Why not strings in C
As we all know, C is a low-level programming language used by CHADS (don’t get offended) who prefer building operating systems, software, or game engines. Recently, when I was learning C, I found some things that might confuse beginners—especially those coming from non-statically typed languages like Miniscript or Python, or those picking C as their first language. If you picked C as your first programming language, I salute you—but sorry, there will be no one at your funeral other than me to salute. In short, picking C as a first language when your brain struggles with simple math is just not the right option. I suggest first using Go (Golang) and then moving to C. If you somehow stick with C and don’t turn back, you are a true C lover and should definitely give C++ and Rust a try. Now, let’s clear up the doubt mentioned in the title. Why Doesn’t C Have Strings? If you are learning C, you might have already noticed that there are no built-in strings—unlike modern programming languages where string handling is standard. Even Rust and Golang have dedicated string types. So why not C? Simplicity and Efficiency One may argue: How is this simple? It actually makes things more complex! Declaring a string is way easier than declaring an array of characters to handle a string. And you’d be right. Manually handling strings in C is harder than using built-in string types in other languages. But the decision to not include a built-in string type in C was intentional. C was designed for maximum performance and minimal overhead, giving developers full control over memory management. In C, a string is just an array of characters ending with a null terminator (\0). This makes string handling efficient but requires the programmer to manage memory manually. For example: #include int main() { char myString[] = "Hello, World!"; // Implicit null terminator printf("%s\n", myString); return 0; } This is simple, but if you need dynamic strings, you have to allocate memory manually: #include #include #include int main() { char *myStr = (char *)malloc(20 * sizeof(char)); // Allocating memory strcpy(myStr, "Hello"); printf("%s\n", myStr); free(myStr); // Free memory to avoid leaks return 0; } This is more complex than simply writing std::string in C++ or String in Rust, but it provides full control over how memory is used and allocated. Why Did C Avoid Built-in Strings? Performance: Built-in string handling often requires extra memory and processing. Low-Level Control: C is designed to give developers direct access to memory. Minimalism: C was created to be a simple, efficient language without unnecessary abstractions. Conclusion C doesn’t have strings because it wasn’t designed to hold your hand—it’s a language for people who like to control every byte of memory. If you want an easier experience, languages like C++, Rust, or Golang provide better string management. But if you master C’s manual string handling, you will surely gain a deeper understanding of memory management, which is essential for systems programming , game engine programming and operating system programming.
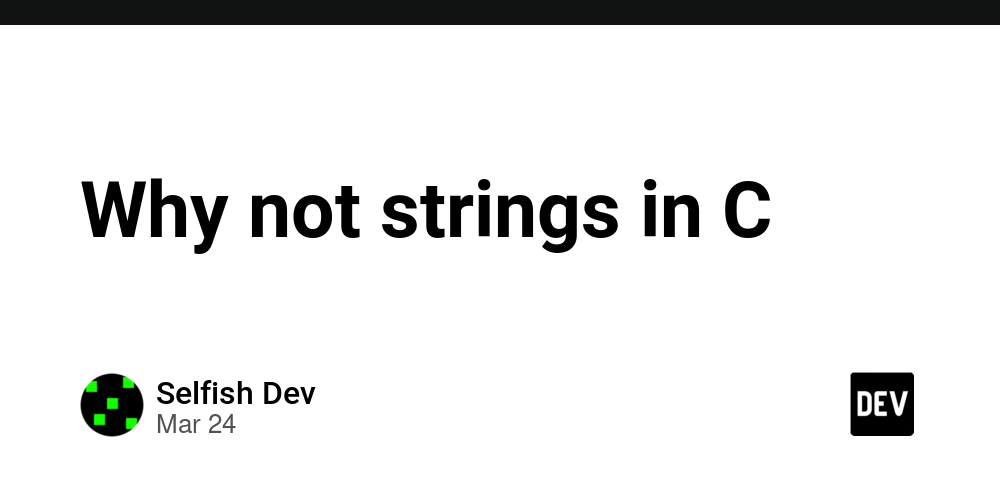
As we all know, C is a low-level programming language used by CHADS (don’t get offended) who prefer building operating systems, software, or game engines.
Recently, when I was learning C, I found some things that might confuse beginners—especially those coming from non-statically typed languages like Miniscript or Python, or those picking C as their first language.
If you picked C as your first programming language, I salute you—but sorry, there will be no one at your funeral other than me to salute. In short, picking C as a first language when your brain struggles with simple math is just not the right option. I suggest first using Go (Golang) and then moving to C. If you somehow stick with C and don’t turn back, you are a true C lover and should definitely give C++ and Rust a try.
Now, let’s clear up the doubt mentioned in the title.
Why Doesn’t C Have Strings?
If you are learning C, you might have already noticed that there are no built-in strings—unlike modern programming languages where string handling is standard. Even Rust and Golang have dedicated string types. So why not C?
Simplicity and Efficiency
One may argue: How is this simple? It actually makes things more complex! Declaring a string is way easier than declaring an array of characters to handle a string.
And you’d be right. Manually handling strings in C is harder than using built-in string types in other languages. But the decision to not include a built-in string type in C was intentional. C was designed for maximum performance and minimal overhead, giving developers full control over memory management.
In C, a string is just an array of characters ending with a null terminator (\0
). This makes string handling efficient but requires the programmer to manage memory manually.
For example:
#include
int main() {
char myString[] = "Hello, World!"; // Implicit null terminator
printf("%s\n", myString);
return 0;
}
This is simple, but if you need dynamic strings, you have to allocate memory manually:
#include
#include
#include
int main() {
char *myStr = (char *)malloc(20 * sizeof(char)); // Allocating memory
strcpy(myStr, "Hello");
printf("%s\n", myStr);
free(myStr); // Free memory to avoid leaks
return 0;
}
This is more complex than simply writing std::string
in C++ or String
in Rust, but it provides full control over how memory is used and allocated.
Why Did C Avoid Built-in Strings?
- Performance: Built-in string handling often requires extra memory and processing.
- Low-Level Control: C is designed to give developers direct access to memory.
- Minimalism: C was created to be a simple, efficient language without unnecessary abstractions.
Conclusion
C doesn’t have strings because it wasn’t designed to hold your hand—it’s a language for people who like to control every byte of memory. If you want an easier experience, languages like C++, Rust, or Golang provide better string management. But if you master C’s manual string handling, you will surely gain a deeper understanding of memory management, which is essential for systems programming , game engine programming and operating system programming.