Error Handling in Go vs. C#: Trading Exceptions for Clarity
As a C# developer, you’re familiar with the convenience of exceptions. When something goes wrong, you throw an error, catch it upstream, and let the runtime unwind the stack. Go, however, takes a different path: it ditches exceptions entirely and opts for explicit error handling. Let’s break down why Go’s approach feels jarring at first—and how it can lead to more robust code. C# Exceptions: The “Fire Alarm” Approach In C#, exceptions act like fire alarms. When a problem arises (e.g., a null reference, failed I/O operation), you throw an exception, which propagates up the call stack until it’s caught: try { var data = File.ReadAllText("missing.txt"); // Throws if file doesn’t exist } catch (FileNotFoundException ex) { Console.WriteLine($"Oops: {ex.Message}"); } This model is powerful but can lead to: Unpredictable control flow: Exceptions can emerge from anywhere. Hidden costs: Stack unwinding and try/catch blocks add overhead. Silent failures: Uncaught exceptions crash applications. Go’s Errors: Manual Checks with Benefits Go treats errors as ordinary values. Functions return errors alongside results, forcing developers to handle them immediately. The infamous if err != nil pattern becomes second nature: file, err := os.Open("missing.txt") if err != nil { fmt.Printf("Oops: %v\n", err) return // Handle or propagate the error } defer file.Close() Key differences: Explicit over implicit: No hidden control flow—errors are part of the function signature. No stack traces by default: Errors are simple values, but you can enrich them with context. Performance-friendly: No runtime cost unless you explicitly check. Panic/Recover: Go’s “Emergency Exit” Go does have a panic keyword, which works like throwing an exception. However, panic is reserved for unrecoverable issues (e.g., nil pointer dereferences). It’s not intended for routine error handling: func riskyOperation() { if somethingBad { panic("This is catastrophic!") } } // Recover from a panic (use sparingly!) defer func() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) }}() riskyOperation() This is closer to C#’s Environment.FailFast than a typical try/catch. In Go, panics are not for business logic. Why Go’s Approach Wins (and Frustrates) The Good Transparency: Errors are part of the code flow, making it easier to trace issues. Encourages resilience: You’re forced to address errors where they occur. Simpler codebases: No deep stack unwinding or complex exception hierarchies. The Annoying Verbosity: Writing if err != nil repeatedly feels tedious. Boilerplate: Adding context to errors requires manual effort (e.g., fmt.Errorf("step failed: %w", err)). Tips for C# Developers Transitioning to Go Adopt the Zen of Explicit Checks: Embrace the clarity of handling errors immediately. Wrap Errors Early: Use %w in fmt.Errorf to create wrapped errors for better debugging: if _, err := ParseConfig(); err != nil { return fmt.Errorf("config parsing failed: %w", err) } Use Linters: Tools like errcheck ensure you don’t ignore returned errors. Avoid Panic: Reserve it for truly unrecoverable scenarios (e.g., startup dependency failures). When to Use Which Model Stick with C# Exceptions: For complex applications with deep call stacks and centralized error logging. Choose Go’s Errors: For systems where predictability and performance matter (e.g., APIs, distributed services). Final Thoughts Go’s error handling feels like a step backward to C# developers—until it doesn’t. By treating errors as data, Go trades convenience for reliability, ensuring that no error goes unnoticed. Yes, you’ll write more if statements, but you’ll also spend less time debugging mysterious crashes. Up next: Comparing Go’s goroutines with C#’s async/await. Spoiler: It’s not even close.
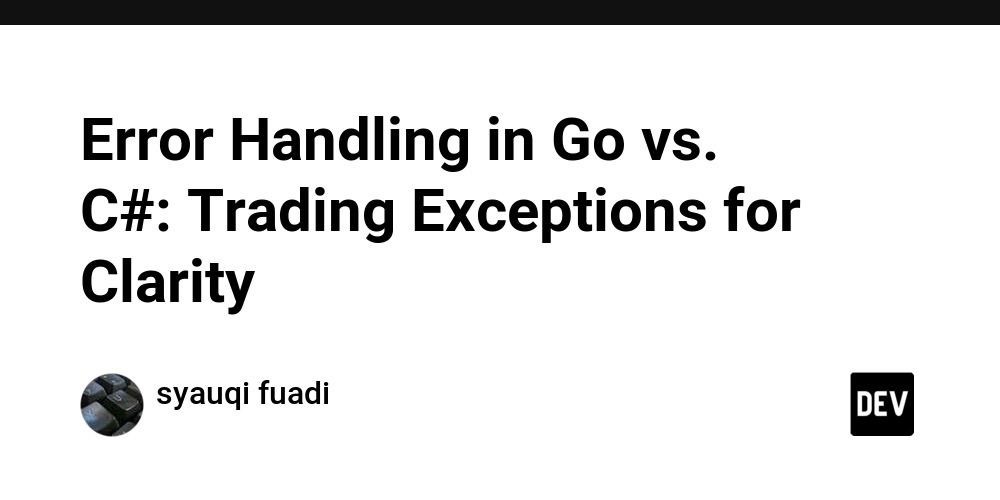
As a C# developer, you’re familiar with the convenience of exceptions. When something goes wrong, you throw
an error, catch
it upstream, and let the runtime unwind the stack. Go, however, takes a different path: it ditches exceptions entirely and opts for explicit error handling. Let’s break down why Go’s approach feels jarring at first—and how it can lead to more robust code.
C# Exceptions: The “Fire Alarm” Approach
In C#, exceptions act like fire alarms. When a problem arises (e.g., a null reference, failed I/O operation), you throw
an exception, which propagates up the call stack until it’s caught
:
try {
var data = File.ReadAllText("missing.txt"); // Throws if file doesn’t exist
}
catch (FileNotFoundException ex) {
Console.WriteLine($"Oops: {ex.Message}");
}
This model is powerful but can lead to:
- Unpredictable control flow: Exceptions can emerge from anywhere.
-
Hidden costs: Stack unwinding and
try/catch
blocks add overhead. - Silent failures: Uncaught exceptions crash applications.
Go’s Errors: Manual Checks with Benefits
Go treats errors as ordinary values. Functions return errors alongside results, forcing developers to handle them immediately. The infamous if err != nil
pattern becomes second nature:
file, err := os.Open("missing.txt")
if err != nil {
fmt.Printf("Oops: %v\n", err)
return // Handle or propagate the error
}
defer file.Close()
Key differences:
- Explicit over implicit: No hidden control flow—errors are part of the function signature.
- No stack traces by default: Errors are simple values, but you can enrich them with context.
- Performance-friendly: No runtime cost unless you explicitly check.
Panic/Recover: Go’s “Emergency Exit”
Go does have a panic
keyword, which works like throwing an exception. However, panic
is reserved for unrecoverable issues (e.g., nil pointer dereferences). It’s not intended for routine error handling:
func riskyOperation() {
if somethingBad {
panic("This is catastrophic!")
}
}
// Recover from a panic (use sparingly!)
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}}()
riskyOperation()
This is closer to C#’s Environment.FailFast
than a typical try/catch
. In Go, panics are not for business logic.
Why Go’s Approach Wins (and Frustrates)
The Good
- Transparency: Errors are part of the code flow, making it easier to trace issues.
- Encourages resilience: You’re forced to address errors where they occur.
- Simpler codebases: No deep stack unwinding or complex exception hierarchies.
The Annoying
-
Verbosity: Writing
if err != nil
repeatedly feels tedious. -
Boilerplate: Adding context to errors requires manual effort (e.g.,
fmt.Errorf("step failed: %w", err)
).
Tips for C# Developers Transitioning to Go
- Adopt the Zen of Explicit Checks: Embrace the clarity of handling errors immediately.
-
Wrap Errors Early: Use
%w
infmt.Errorf
to create wrapped errors for better debugging:
if _, err := ParseConfig(); err != nil {
return fmt.Errorf("config parsing failed: %w", err)
}
-
Use Linters: Tools like
errcheck
ensure you don’t ignore returned errors. - Avoid Panic: Reserve it for truly unrecoverable scenarios (e.g., startup dependency failures).
When to Use Which Model
- Stick with C# Exceptions: For complex applications with deep call stacks and centralized error logging.
- Choose Go’s Errors: For systems where predictability and performance matter (e.g., APIs, distributed services).
Final Thoughts
Go’s error handling feels like a step backward to C# developers—until it doesn’t. By treating errors as data, Go trades convenience for reliability, ensuring that no error goes unnoticed. Yes, you’ll write more if
statements, but you’ll also spend less time debugging mysterious crashes.
Up next: Comparing Go’s goroutines with C#’s async/await. Spoiler: It’s not even close.