Next.js 15 Scroll Behavior: A Comprehensive Guide
Next.js 15 introduced significant improvements to navigation and scroll behavior control, giving developers more fine-grained options for creating smooth user experiences. This article dives deep into these features, providing practical examples for both beginners and advanced developers. Understanding Scroll Behavior in Next.js Historically, web frameworks have defaulted to scrolling to the top of the page when navigating between routes. While this behavior makes sense in traditional multi-page applications, modern web apps often require more nuanced control over scroll position. Next.js 15 addresses this with the new scroll property, which gives developers explicit control over scroll restoration during navigation. Basic Usage: The scroll Prop With the Link Component The most straightforward way to control scroll behavior is through the Link component: import Link from 'next/link' // Default behavior: scrolls to top View Products // Prevents scrolling to top View Products When scroll={false} is specified, Next.js will maintain the user's scroll position after navigation, creating a seamless experience. With Programmatic Navigation For programmatic navigation, the scroll option is available through the router: import { useRouter } from 'next/navigation' function FilterProducts() { const router = useRouter() const applyFilter = (filter) => { // Maintain scroll position when applying filters router.push(`/products?category=${filter}`, { scroll: false }) } return ( applyFilter('electronics')}> Filter by Electronics ) } Advanced Use Cases Implementing Infinite Scroll The scroll={false} option shines when implementing infinite scroll patterns: import { useEffect, useState } from 'react' import { useRouter } from 'next/navigation' function InfiniteProductList() { const [page, setPage] = useState(1) const [products, setProducts] = useState([]) const router = useRouter() const loadMore = async () => { const newPage = page + 1 // Update URL to reflect new page without scrolling router.push(`/products?page=${newPage}`, { scroll: false }) // Fetch more products const newProducts = await fetchProducts(newPage) setProducts([...products, ...newProducts]) setPage(newPage) } // Intersection Observer to detect when user reaches bottom useEffect(() => { // Implementation details... }, []) return ( {products.map(product => ( ))} Loading more... ) } Multi-step Forms For multi-step forms where maintaining context is crucial: function CheckoutProcess() { const router = useRouter() const [formData, setFormData] = useState({}) const goToNextStep = (step) => { // Save current form data localStorage.setItem('checkout-data', JSON.stringify(formData)) // Navigate to next step without scrolling to top router.push(`/checkout/step-${step}`, { scroll: false }) } return ( goToNextStep(2)}> {/* Form fields */} Continue to Shipping ) } Scroll to Specific Elements While scroll={false} prevents automatic scrolling to the top, you might want to scroll to specific elements after navigation. You can combine the scroll property with the useEffect hook: import { useEffect, useRef } from 'react' import { useSearchParams } from 'next/navigation' function ProductPage() { const reviewsRef = useRef(null) const searchParams = useSearchParams() useEffect(() => { // Check if we should scroll to reviews section if (searchParams.get('section') === 'reviews' && reviewsRef.current) { reviewsRef.current.scrollIntoView({ behavior: 'smooth', block: 'start' }) } }, [searchParams]) return ( Customer Reviews {/* Reviews content */} ) } Performance Implications The scroll behavior API is implemented efficiently in Next.js 15, but there are some considerations: Memory Usage: When using scroll={false}, Next.js needs to maintain state information about the scroll position, which consumes a small amount of memory. Initial Page Load: The scroll prop only affects navigation between routes within your application, not the initial page load. Nested Layouts: In the App Router, the behavior applies to the changed segments of the route, respecting the nested layout structure. Best Practices When to Use scroll={false} Filtering or sorting operations where maintaining context is important Tabbed interfaces where the content changes but the layout remains the same Infinite scroll implementations Multi-step forms or wizards When to Keep Default Scroll Behavior Major route changes where new content should be viewed from the top When transitioning between fundamentally different sections of your application For ac
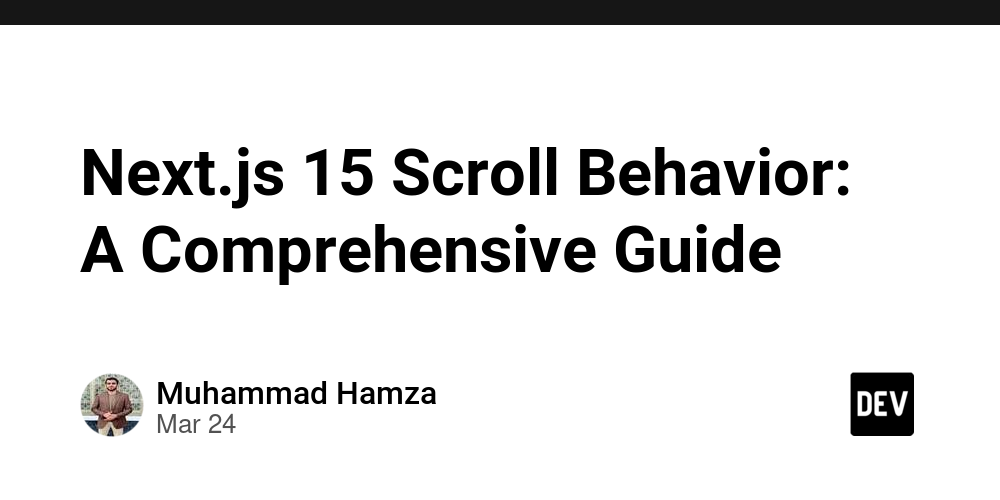
Next.js 15 introduced significant improvements to navigation and scroll behavior control, giving developers more fine-grained options for creating smooth user experiences. This article dives deep into these features, providing practical examples for both beginners and advanced developers.
Understanding Scroll Behavior in Next.js
Historically, web frameworks have defaulted to scrolling to the top of the page when navigating between routes. While this behavior makes sense in traditional multi-page applications, modern web apps often require more nuanced control over scroll position.
Next.js 15 addresses this with the new scroll
property, which gives developers explicit control over scroll restoration during navigation.
Basic Usage: The scroll
Prop
With the Link Component
The most straightforward way to control scroll behavior is through the Link
component:
import Link from 'next/link'
// Default behavior: scrolls to top
<Link href="/products">View Products</Link>
// Prevents scrolling to top
<Link href="/products" scroll={false}>View Products</Link>
When scroll={false}
is specified, Next.js will maintain the user's scroll position after navigation, creating a seamless experience.
With Programmatic Navigation
For programmatic navigation, the scroll
option is available through the router:
import { useRouter } from 'next/navigation'
function FilterProducts() {
const router = useRouter()
const applyFilter = (filter) => {
// Maintain scroll position when applying filters
router.push(`/products?category=${filter}`, { scroll: false })
}
return (
<button onClick={() => applyFilter('electronics')}>
Filter by Electronics
button>
)
}
Advanced Use Cases
Implementing Infinite Scroll
The scroll={false}
option shines when implementing infinite scroll patterns:
import { useEffect, useState } from 'react'
import { useRouter } from 'next/navigation'
function InfiniteProductList() {
const [page, setPage] = useState(1)
const [products, setProducts] = useState([])
const router = useRouter()
const loadMore = async () => {
const newPage = page + 1
// Update URL to reflect new page without scrolling
router.push(`/products?page=${newPage}`, { scroll: false })
// Fetch more products
const newProducts = await fetchProducts(newPage)
setProducts([...products, ...newProducts])
setPage(newPage)
}
// Intersection Observer to detect when user reaches bottom
useEffect(() => {
// Implementation details...
}, [])
return (
<div>
{products.map(product => (
<ProductCard key={product.id} product={product} />
))}
<div ref={observerRef}>Loading more...div>
div>
)
}
Multi-step Forms
For multi-step forms where maintaining context is crucial:
function CheckoutProcess() {
const router = useRouter()
const [formData, setFormData] = useState({})
const goToNextStep = (step) => {
// Save current form data
localStorage.setItem('checkout-data', JSON.stringify(formData))
// Navigate to next step without scrolling to top
router.push(`/checkout/step-${step}`, { scroll: false })
}
return (
<form onSubmit={() => goToNextStep(2)}>
{/* Form fields */}
<button type="submit">Continue to Shippingbutton>
form>
)
}
Scroll to Specific Elements
While scroll={false}
prevents automatic scrolling to the top, you might want to scroll to specific elements after navigation. You can combine the scroll property with the useEffect
hook:
import { useEffect, useRef } from 'react'
import { useSearchParams } from 'next/navigation'
function ProductPage() {
const reviewsRef = useRef(null)
const searchParams = useSearchParams()
useEffect(() => {
// Check if we should scroll to reviews section
if (searchParams.get('section') === 'reviews' && reviewsRef.current) {
reviewsRef.current.scrollIntoView({
behavior: 'smooth',
block: 'start'
})
}
}, [searchParams])
return (
<div>
<ProductDetails />
<div ref={reviewsRef} id="reviews">
<h2>Customer Reviewsh2>
{/* Reviews content */}
div>
div>
)
}
Performance Implications
The scroll behavior API is implemented efficiently in Next.js 15, but there are some considerations:
Memory Usage: When using
scroll={false}
, Next.js needs to maintain state information about the scroll position, which consumes a small amount of memory.Initial Page Load: The
scroll
prop only affects navigation between routes within your application, not the initial page load.Nested Layouts: In the App Router, the behavior applies to the changed segments of the route, respecting the nested layout structure.
Best Practices
When to Use scroll={false}
- Filtering or sorting operations where maintaining context is important
- Tabbed interfaces where the content changes but the layout remains the same
- Infinite scroll implementations
- Multi-step forms or wizards
When to Keep Default Scroll Behavior
- Major route changes where new content should be viewed from the top
- When transitioning between fundamentally different sections of your application
- For accessibility reasons, when users would expect to start at the top
Browser Compatibility
Next.js scroll behavior control works across all modern browsers. The implementation uses the History API and falls back gracefully in older browsers.
Custom Scroll Restoration
For more complex scenarios, you might want to implement custom scroll restoration logic:
'use client'
import { useEffect } from 'react'
import { usePathname, useSearchParams } from 'next/navigation'
export function ScrollRestorationManager() {
const pathname = usePathname()
const searchParams = useSearchParams()
useEffect(() => {
// Save current scroll position for this route
const saveScrollPosition = () => {
const positions = JSON.parse(sessionStorage.getItem('scrollPositions') || '{}')
positions[pathname + searchParams.toString()] = window.scrollY
sessionStorage.setItem('scrollPositions', JSON.stringify(positions))
}
// Restore scroll position if available
const restoreScrollPosition = () => {
const positions = JSON.parse(sessionStorage.getItem('scrollPositions') || '{}')
const savedPosition = positions[pathname + searchParams.toString()]
if (savedPosition !== undefined) {
window.scrollTo(0, savedPosition)
}
}
// Add event listeners
window.addEventListener('beforeunload', saveScrollPosition)
restoreScrollPosition()
return () => {
window.removeEventListener('beforeunload', saveScrollPosition)
}
}, [pathname, searchParams])
return null
}
This component can be included in your layout to provide custom scroll restoration across your entire application.
Integration with Other Next.js Features
With Server Components
Remember that the scroll
prop functionality requires client-side JavaScript. When using Server Components, you'll need to create a Client Component wrapper to handle scroll behavior:
// ServerComponent.jsx
export default function ProductList({ products }) {
return (
<div>
{products.map(product => (
<ProductItem key={product.id} product={product} />
))}
div>
)
}
// ClientWrapper.jsx
'use client'
import { useRouter } from 'next/navigation'
import ProductList from './ServerComponent'
export default function ProductListWrapper({ products }) {
const router = useRouter()
const handleProductFilter = (category) => {
router.push(`/products?category=${category}`, { scroll: false })
}
return (
<>
<div className="filters">
<button onClick={() => handleProductFilter('electronics')}>Electronicsbutton>
<button onClick={() => handleProductFilter('clothing')}>Clothingbutton>
div>
<ProductList products={products} />
>
)
}
With Suspense and Loading States
The scroll behavior works well with Suspense boundaries, maintaining the scroll position even when content is still loading:
import { Suspense } from 'react'
import LoadingSpinner from '@/components/LoadingSpinner'
import ProductList from '@/components/ProductList'
export default function ProductsPage() {
return (
<div>
<h1>Productsh1>
<Suspense fallback={<LoadingSpinner />}>
<ProductList />
Suspense>
div>
)
}
Conclusion
Next.js 15's scroll behavior control options provide a powerful way to create more intuitive navigation experiences. By leveraging the scroll
prop in both the Link
component and programmatic navigation, developers can craft smooth, context-preserving interactions that maintain user position exactly when needed.
Whether you're building an e-commerce site with infinite product loading, a multi-step form, or just want to improve the feel of your application, these tools offer the right level of control without requiring complex custom implementations.
As web applications continue to evolve toward more app-like experiences, features like scroll control become increasingly important for maintaining user context and creating seamless transitions between different states of your application.