Integrating with LoyVerse POS – A proper Guide
LoyVerse POS is a popular point-of-sale system for small businesses, offering APIs for seamless third-party integrations. Here’s a concise, developer-focused loyverse integration Step 1: Access LoyVerse Developer Portal Register as a Developer: Create an account on the LoyVerse Developer Portal. Generate API credentials (Client ID and Client Secret) for authentication. Review API Documentation: Study endpoints for key operations: Items: Retrieve or update product catalogs. Orders: Access sales data. Inventory: Sync stock levels. Step 2: Implement OAuth 2.0 Authentication Request Authorization: Redirect users to LoyVerse’s OAuth 2.0 authorization URL: Copy https://cloud.loyverse.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code Exchange Code for Tokens: Use the returned authorization_code to fetch access_token and refresh_token via POST to /oauth/token . Step 3: Call LoyVerse APIs Fetch Data: Use the access_token to call endpoints like /v1/items or /v1/orders. Example (Python): python Copy import requests headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'} response = requests.get('https://api.loyverse.com/v1/items', headers=headers) Handle Pagination: LoyVerse APIs paginate large datasets. Loop through cursor parameters to retrieve all records. Step 4: Set Up Webhooks (Optional) Subscribe to Events: Register webhooks to receive real-time updates (e.g., new orders, inventory changes). Use the /v1/webhooks endpoint to configure URLs for events like ORDER_CREATED or ITEM_UPDATED. Verify Signatures: Validate incoming webhook payloads using the X-Loyverse-Webhook-Signature header. Step 5: Test in Sandbox Mode Use LoyVerse’s sandbox environment to mock API interactions without affecting live data. Simulate edge cases (e.g., out-of-stock items, refunds). Step 6: Deploy & Monitor Rate Limits: LoyVerse enforces rate limits (e.g., 60 requests/minute). Implement retries with exponential backoff. Logging: Track API errors (e.g., 401 Unauthorized, 429 Too Many Requests
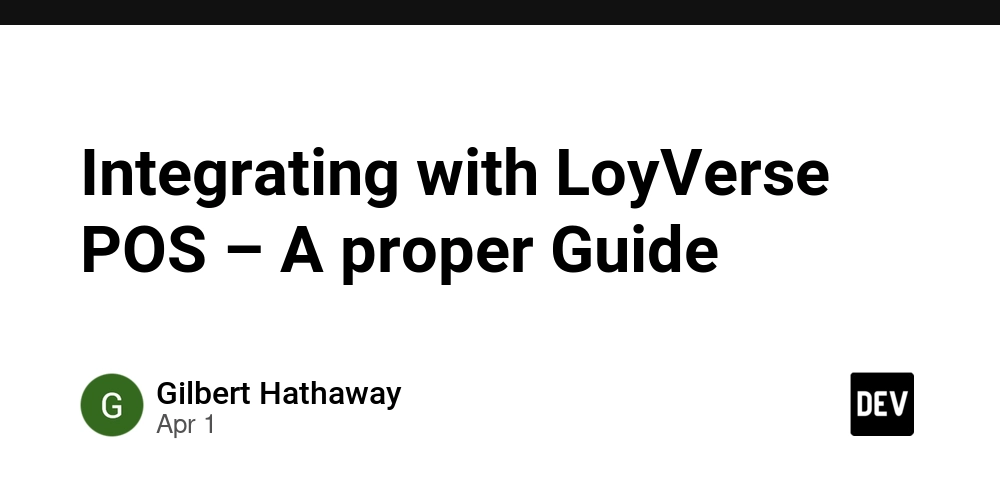
LoyVerse POS is a popular point-of-sale system for small businesses, offering APIs for seamless third-party integrations. Here’s a concise, developer-focused loyverse integration
Step 1: Access LoyVerse Developer Portal
- Register as a Developer:
- Create an account on the LoyVerse Developer Portal.
- Generate API credentials (Client ID and Client Secret) for authentication.
- Review API Documentation:
- Study endpoints for key operations:
- Items: Retrieve or update product catalogs.
- Orders: Access sales data.
- Inventory: Sync stock levels.
Step 2: Implement OAuth 2.0 Authentication
Request Authorization:
Redirect users to LoyVerse’s OAuth 2.0 authorization URL:
Copy
https://cloud.loyverse.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
Exchange Code for Tokens:
Use the returned authorization_code to fetch access_token and refresh_token via POST to /oauth/token
.
Step 3: Call LoyVerse APIs
Fetch Data:
Use the access_token to call endpoints like /v1/items or /v1/orders.
Example (Python):
python
Copy
import requests
headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}
response = requests.get('https://api.loyverse.com/v1/items', headers=headers)
Handle Pagination:
LoyVerse APIs paginate large datasets. Loop through cursor parameters to retrieve all records.
Step 4: Set Up Webhooks (Optional)
Subscribe to Events:
Register webhooks to receive real-time updates (e.g., new orders, inventory changes).
Use the /v1/webhooks endpoint to configure URLs for events like ORDER_CREATED or ITEM_UPDATED.
Verify Signatures:
Validate incoming webhook payloads using the X-Loyverse-Webhook-Signature header.
Step 5: Test in Sandbox Mode
Use LoyVerse’s sandbox environment to mock API interactions without affecting live data.
Simulate edge cases (e.g., out-of-stock items, refunds).
Step 6: Deploy & Monitor
Rate Limits: LoyVerse enforces rate limits (e.g., 60 requests/minute). Implement retries with exponential backoff.
Logging: Track API errors (e.g., 401 Unauthorized, 429 Too Many Requests