Mastering JavaScript Functions: Declarations, Expressions, and Arrow Functions
Introduction: In this article, we can master the Functions in JavaScript, including how they work internally, how to write different function types and more! So, let’s start with what is a Function exactly. In simple terms, we can say that a “Function is a block of code designed to perform a particular task“ Why do we use Functions? Using functions allows us to reuse code effectively, avoiding the need to duplicate it repeatedly. We can write code that can be used many times We can use the same code with different arguments, to produce different results Syntax: Let’s understand the Function syntax, function name(parameter 1, parameter 2){ // code to be executed } In JavaScript, a Function is defined with the function keyword, followed by a name, and followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). You understood the syntax of the function and now we can write an example of how a function works // Example 1 function myName(){ console.log("My name is Pavan Varma"); } myName(); // this will print - My name is Pavan Varma Now, we write an example of a function with parameters: // Example 2 function fruitJuice(apples, oranges){ console.log(apples, oranges); console.log(`Juice with ${apples} apples and ${oranges} oranges`); } fruitJuice(2, 3); // it prints // 2, 3 // Juice with 2 apples and 3 oranges Different Function Types: There are three different writing functions, but they all work similarly: receive input data, transform data, and then output data. 1) Function Declaration: A function that can be used before it’s declared // function declaration example function(birthYear){ return 2025 - birthYear; } 2) Function Expression: Function value that stored in a variable // function expression example const calcAge = function(birthYear){ return 2025 - birthYear; } 3) Arrow Function: Arrow Functions are great for quick one-line functions. It has no ‘this’ keyword. // Arrow function example const calcAge = birthYear => 2025 - birthYear; Parameters and Arguments in Functions In functions, parameters are placeholders to receive input values like local variables of a function Arguments are actual values of parameters to input data Sometimes, we can assign default values to parameters. If no argument is passed, the default value will act as the argument. Default parameters are introduced in ES6. // parameters function greet(parameter1 = 'Hey', parameter2){ // in parameter1 we passed a value which acts as the default parameter // body } greet('hi', 'hello'); // hi and hello are the arguments Return Statement: Whenever JavaScript reaches the return then the functions stop executing and terminate. Then, the caller will receive the return value. // return example // sum of two numbers function sum(a, b){ return a + b; } let a = 10; let b = 20; const sumOfTwo = sum(a, b); // return value stores here console.log(sumOfTwo); // prints the result Function Scope: Scope refers to the accessibility and visibility of variables within different parts of a program. It determines where variables can be used and how they are accessed in the code. In Function Scope, variables are accessible only inside the function, NOT outside. // global scope let language = 'JavaScript'; // function scope example console.log(firstName); // it throws an error because the firstName is only available in myName fn function myName(){ let firstName = 'Pavan'; let lastName = 'Varma'; console.log(`${firstName}${lastName} likes ${language}`); //It works here, because the language variable is a global variable // Pavan Varma likes JavaScript. } console.log(lastName); // throws error as well..! Function Review: Anatomy of a function: Callback Functions: On a simple level, Calling one function inside another is called a Callback Function. // callback function example function calculator(a, b, fnToCall){ //(2, 5, sum) const ans = fnToCall(a, b); // goes to sum(2, 5) fn return ans; // returns to caller } function sum(a, b){ // sum(2, 5) return a + b; // 7 returns to calculator fn } function sub(a, b){ return a - b; } function product(a, b) { return a * b; } const value = calculator(2, 5, sum); // Here the callback function is applied and will receive the return value console.log(value); // prints the answer Now we see some functions that act as callback functions, setTimeout(): It is a function, that is supposed to call a function after a certain duration. // example function greet() { console.log("Hello World!"); } setTimeout(greet, 2 * 1000); // Now the greet fn will run after 2 seconds. setInterval(): It is a function, that ru
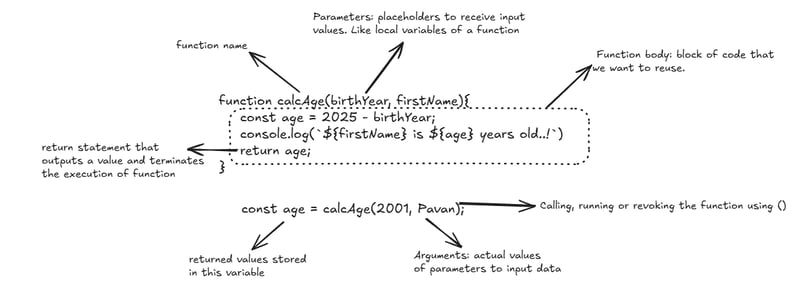
Introduction:
In this article, we can master the Functions in JavaScript, including how they work internally, how to write different function types and more!
So, let’s start with what is a Function exactly.
In simple terms, we can say that a “Function is a block of code designed to perform a particular task“
Why do we use Functions?
Using functions allows us to reuse code effectively, avoiding the
need to duplicate it repeatedly.We can write code that can be used many times
We can use the same code with different arguments, to produce
different results
Syntax:
Let’s understand the Function syntax,
function name(parameter 1, parameter 2){
// code to be executed
}
In JavaScript, a Function is defined with the function keyword, followed by a name, and followed by parentheses ().
Function names can contain letters, digits, underscores, and dollar signs (same rules as variables).
You understood the syntax of the function and now we can write an example of how a function works
// Example 1
function myName(){
console.log("My name is Pavan Varma");
}
myName(); // this will print - My name is Pavan Varma
Now, we write an example of a function with parameters:
// Example 2
function fruitJuice(apples, oranges){
console.log(apples, oranges);
console.log(`Juice with ${apples} apples and ${oranges} oranges`);
}
fruitJuice(2, 3);
// it prints
// 2, 3
// Juice with 2 apples and 3 oranges
Different Function Types:
There are three different writing functions, but they all work similarly: receive input data, transform data, and then output data.
1) Function Declaration:
A function that can be used before it’s declared
// function declaration example
function(birthYear){
return 2025 - birthYear;
}
2) Function Expression:
Function value that stored in a variable
// function expression example
const calcAge = function(birthYear){
return 2025 - birthYear;
}
3) Arrow Function:
Arrow Functions are great for quick one-line functions. It has no ‘this’ keyword.
// Arrow function example
const calcAge = birthYear => 2025 - birthYear;
Parameters and Arguments in Functions
In functions, parameters are placeholders to receive input values
like local variables of a functionArguments are actual values of parameters to input data
Sometimes, we can assign default values to parameters. If no argument
is passed, the default value will act as the argument.
Default parameters are introduced in ES6.
// parameters
function greet(parameter1 = 'Hey', parameter2){
// in parameter1 we passed a value which acts as the default parameter
// body
}
greet('hi', 'hello'); // hi and hello are the arguments
Return Statement:
Whenever JavaScript reaches the return then the functions stop executing and terminate.
Then, the caller will receive the return value.
// return example
// sum of two numbers
function sum(a, b){
return a + b;
}
let a = 10;
let b = 20;
const sumOfTwo = sum(a, b); // return value stores here
console.log(sumOfTwo); // prints the result
Function Scope:
Scope refers to the accessibility and visibility of variables within different parts of a program. It determines where variables can be used and how they are accessed in the code.
In Function Scope, variables are accessible only inside the function, NOT outside.
// global scope
let language = 'JavaScript';
// function scope example
console.log(firstName); // it throws an error because the firstName is only available in myName fn
function myName(){
let firstName = 'Pavan';
let lastName = 'Varma';
console.log(`${firstName}${lastName} likes ${language}`);
//It works here, because the language variable is a global variable
// Pavan Varma likes JavaScript.
}
console.log(lastName); // throws error as well..!
Function Review: Anatomy of a function:
Callback Functions:
On a simple level, Calling one function inside another is called a Callback Function.
// callback function example
function calculator(a, b, fnToCall){ //(2, 5, sum)
const ans = fnToCall(a, b); // goes to sum(2, 5) fn
return ans; // returns to caller
}
function sum(a, b){ // sum(2, 5)
return a + b; // 7 returns to calculator fn
}
function sub(a, b){
return a - b;
}
function product(a, b) {
return a * b;
}
const value = calculator(2, 5, sum);
// Here the callback function is applied and will receive the return value
console.log(value); // prints the answer
Now we see some functions that act as callback functions,
setTimeout():
It is a function, that is supposed to call a function after a certain duration.
// example
function greet() {
console.log("Hello World!");
}
setTimeout(greet, 2 * 1000); // Now the greet fn will run after 2 seconds.
setInterval():
It is a function, that runs after runs on some interval which is given by the user.
// example
function greet() {
console.log("Hello World!");
}
setInterval(greet, 3 * 1000); // Now the greet fn will run again and again for every 3 seconds.
Higher-Order Functions:
A function that receives another function as an argument, that returns a new function, or both. This is only possible because of first-class functions.
The function that receives another function:
//We have already seen this example in the callback function that will receive fn as an argument
function greet() {
console.log("Hey Pavan");
}
btnClose.addEventListener('click', greet);
// Here addEventListener is a function and that receives greet as an argument which is a callback fn
The function that returns a new function:
// example
const greet = function(greeting){
// Here the fn returning a new fn.
return function(name){
// The greeting parameter is accessible in this return fn.
console.log(`${greeting} ${name}`);
}
}
// Firstly, the greet fn will called and returns the new fn and now
// greetHello will act as new return fn
const greetHello = greet("Hello");
greetHello("Pavan"); // prints - Hello Pavan
Closures:
A closure is the closed-over variable environment of the execution
context in which a function was created, even after that execution
context is gone.A closure gives a function access to all the variables of its parent
function, even after that parent function has returned. The function
keeps a reference to its outer scope, which preserves the scope chain
throughout time.A closure is like a backpack that a function carries around wherever
it goes. This backpack has all the variables that were present in the
environment where the function was created.We do NOT have to manually create a closure, this is a JavaScript
feature that happens automatically.We can’t even access closed-over variables explicitly.
// Example 1
const a = function(){
const x = 23;
y = function (){
console.log(x * 2);
}
}
a(); // it executes upto y fn
y(); // now y fn has access to x variable even after a fn stopped executing
// prints 23 * 2 = 46
// Example 2
const secureBooking = function () {
let passengerCount = 0;
return function () {
passengerCount++;
console.log(
`${passengerCount} ${passengerCount > 1 ? 'passengers' : 'passenger'}`
);
};
};
const booker = secureBooking();
booker(); // 1
booker(); // 2
booker(); // 3
IIFE (Immediately Invoked Function Expressions):
An IIFE (Immediately Invoked Function Expression) is a JavaScript function that runs as soon as it is defined. It is a function that is executed immediately after its declaration, without needing to be called separately.
If we ever want to run a function just once then we use IIFE.
// Example
const runOnce = function(){
console.log("This will never run again");
}
runOnce(); // this will run for one time
// but if we again call it, then it runs one more time
// IIFE
(function (){
console.log("This will never run again");
})(); // This will only run once and then terminated..!
Summary:
So, we covered almost every topic on Functions and if I missed anything I will write a new article for you to learn it.
We covered what is Functions, Syntax, Different types of functions, Parameters and Arguments, Return statements, Function Scope, Callbacks, Higher-order functions, Closures, and IIFE.
Hope you’ve learned something from this article, if you find any mistakes in the article please mention them in the comments I will fix them..!
It’s been a long time since my last article but from now on I will keep posting a new article every week so you learn something, for me also it will be good practice to post something that I’ve learned
Thanks for Reading this article :)
I started reading Atomic Habits recently so I’m adding one line from the book that inspires you
“ The most effective way to change your habits is to focus not on what you want to achieve, but on who you wish to become ”