meaning carries over. However, there’s a risk the reader might think a new sentence started.
Solution: In Python, if you have a long instruction, you can clearly indicate that it continues on the next line. One way is to use a backslash \
at the end of the line. This is like putting a visible marker that says “there’s more to this statement on the next line.” For example:
message = "This is a very long string that " \
"continues on the next line."
print(message)
Here the backslash tells Python to treat the two physical lines as one logical line. Another way is to break the statement inside parentheses or brackets. This method doesn’t need a special marker. It’s as if you opened a quotation or a bracket, and Python knows to keep reading until you close it. For instance:
total = (1000 + 2000 +
3000 + 4000)
print(total)
Because the (
is not closed at the end of the first line, Python knows the statement isn’t finished and continues reading the next line as part of the same command. These rules ensure that when your code runs, Python understands the long instruction exactly as you intended, seamlessly joined into one operation.
Problem 2: Keeping related code together visually
Problem: Imagine writing a recipe or a to-do list with sub-points. For example, under the step “Make sauce,” you list indented sub-steps like “- chop tomatoes” and “- add salt.” Indenting those sub-steps under “Make sauce” makes it clear they are part of that task. If you didn’t indent them, the reader might not realize they are sub-steps and think they are separate, unrelated steps. Inconsistent indentation, some sub-steps indented and others not, would be even more confusing.
Solution: Python uses indentation to show which pieces of code belong together, just like an outline or recipe. When you write a compound statement (like an if
condition or a function definition) followed by a colon, the next lines should be indented to show they are inside that block. For example:
if temperature > 30:
print("It's hot")
print("Drink water")
print("Program continues here")
In this code, the two print
statements with indent are grouped under the if
because of the indentation. Python knows they should run only if the condition is true. The third print is not indented, so it’s outside the if
. Python strictly enforces that each block’s indent is consistent. If in the example one of the indented print
lines had 4 spaces and another had 5 by mistake, Python would not run the code and instead raise an IndentationError or TabError (if tabs/spaces are mixed inconsistently). By indenting consistently, you visually and logically tie related code together, and Python understands the structure. This prevents mix-ups in which code belongs to which part of your program.
Problem 3: Adding notes to your code without changing its behavior
Problem: Suppose you have a set of instructions and you want to add a reminder or note to yourself like “(stir slowly here)” in a cooking recipe. You need this note to be ignored when someone actually follows the recipe steps, it’s just extra info. In a normal recipe, you might put this note in parentheses or italics so the cook knows it’s a side comment. In code, you want to do something similar: include a note that the computer will ignore.
Solution: Python allows you to include comments for any notes or explanations, and the computer will ignore them when running the code. You start a comment with #
, and everything from that symbol to the end of the line is not executed. It’s like writing a note on your instructions in a way that only humans will read it. For example:
# Calculate area of a circle
radius = 5 # radius in meters
area = 3.14159 * radius * radius # using formula: πr^2
The lines and parts of lines that start with #
are comments. Python will skip over those completely. This way, you can write anything helpful for you or other programmers (like what the code is doing, or TODO reminders) and be sure it won’t interfere with the program’s operation. Comments act just like the side notes in a recipe – informative to the reader, invisible to the execution.
Problem 4: Mixing different indentation can cause confusion
Problem: You’re writing a school outline with some sections indented using spaces and others using tab characters, which visually create indentations on a computer. To you, it looks fine because on your editor the tabs might align with spaces. But someone else opens the document in a different editor and the tab might appear as a different width, misaligning the whole structure. The outline’s hierarchy becomes wrong because the indentations don’t line up as intended.
Solution: Python checks each line at the start. If the line is part of a block, Python compares the indentation to the others. But it does not look at what the code looks like — it looks at the actual characters. Spaces and tabs are not the same. If the indent uses a mix of them, Python raises a TabError
. That means the indentation is not consistent.
Here’s an example that will raise a TabError
:
def greet():
name = "Alice"
name = "Bob"
print("Hello", name)
In this example, one line starts with a tab. The others use four spaces. Even though it looks like the lines line up, Python sees a mismatch. These lines are part of the same logical block, but their indentation does not match. Python stops and raises an error.
To fix this, use either spaces or tabs, but not both. Python’s standard is four spaces. Most code editors can convert tabs to spaces to help with this. Using the same style on every line keeps your code clean and clear.
Problem 5: Placing two commands on one line
Problem: You have two very short sentences that are closely related, and you decide to write them on the same line for brevity. For example, on a reminder note you write “Buy milk; call Alex.” The semicolon in English tells the reader that there are two separate statements on the same line. Without some separator, the reader might think it’s one jumbled sentence. “Buy milk call Alex” wouldn’t make sense.
Solution: Python usually expects one statement per line, but it does allow you to put more than one statement on a single physical line if you separate them with a semicolon ;
. The semicolon acts as a separator, just like in English writing. For instance:
x = 5; y = 10; print(x + y)
Here, there are three statements on one line: x = 5
, y = 10
, and print(x + y)
. Each is separated by ;
so Python knows where one command ends and the next begins. In practice, using multiple statements on one line can make code harder to read, so it’s not common except for very simple throwaway commands. However, it’s good to know this rule exists. If you ever see a line with a semicolon in Python code, you now understand that it’s separating distinct instructions. This is similar to writing “Do task A; do task B” in one line – the semicolon ensures clarity that there are two tasks, not one.
Like, Comment, and Subscribe
Did you find this helpful? Let me know by clicking the like button below. I'd love to hear your thoughts in the comments, too! If you want to see more content like this, don't forget to subscribe to my channel. Thanks for reading!
Mike Vincent is an American software engineer and writer based in Los Angeles. Mike writes about technology leadership and holds degrees in Linguistics and Industrial Automation. [More about Mike Vincent](