Master Django REST Framework: Build a Student Management System API with Function-Based Views
Introduction Django REST Framework (DRF) is a powerful tool for building APIs with Django. In this tutorial, we will explore Function-Based Views (FBVs) by creating a Student Management System API. This CRUD-based API will allow you to perform essential operations such as retrieving, adding, updating, and deleting student records. Whether you're new to DRF or want to solidify your API development skills, this step-by-step guide will walk you through the entire process. What You’ll Learn Setting up Serializers Creating URLs and Function-Based Views Implementing CRUD operations (GET, POST, PUT, DELETE) Handling database interactions in DRF Video Tutorial Tutorial Breakdown 1. Serializers (00:00) Serializers in DRF convert complex data types such as Django models into JSON format. They are essential for sending and receiving API responses. In this section, we define a serializer for our Student model. 2. URLs and Views Setup (03:37) We define API endpoints using Django’s urls.py and connect them to corresponding function-based views. 3. GET - Retrieve Students from Database (08:17) This endpoint retrieves all student records from the database using DRF’s Response and Django QuerySet. 4. POST - Add Data to the Database (13:00) We create a new student entry by handling HTTP POST requests, validating data, and saving it to the database. 5. GET - Retrieve One Student's Data (16:05) Fetching a single student’s details using an ID-based lookup ensures targeted retrieval of specific records. 6. PUT - Update One Student's Data (20:21) This section covers modifying existing student data using the HTTP PUT method. We validate input and update records accordingly. 7. DELETE - Remove Data from the Database (22:35) Handling HTTP DELETE requests allows us to remove student records safely from our system. Why Use Function-Based Views (FBVs)? FBVs offer simplicity and flexibility, making them a great choice for small-to-medium-sized projects. While Django also supports Class-Based Views (CBVs) and ViewSets, FBVs provide: Better readability for beginners Direct control over request handling Lightweight implementations without added complexity Conclusion By following this tutorial, you now have a fully functional Student Management System API using Function-Based Views (FBVs) in Django REST Framework. You’ve learned how to create API endpoints for CRUD operations, manage serialization, and handle database interactions.
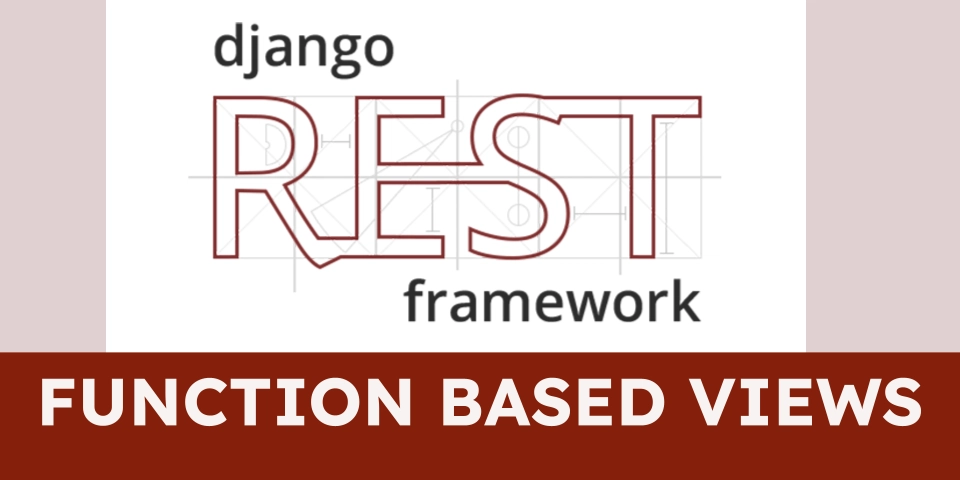
Introduction
Django REST Framework (DRF) is a powerful tool for building APIs with Django. In this tutorial, we will explore Function-Based Views (FBVs) by creating a Student Management System API. This CRUD-based API will allow you to perform essential operations such as retrieving, adding, updating, and deleting student records.
Whether you're new to DRF or want to solidify your API development skills, this step-by-step guide will walk you through the entire process.
What You’ll Learn
- Setting up Serializers
- Creating URLs and Function-Based Views
- Implementing CRUD operations (GET, POST, PUT, DELETE)
- Handling database interactions in DRF
Video Tutorial
Tutorial Breakdown
1. Serializers (00:00)
Serializers in DRF convert complex data types such as Django models into JSON format. They are essential for sending and receiving API responses. In this section, we define a serializer for our Student model.
2. URLs and Views Setup (03:37)
We define API endpoints using Django’s urls.py
and connect them to corresponding function-based views.
3. GET - Retrieve Students from Database (08:17)
This endpoint retrieves all student records from the database using DRF’s Response
and Django QuerySet.
4. POST - Add Data to the Database (13:00)
We create a new student entry by handling HTTP POST requests, validating data, and saving it to the database.
5. GET - Retrieve One Student's Data (16:05)
Fetching a single student’s details using an ID-based lookup ensures targeted retrieval of specific records.
6. PUT - Update One Student's Data (20:21)
This section covers modifying existing student data using the HTTP PUT method. We validate input and update records accordingly.
7. DELETE - Remove Data from the Database (22:35)
Handling HTTP DELETE requests allows us to remove student records safely from our system.
Why Use Function-Based Views (FBVs)?
FBVs offer simplicity and flexibility, making them a great choice for small-to-medium-sized projects. While Django also supports Class-Based Views (CBVs) and ViewSets, FBVs provide:
- Better readability for beginners
- Direct control over request handling
- Lightweight implementations without added complexity
Conclusion
By following this tutorial, you now have a fully functional Student Management System API using Function-Based Views (FBVs) in Django REST Framework. You’ve learned how to create API endpoints for CRUD operations, manage serialization, and handle database interactions.