Canary Release: A Smart Approach to Software Deployment
In the world of continuous delivery and DevOps, deploying new versions of software in a safe and controlled manner is crucial. One of the most effective strategies for minimizing risk while introducing new features is the Canary Release. This technique allows you to gradually roll out changes to a subset of users before making them available to everyone. In this post, we'll explore the benefits and drawbacks of Canary Releases and provide a practical example using Spring Boot and Spring Cloud Gateway. The History Behind Canary Releases The term Canary Release originates from an old mining practice where miners would carry caged canaries into coal mines to detect toxic gases such as carbon monoxide. Since canaries are more sensitive to these gases than humans, if the bird showed signs of distress or died, it served as an early warning for the miners to evacuate. Similarly, in software deployment, a Canary Release involves exposing a small portion of users to a new version to detect issues before a full rollout, thereby mitigating risk. What is a Canary Release? A Canary Release is a deployment strategy where a new version of an application is rolled out to a small, controlled percentage of users before being fully released. The goal is to test the new version in a real-world scenario while limiting the impact of potential issues. Pros and Cons of Canary Releases ✅ Pros: Reduced Risk: Since only a small percentage of users interact with the new version initially, any major issue will affect only a limited audience. Real-World Feedback: Canary releases allow for collecting real-time performance metrics and user feedback before a full deployment. Faster Rollbacks: If an issue is detected, rolling back to the stable version is straightforward and quick. Gradual Performance Monitoring: By analyzing error rates, latency, and other key metrics, teams can ensure that the new version meets performance expectations before a full rollout. ❌ Cons: Complexity in Traffic Routing: Implementing Canary Releases requires proper infrastructure to direct traffic dynamically, often requiring load balancers, API gateways, or feature flagging tools. Monitoring Overhead: Continuous monitoring and logging are essential to detect issues early, which can add operational complexity. State and Data Consistency Issues: If the new version introduces breaking changes to databases or shared resources, users may face inconsistencies. Implementing a Canary Release with Spring Boot and Spring Cloud Gateway To illustrate a practical Canary Release setup, we'll use Spring Boot to create two APIs: a Stable API Service and a Canary API Service. Spring Cloud Gateway will manage traffic distribution, directing 90% of requests to the stable version and 10% to the canary version. Steps: One - Create Two Spring Boot Applications: stable-api (Current stable version) canary-api (New canary version with changes) Both will be using Spring web dependency. org.springframework.boot spring-boot-starter-web Stable Api will run on port 8081. Stable Api service will have this controller: @RestController @RequestMapping("stable") public class StableController { @GetMapping("/") public ResponseEntity stableResponse() { return ResponseEntity.ok().body("Hello World from Stable Service."); } } Canary Api will run on port 8082. Canary Api service will have this controller: @RestController @RequestMapping("canary") public class CanaryController { @GetMapping("/") public ResponseEntity canaryResponse() { return ResponseEntity.ok().body("Hello World from Canary Service."); } } Two - Set Up Spring Cloud Gateway: Configure routing rules to control traffic split. Create a gateway on Spring initializr with the dependency: org.springframework.cloud spring-cloud-starter-gateway By leveraging Spring Cloud Gateway, we can configure a weighted routing mechanism that ensures a smooth rollout: spring: application: name: gateway_service cloud: gateway: routes: - id: stable-api-service uri: http://localhost:8081 predicates: - Path=/api/** - Weight=group1,90 filters: - RewritePath=/api/(?.*), /stable/${segment} - id: canary-api-service uri: http://localhost:8082 predicates: - Path=/api/** - Weight=group1,10 filters: - RewritePath=/api/(?.*), /canary/${segment} Configuration Explanation Predicate `Path=/api/`** Any request that starts with /api/ will be captured by the Gateway. Predicate Weight=group, X Defines the traffic distribution: 90% of the requests go to the Stable API (http://localhost:8081/stable). 10% of the requests go to the Canary API (http://localhost:9092/canary).
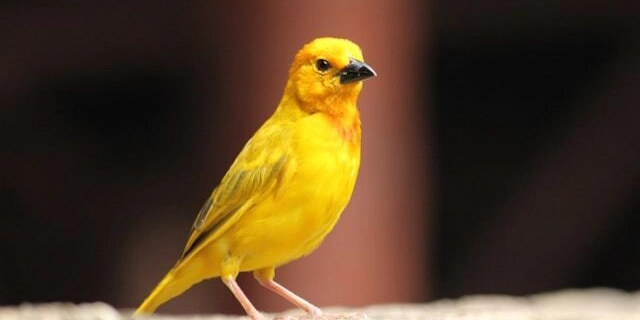
In the world of continuous delivery and DevOps, deploying new versions of software in a safe and controlled manner is crucial. One of the most effective strategies for minimizing risk while introducing new features is the Canary Release. This technique allows you to gradually roll out changes to a subset of users before making them available to everyone. In this post, we'll explore the benefits and drawbacks of Canary Releases and provide a practical example using Spring Boot and Spring Cloud Gateway.
The History Behind Canary Releases
The term Canary Release originates from an old mining practice where miners would carry caged canaries into coal mines to detect toxic gases such as carbon monoxide. Since canaries are more sensitive to these gases than humans, if the bird showed signs of distress or died, it served as an early warning for the miners to evacuate. Similarly, in software deployment, a Canary Release involves exposing a small portion of users to a new version to detect issues before a full rollout, thereby mitigating risk.
What is a Canary Release?
A Canary Release is a deployment strategy where a new version of an application is rolled out to a small, controlled percentage of users before being fully released. The goal is to test the new version in a real-world scenario while limiting the impact of potential issues.
Pros and Cons of Canary Releases
✅ Pros:
- Reduced Risk: Since only a small percentage of users interact with the new version initially, any major issue will affect only a limited audience.
- Real-World Feedback: Canary releases allow for collecting real-time performance metrics and user feedback before a full deployment.
- Faster Rollbacks: If an issue is detected, rolling back to the stable version is straightforward and quick.
- Gradual Performance Monitoring: By analyzing error rates, latency, and other key metrics, teams can ensure that the new version meets performance expectations before a full rollout.
❌ Cons:
- Complexity in Traffic Routing: Implementing Canary Releases requires proper infrastructure to direct traffic dynamically, often requiring load balancers, API gateways, or feature flagging tools.
- Monitoring Overhead: Continuous monitoring and logging are essential to detect issues early, which can add operational complexity.
- State and Data Consistency Issues: If the new version introduces breaking changes to databases or shared resources, users may face inconsistencies.
Implementing a Canary Release with Spring Boot and Spring Cloud Gateway
To illustrate a practical Canary Release setup, we'll use Spring Boot to create two APIs: a Stable API Service and a Canary API Service. Spring Cloud Gateway will manage traffic distribution, directing 90% of requests to the stable version and 10% to the canary version.
Steps:
One - Create Two Spring Boot Applications:
-
stable-api
(Current stable version) -
canary-api
(New canary version with changes)
Both will be using Spring web dependency.
org.springframework.boot
spring-boot-starter-web
Stable Api will run on port 8081.
Stable Api service will have this controller:
@RestController
@RequestMapping("stable")
public class StableController {
@GetMapping("/")
public ResponseEntity<String> stableResponse() {
return ResponseEntity.ok().body("Hello World from Stable Service.");
}
}
Canary Api will run on port 8082.
Canary Api service will have this controller:
@RestController
@RequestMapping("canary")
public class CanaryController {
@GetMapping("/")
public ResponseEntity<String> canaryResponse() {
return ResponseEntity.ok().body("Hello World from Canary Service.");
}
}
Two - Set Up Spring Cloud Gateway: Configure routing rules to control traffic split.
Create a gateway on Spring initializr with the dependency:
org.springframework.cloud
spring-cloud-starter-gateway
By leveraging Spring Cloud Gateway, we can configure a weighted routing mechanism that ensures a smooth rollout:
spring:
application:
name: gateway_service
cloud:
gateway:
routes:
- id: stable-api-service
uri: http://localhost:8081
predicates:
- Path=/api/**
- Weight=group1,90
filters:
- RewritePath=/api/(?.*), /stable/${segment}
- id: canary-api-service
uri: http://localhost:8082
predicates:
- Path=/api/**
- Weight=group1,10
filters:
- RewritePath=/api/(?.*), /canary/${segment}
Configuration Explanation
-
Predicate `Path=/api/`**
- Any request that starts with
/api/
will be captured by the Gateway.
- Any request that starts with
-
Predicate
Weight=group, X
- Defines the traffic distribution:
-
90% of the requests go to the Stable API (
http://localhost:8081/stable
). -
10% of the requests go to the Canary API (
http://localhost:9092/canary
).
-
Filter
RewritePath=/api/(?
.*), /stable/${segment} - This rewrites the URL so that requests to
/api/something
are redirected to/stable/something
in the Stable service.
- This rewrites the URL so that requests to
-
Filter
RewritePath=/api/(?
.*), /canary/${segment} - Similarly, this rewrites the URL so that requests are redirected to
/canary/something
in the Canary service.
- Similarly, this rewrites the URL so that requests are redirected to
This configuration ensures that 90% of requests go to the stable API, while 10% are routed to the canary API.
Conclusion
Canary Releases offer a powerful way to deploy software updates incrementally, reducing risks while ensuring new features perform as expected. By using Spring Boot and Spring Cloud Gateway, implementing a Canary Release becomes straightforward and manageable.