Boost Your Software Quality with Assertion Testing Techniques
Introduction In coding land, it's really important to ensure that your app behaves as it should, isn't it? One of the ways people do this is by doing something called assertion testing. Assertion testing is about creating checkpoints in the form of assertions. They're small tests that verify everything's going as expected while the program's executing its task. If things don't go as planned and the test fails, an error appears, and that's great because it allows you to solve issues way earlier than they become huge. So sure, we're going to go deep into this entire assertion testing. We'll discuss why it matters, how you can use it in various coding languages, how it integrates into testing frameworks, and the most intelligent ways to approach it. What is an Assertion? An assertion is a statement employed to confirm that a particular condition is true at a particular moment in a program. Assertions assist in guaranteeing that the software acts as anticipated. When an assertion fails, it means that an unforeseen condition has been reached, usually resulting in an error or program termination. Assertion Meaning in Software Testing An assertion, in software testing, is a milestone that confirms whether the anticipated outcome equals the result. Assertions are used by test automation frameworks to ensure functions return values and systems perform as designed. Purpose of Assertions in Testing Verifies correctness – Ensures the actual output is correct. Catches defects early – Allows defects to be caught during unit and automation testing. Facilitates debugging – Gives instant feedback when a test fails. Enables automation – Employed within test scripts to ensure software functionality. Types of Assertions Boolean Assertions – Verifies whether a condition is true or false. Example: assertTrue(condition), assertFalse(condition) Equality Assertions – Verifies expected and actual values. Example: assertEqual(expected, actual), assertNotEqual(expected, actual) Null Assertions – Check whether an object is null or not. Example: assertIsNone(obj), assertIsNotNone(obj) Exception Assertions – Check that a function raises a specified exception. Example: assertRaises(Exception, function, *args) List/Collection Assertions – Check the presence or order of elements in a list. Example: assertIn(element, list), assertNotIn(element, list) What is Assertion Testing? Assertion testing is a technique employed to ensure that a program acts as it is supposed to. It entails inserting assertion statements within the code to test for given conditions. When an assertion fails, the program usually stops executing or throws an error, indicating something wrong in the code. The main objective of assertion testing is to detect bugs early and ensure software quality. It is especially helpful in unit testing, debugging, and automated test frameworks. Why is Assertion Testing Important? Early Bug Detection – Assertions assist developers in detecting problems before they become significant defects. Improved Code Quality – By imposing some conditions, assertions guarantee code integrity and strength. Improved Debugging – Assertion failures pinpoint where and why a program went astray. Effective Testing – Automated tests frequently use assertions to check results against anticipated outcomes. Improved Maintainability – Assertions are self-documenting code, simplifying the task of understanding and changing programs for developers. Assertion Testing in Various Programming Languages Most programming languages offer built-in assertion tools to allow for assertion testing. Some examples of the use of assertion testing in various languages are given below. ### Python The assert statement is provided in Python for assertion testing. When an assertion fails, a program raises an AssertionError. x = 10 y = 0 assert y != 0, "Division by zero is not allowed" result = x / y # This will raise an AssertionError if y is 0 Python also has support for assertion testing in unit test frameworks such as unittest and pytest. ### Java Java has an assert keyword which can be enabled or disabled at runtime. Failure of an assertion raises an AssertionError. public class AssertionTest { public static void main(String\[\] args) { int value = -1; assert value >= 0 : "Value should be non-negative"; System.out.println("Value: " + value); } } Assertions are commonly used in Java unit tests with frameworks like JUnit. ### C++ C++ provides an assertion mechanism through the header file. #include #include int main() { int age = -5; assert(age >= 0 && "Age cannot be negative"); std::cout
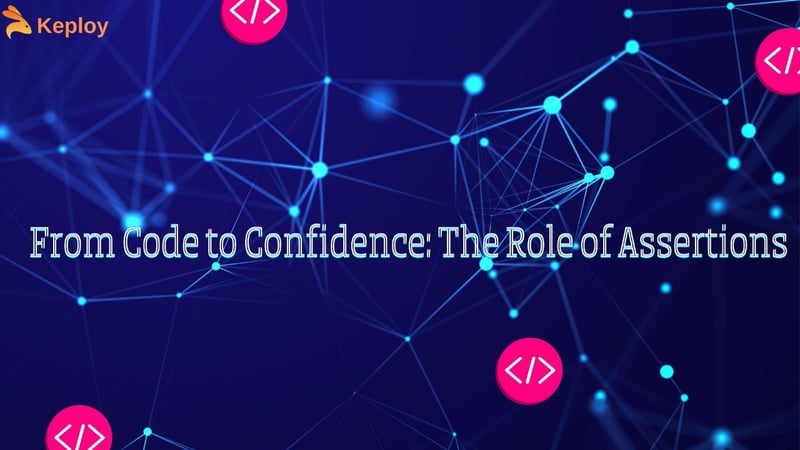
Introduction
In coding land, it's really important to ensure that your app behaves as it should, isn't it? One of the ways people do this is by doing something called assertion testing. Assertion testing is about creating checkpoints in the form of assertions. They're small tests that verify everything's going as expected while the program's executing its task. If things don't go as planned and the test fails, an error appears, and that's great because it allows you to solve issues way earlier than they become huge.
So sure, we're going to go deep into this entire assertion testing. We'll discuss why it matters, how you can use it in various coding languages, how it integrates into testing frameworks, and the most intelligent ways to approach it.
What is an Assertion?
An assertion is a statement employed to confirm that a particular condition is true at a particular moment in a program. Assertions assist in guaranteeing that the software acts as anticipated. When an assertion fails, it means that an unforeseen condition has been reached, usually resulting in an error or program termination.
Assertion Meaning in Software Testing
An assertion, in software testing, is a milestone that confirms whether the anticipated outcome equals the result. Assertions are used by test automation frameworks to ensure functions return values and systems perform as designed.
Purpose of Assertions in Testing
Verifies correctness – Ensures the actual output is correct.
Catches defects early – Allows defects to be caught during unit and automation testing.
Facilitates debugging – Gives instant feedback when a test fails.
Enables automation – Employed within test scripts to ensure software functionality.
Types of Assertions
- Boolean Assertions – Verifies whether a condition is true or false.
Example: assertTrue(condition), assertFalse(condition)
- Equality Assertions – Verifies expected and actual values.
Example: assertEqual(expected, actual), assertNotEqual(expected, actual)
- Null Assertions – Check whether an object is null or not.
Example: assertIsNone(obj), assertIsNotNone(obj)
- Exception Assertions – Check that a function raises a specified exception.
Example: assertRaises(Exception, function, *args)
- List/Collection Assertions – Check the presence or order of elements in a list.
Example: assertIn(element, list), assertNotIn(element, list)
What is Assertion Testing?
Assertion testing is a technique employed to ensure that a program acts as it is supposed to. It entails inserting assertion statements within the code to test for given conditions. When an assertion fails, the program usually stops executing or throws an error, indicating something wrong in the code.
The main objective of assertion testing is to detect bugs early and ensure software quality. It is especially helpful in unit testing, debugging, and automated test frameworks.
Why is Assertion Testing Important?
Early Bug Detection – Assertions assist developers in detecting problems before they become significant defects.
Improved Code Quality – By imposing some conditions, assertions guarantee code integrity and strength.
Improved Debugging – Assertion failures pinpoint where and why a program went astray.
Effective Testing – Automated tests frequently use assertions to check results against anticipated outcomes.
Improved Maintainability – Assertions are self-documenting code, simplifying the task of understanding and changing programs for developers.
Assertion Testing in Various Programming Languages
Most programming languages offer built-in assertion tools to allow for assertion testing. Some examples of the use of assertion testing in various languages are given below.
- ### Python
The assert statement is provided in Python for assertion testing. When an assertion fails, a program raises an AssertionError.
x = 10
y = 0
assert y != 0, "Division by zero is not allowed"
result = x / y # This will raise an AssertionError if y is 0
Python also has support for assertion testing in unit test frameworks such as unittest and pytest.
- ### Java
Java has an assert keyword which can be enabled or disabled at runtime. Failure of an assertion raises an AssertionError.
public class AssertionTest {
public static void main(String\[\] args) {
int value = -1;
assert value >= 0 : "Value should be non-negative";
System.out.println("Value: " + value);
}
}
Assertions are commonly used in Java unit tests with frameworks like JUnit.
- ### C++
C++ provides an assertion mechanism through the Assertion failures in C++ kill the program, which makes them good for debugging.
JavaScript lacks assertions but assertion testing is possible with test frameworks such as Mocha and Chai.
const assert = require('assert');
let result = 5 + 5;
assert.strictEqual(result, 10, "Addition result is incorrect");
Assertions are an essential part of software testing frameworks. Whether unit testing, integration testing, or UI testing, assertions are employed to ensure expected behavior.
Unit testing frameworks make extensive use of assertions to ensure individual functions or modules function properly.
Example: Python's unittest framework
import unittest
if name == "__main__":
Automated UI testing frameworks like Selenium use assertions to validate webpage elements.
from selenium import webdriver
browser = webdriver.Chrome()
browser.get("https://example.com")
assert "Example Domain" in browser.title, "Title does not match!"
browser.quit()
This assertion verifies that the title of the webpage is as expected.
Even with its advantages, assertion testing can be abused. Below are some common pitfalls:
Overusing Assertions – Too many assertions can increase execution time and make code messy. Using Assertions for Error Handling – Assertions should not be used in place of exception handling mechanisms. Disabling Assertions in Production – Some languages provide the ability to disable assertions at runtime, which can result in undetected errors. Ignoring Assertion Failures – Developers should examine and fix assertion failures rather than skipping them. To get the most from assertion testing, use these best practices:
Use Assertions for Critical Assumptions – Reserve assertions for conditions that should never occur in regular execution. Write Intelligible Error Messages – Supply informative messages to ease debugging. Juxtapose Assertions with Logging – Employ logging mechanisms to capture assertion failures for investigation. Test Assertions Periodically – Make certain that assertions still hold valid as code changes over time. Enable Assertions in Test Environments – Leave assertions on during testing but turn them off in production to improve performance. Testing assertions is super valuable when it comes to making sure software works right and is trustworthy. When devs get how to use these assertions well, they catch glitches way sooner, make their code better, and the stuff they build is solid. Whether we're talking about coding languages, the tools for testing software, or the automatic testing gear, testing assertions is key for checking if software makes the grade.
If you know how to put assertion testing into play, your software-making game gets a whole lot stronger and more reliable. Stick to the good stuff, steer clear of the traps, and devs can totally get the most out of testing assertions for crafting top-notch software goodies.
An assertion is a statement that verifies whether a condition is valid. It helps verify the expected outcome in test cases. When a statement fails, the test fails and reports an error. Assertions are used more and more in unit and automating testing. Assertions help make the software act as anticipated. They help to catch bugs early, improving software quality. Through automating the tests, they increase testing efficiency. They also help debug by showing failures. In case of a failing test, testing is terminated. An error message is printed, indicating what went wrong. This helps testers identify and fix issues conveniently. It ensures that faulty software is not missed. Typical examples include boolean, equality, and null checks. Boolean statements confirm true or false statements. Equality statements contrast expected and actual values. Exception assertions verify whether the error is thrown appropriately. #include
Assertion Testing in Software Testing Frameworks
Unit Testing using Assertion Testing
class TestMathOperations(unittest.TestCase):
def test\_addition(self):
self.assertEqual(2 + 3, 5)
def test\_division(self):
self.assertRaises(ZeroDivisionError, lambda: 10 / 0)
unittest.main()
Automated UI Testing with Assertions
Common Pitfalls in Assertion Testing
Best Practices for Assertion Testing
Conclusion
FAQ’s
1. What is an assertion in software testing?
2. Why are assertions helpful while testing?
3. What happens if an assertion fails in a test?
4. What are common types of assertions?