SOLID design principles in Game Development
This post is an excerpt from the book Learning Game Architecture with Unity Design principles in game development are fundamental guidelines that dictate the process of creating clean, maintainable, and scalable code. They are essential for crafting robust, adaptable, and high-quality software systems. These principles act as the building blocks upon which developers construct their code, guiding the architectural decisions and coding practices to achieve better code quality and maintainability. By following design principles, developers create code that is more adaptable to changes, as it becomes easier to refactor, debug, and extend without causing unintended consequences or introducing bugs. These principles encourage a structured approach to coding, promoting separation of concerns, maintainability, and code that is easier to test and debug. Need for software design principles Imagine a team developing a complex multiplayer game. Initially, the code lacks adherence to clean code principles. As the project advances, new features like character customization, interactive environments, and diverse gameplay mechanics are added, causing the codebase to grow in complexity. Problems without clean code practices would be: Maintenance nightmare: Developers find it hard to make changes or add features without inadvertently causing issues elsewhere. Even a small modification could lead to unexpected bugs in other parts of the game. Increased bugs: With intricate and tangled code, the likelihood of introducing bugs rises significantly. The complexity makes it harder to test thoroughly, leading to more hidden issues. Collaboration hurdles: The team struggles to collaborate efficiently due to the lack of clear code. Understanding and integrating contributions from different developers becomes time-consuming and error-prone. Scalability challenges: As the game evolves, maintaining and extending functionalities becomes increasingly cumbersome. The codebase becomes a hindrance, slowing down the addition of new features. Popular software design principles Clean code transcends language-specific rules; it applies universally across diverse software languages, even those used in gaming projects. These design principles remain language-agnostic in their application. Refer to the following figure: SOLID principles: This set of five principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion) focuses on creating more maintainable, flexible, and understandable software systems. Keep It Simple, Stupid (KISS): Emphasizes simplicity in design and development, advocating for straightforward solutions over complex ones. Don't Repeat Yourself (DRY): Encourages the elimination of redundant code to enhance maintainability by reusing existing code. You Aren't Gonna Need It (YAGNI): Advises against adding functionality or code until it is necessary, avoiding unnecessary complexities. Among the pantheon of widely recognized design principles, the SOLID principles stand as a bedrock, renowned for their effectiveness in shaping resilient and scalable software architectures. SOLID design principles The SOLID principles, a fundamental set of design principles in software engineering, encapsulate a structured approach to crafting robust, maintainable, and adaptable codebases. Coined by Robert C. Martin, these principles serve as foundational pillars guiding developers toward writing flexible, scalable, and comprehensible software. Here is a concise summary of each principle's role: Single Responsibility Principle (SRP): Encourages each class or module to have a single responsibility, ensuring clarity and maintainability. Open/Closed Principle (OCP): Fosters code that is open for extension but closed for modification, enabling new functionalities without altering existing code. Liskov Substitution Principle (LSP): Ensures that objects of a superclass can be replaced by objects of its subclasses without affecting the program's functionality. Interface Segregation Principle (ISP): Promotes smaller, cohesive interfaces rather than large, all-encompassing ones, preventing clients from depending on interfaces they do not use. Dependency Inversion Principle (DIP): Encourages abstraction and decoupling by depending on abstractions rather than concrete implementations, facilitating flexibility and scalability. SOLID design principles and their usage are explained in the the context of game development with great details in the book: Learning Game Architecture with Unity, which is available @ Amazon, Amazon India, BPB International, BPB India.
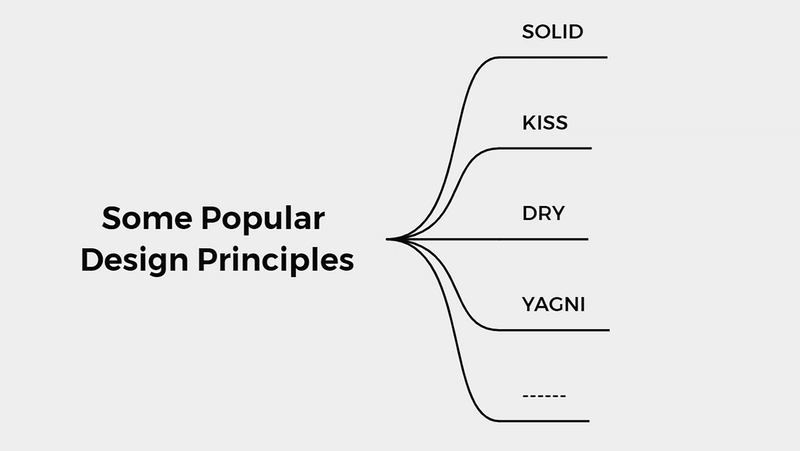
This post is an excerpt from the book Learning Game Architecture with Unity
Design principles in game development are fundamental guidelines that dictate the process of creating clean, maintainable, and scalable code. They are essential for crafting robust, adaptable, and high-quality software systems. These principles act as the building blocks upon which developers construct their code, guiding the architectural decisions and coding practices to achieve better code quality and maintainability.
By following design principles, developers create code that is more adaptable to changes, as it becomes easier to refactor, debug, and extend without causing unintended consequences or introducing bugs. These principles encourage a structured approach to coding, promoting separation of concerns, maintainability, and code that is easier to test and debug.
Need for software design principles
Imagine a team developing a complex multiplayer game. Initially, the code lacks adherence to clean code principles. As the project advances, new features like character customization, interactive environments, and diverse gameplay mechanics are added, causing the codebase to grow in complexity.
Problems without clean code practices would be:
- Maintenance nightmare: Developers find it hard to make changes or add features without inadvertently causing issues elsewhere. Even a small modification could lead to unexpected bugs in other parts of the game.
- Increased bugs: With intricate and tangled code, the likelihood of introducing bugs rises significantly. The complexity makes it harder to test thoroughly, leading to more hidden issues.
- Collaboration hurdles: The team struggles to collaborate efficiently due to the lack of clear code. Understanding and integrating contributions from different developers becomes time-consuming and error-prone.
- Scalability challenges: As the game evolves, maintaining and extending functionalities becomes increasingly cumbersome. The codebase becomes a hindrance, slowing down the addition of new features.
Popular software design principles
Clean code transcends language-specific rules; it applies universally across diverse software languages, even those used in gaming projects. These design principles remain language-agnostic in their application. Refer to the following figure:
- SOLID principles: This set of five principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion) focuses on creating more maintainable, flexible, and understandable software systems.
- Keep It Simple, Stupid (KISS): Emphasizes simplicity in design and development, advocating for straightforward solutions over complex ones.
- Don't Repeat Yourself (DRY): Encourages the elimination of redundant code to enhance maintainability by reusing existing code.
- You Aren't Gonna Need It (YAGNI): Advises against adding functionality or code until it is necessary, avoiding unnecessary complexities.
Among the pantheon of widely recognized design principles, the SOLID principles stand as a bedrock, renowned for their effectiveness in shaping resilient and scalable software architectures.
SOLID design principles
The SOLID principles, a fundamental set of design principles in software engineering, encapsulate a structured approach to crafting robust, maintainable, and adaptable codebases. Coined by Robert C. Martin, these principles serve as foundational pillars guiding developers toward writing flexible, scalable, and comprehensible software.
Here is a concise summary of each principle's role:
- Single Responsibility Principle (SRP): Encourages each class or module to have a single responsibility, ensuring clarity and maintainability.
- Open/Closed Principle (OCP): Fosters code that is open for extension but closed for modification, enabling new functionalities without altering existing code.
- Liskov Substitution Principle (LSP): Ensures that objects of a superclass can be replaced by objects of its subclasses without affecting the program's functionality.
- Interface Segregation Principle (ISP): Promotes smaller, cohesive interfaces rather than large, all-encompassing ones, preventing clients from depending on interfaces they do not use.
- Dependency Inversion Principle (DIP): Encourages abstraction and decoupling by depending on abstractions rather than concrete implementations, facilitating flexibility and scalability.
SOLID design principles and their usage are explained in the the context of game development with great details in the book: Learning Game Architecture with Unity, which is available @ Amazon, Amazon India, BPB International, BPB India.