How to Implement Middleware in a Phalcon Application?
Middleware functions are an essential part of web development when it comes to managing requests and responses, enhancing security, and providing additional functionality like logging or authentication in our applications. In the Phalcon framework, implementing middleware may have slight nuances compared to other PHP frameworks, but it's equally powerful. In this tutorial, we'll dive into how to effectively implement middleware in a Phalcon application. Understanding Middleware in Phalcon Middleware in Phalcon works by intercepting requests before they reach your application's controller. This mechanism allows developers to execute specific functions that can manipulate the request or terminate it, if necessary. It's invaluable in applications where you need to perform operations such as authentication, authorization, input validation, logging, and more. Steps to Implement Middleware in Phalcon Step 1: Setting Up a New Phalcon Project If you haven't set up a Phalcon project yet, consider using one of the following deployment blogs: Phalcon Framework Deployment Guide on Dreamhost Deploying Phalcon on Amazon Web Services Hostinger Phalcon Integration These resources will guide you through configurations and hosting setups to get your project running seamlessly on various platforms. Step 2: Creating a Middleware Phalcon lacks a formal middleware component as seen in frameworks like Laravel or Slim. However, you can create a middleware by utilizing the ‘Events’ and ‘Plugins’ system. Create a Custom Plugin Navigate to your project and create a Plugins directory, if it doesn't exist. mkdir app/Plugins Create a file named AuthMiddleware.php within this directory:
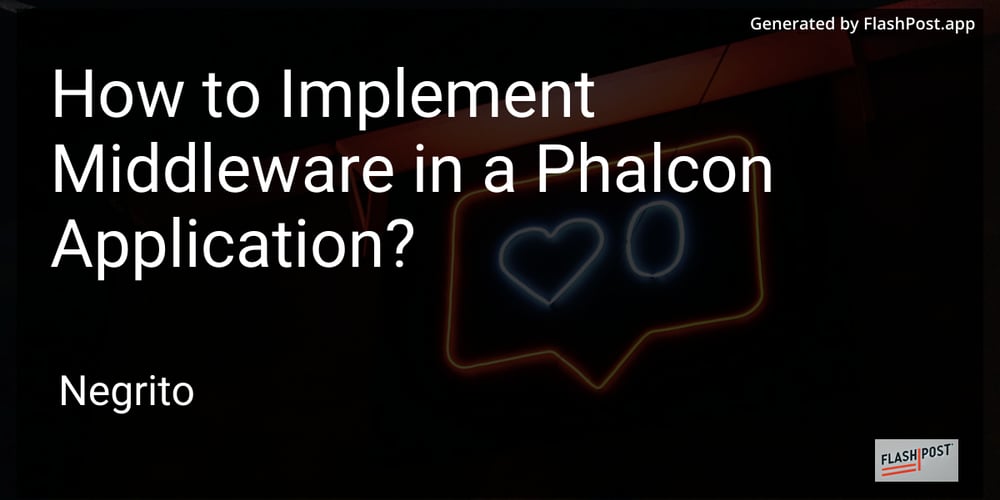
Middleware functions are an essential part of web development when it comes to managing requests and responses, enhancing security, and providing additional functionality like logging or authentication in our applications. In the Phalcon framework, implementing middleware may have slight nuances compared to other PHP frameworks, but it's equally powerful. In this tutorial, we'll dive into how to effectively implement middleware in a Phalcon application.
Understanding Middleware in Phalcon
Middleware in Phalcon works by intercepting requests before they reach your application's controller. This mechanism allows developers to execute specific functions that can manipulate the request or terminate it, if necessary. It's invaluable in applications where you need to perform operations such as authentication, authorization, input validation, logging, and more.
Steps to Implement Middleware in Phalcon
Step 1: Setting Up a New Phalcon Project
If you haven't set up a Phalcon project yet, consider using one of the following deployment blogs:
- Phalcon Framework Deployment Guide on Dreamhost
- Deploying Phalcon on Amazon Web Services
- Hostinger Phalcon Integration
These resources will guide you through configurations and hosting setups to get your project running seamlessly on various platforms.
Step 2: Creating a Middleware
Phalcon lacks a formal middleware component as seen in frameworks like Laravel or Slim. However, you can create a middleware by utilizing the ‘Events’ and ‘Plugins’ system.
Create a Custom Plugin
Navigate to your project and create a Plugins
directory, if it doesn't exist.
mkdir app/Plugins
Create a file named AuthMiddleware.php
within this directory:
namespace App\Plugins;
use Phalcon\Events\Event;
use Phalcon\Mvc\User\Plugin;
use Phalcon\Mvc\Dispatcher;
class AuthMiddleware extends Plugin
{
public function beforeExecuteRoute(Event $event, Dispatcher $dispatcher)
{
// Authenticate user logic
$authenticated = $this->session->get('auth') === true;
if (!$authenticated) {
$dispatcher->forward([
'controller' => 'index',
'action' => 'login',
]);
return false;
}
}
}
Step 3: Registering Your Middleware
To register your newly created middleware, modify the services.php
or the bootstrap file where the dispatcher is initialized.
$di->set(
'dispatcher',
function () use ($di) {
$eventsManager = $di->getShared('eventsManager');
// Attach the middleware plugin
$eventsManager->attach('dispatch:beforeExecuteRoute', new \App\Plugins\AuthMiddleware);
$dispatcher = new \Phalcon\Mvc\Dispatcher();
$dispatcher->setEventsManager($eventsManager);
return $dispatcher;
}
);
Step 4: Testing Your Middleware
Test your middleware by attempting to access a protected route. If the middleware is functioning correctly, unauthenticated users should be redirected to the login page or wherever you've defined.
Conclusion
Implementing middleware in a Phalcon application can significantly enhance your application's capability to handle requests securely and efficiently. Through the use of Events and Plugins, developers can create flexible and reusable middleware components to perform a variety of tasks before a route is executed.
By following these steps and with resources available for deploying Phalcon on different hosting services, you can extend your Phalcon projects efficiently and securely.
For more information on deploying Phalcon, visit:
This article follows SEO best practices, including the use of relevant keywords like "Phalcon middleware," and strategically uses links to strengthen the authority and context regarding deployment environments.