Understanding Node.js Streams — The Unsung Hero of I/O
When working with Node.js, you’ve probably dealt with reading files, sending HTTP responses, or handling data from APIs. But have you ever wondered how Node handles large data efficiently? Welcome to the world of Streams. What Are Streams in Node.js? Streams are a powerful way to handle large chunks of data piece by piece instead of loading it all into memory at once. They follow a simple idea: process data as it comes. Whether it's reading a file, sending a response, or piping video data — streams let you read, write, and transform data efficiently. There are four types of streams in Node.js: Readable – for reading data (e.g., fs.createReadStream) Writable – for writing data (e.g., fs.createWriteStream) Duplex – both readable and writable (e.g., a TCP socket) Transform – a special type of Duplex that modifies data as it passes through (e.g., gzip compression) Why Streams Matter Imagine you’re reading a 1GB log file. With fs.readFile(), you’re loading the entire file into memory. That’s risky. With streams: const fs = require('fs'); const stream = fs.createReadStream('bigfile.log', { encoding: 'utf8' }); stream.on('data', (chunk) => { console.log('Received chunk:', chunk.length); }); stream.on('end', () => { console.log('Done reading file.'); }); This reads the file in small chunks, using less memory and allowing other operations to continue — great for performance and scalability. Stream Piping One of the coolest things about streams is piping — connecting one stream to another: const fs = require('fs'); const readStream = fs.createReadStream('input.txt'); const writeStream = fs.createWriteStream('output.txt'); readStream.pipe(writeStream); Boom! You just copied a file using streams — memory efficient and elegant. Real-World Use Cases Reading/writing large files Streaming video/audio content Handling HTTP requests/responses Processing data in real-time (e.g., CSV parsing, compression) Gotchas and Tips Always handle stream errors: stream.on('error', handler) Backpressure can occur — use .pipe() to manage it automatically Prefer async/await with pipeline for better readability in modern apps const { pipeline } = require('stream/promises'); await pipeline( fs.createReadStream('input.txt'), fs.createWriteStream('output.txt') ); TL;DR Streams are the secret sauce behind Node.js's performance with I/O. They let you process data efficiently, save memory, and keep your app fast and responsive. Next time you’re reading files, dealing with APIs, or handling data flows — think streams. Have you used streams in your projects? Any cool patterns or struggles? Drop them in the comments!
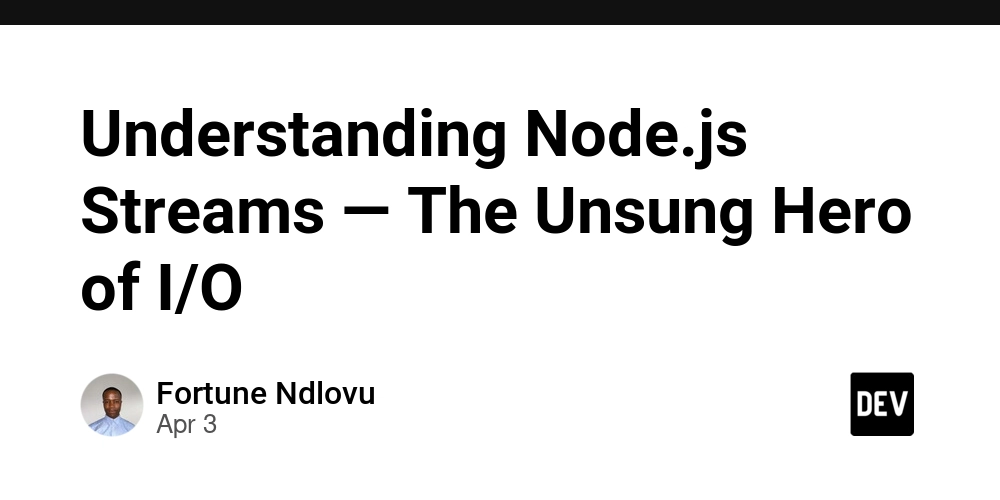
When working with Node.js, you’ve probably dealt with reading files, sending HTTP responses, or handling data from APIs. But have you ever wondered how Node handles large data efficiently?
Welcome to the world of Streams.
What Are Streams in Node.js?
Streams are a powerful way to handle large chunks of data piece by piece instead of loading it all into memory at once.
They follow a simple idea: process data as it comes. Whether it's reading a file, sending a response, or piping video data — streams let you read, write, and transform data efficiently.
There are four types of streams in Node.js:
-
Readable – for reading data (e.g.,
fs.createReadStream
) -
Writable – for writing data (e.g.,
fs.createWriteStream
) - Duplex – both readable and writable (e.g., a TCP socket)
- Transform – a special type of Duplex that modifies data as it passes through (e.g., gzip compression)
Why Streams Matter
Imagine you’re reading a 1GB log file. With fs.readFile()
, you’re loading the entire file into memory. That’s risky.
With streams:
const fs = require('fs');
const stream = fs.createReadStream('bigfile.log', { encoding: 'utf8' });
stream.on('data', (chunk) => {
console.log('Received chunk:', chunk.length);
});
stream.on('end', () => {
console.log('Done reading file.');
});
This reads the file in small chunks, using less memory and allowing other operations to continue — great for performance and scalability.
Stream Piping
One of the coolest things about streams is piping — connecting one stream to another:
const fs = require('fs');
const readStream = fs.createReadStream('input.txt');
const writeStream = fs.createWriteStream('output.txt');
readStream.pipe(writeStream);
Boom! You just copied a file using streams — memory efficient and elegant.
Real-World Use Cases
- Reading/writing large files
- Streaming video/audio content
- Handling HTTP requests/responses
- Processing data in real-time (e.g., CSV parsing, compression)
Gotchas and Tips
- Always handle stream errors:
stream.on('error', handler)
- Backpressure can occur — use
.pipe()
to manage it automatically - Prefer
async/await
withpipeline
for better readability in modern apps
const { pipeline } = require('stream/promises');
await pipeline(
fs.createReadStream('input.txt'),
fs.createWriteStream('output.txt')
);
TL;DR
Streams are the secret sauce behind Node.js's performance with I/O. They let you process data efficiently, save memory, and keep your app fast and responsive.
Next time you’re reading files, dealing with APIs, or handling data flows — think streams.
Have you used streams in your projects? Any cool patterns or struggles? Drop them in the comments!