Explore Golang Metaprogramming: A Deep Dive
Leapcell: The Best of Serverless Web Hosting Introduction Reflection and metaprogramming, as advanced programming concepts, empower developers with the ability to inspect, modify, and control a program's behavior during its runtime. In the Go language ecosystem, although the language itself provides support for the reflection mechanism, considering that reflection operations incur runtime performance overhead and their implementation logic is relatively complex, it is not always the preferred choice in practical development. However, a deep understanding of the operation mechanisms of reflection and metaprogramming helps developers to have a more thorough grasp of the Go language and enables them to use it efficiently in necessary scenarios. Introduction to Reflection The Go language implements the reflection function through the reflect package. This function allows developers to obtain the dynamic type information and values of interfaces during the program's runtime. Common reflection operations include obtaining the kind of a type (such as determining whether the type is a slice, struct, or function, etc.), reading and modifying the content of values, and calling functions. package main import ( "fmt" "reflect" ) type leapstruct struct { Field1 int Field2 string } func (ls *leapstruct) Method1() { fmt.Println("Method1 called") } func main() { // Create a struct instance ls := leapstruct{10, "Hello"} // Get the reflection Value object v := reflect.ValueOf(&ls) // Get the method of the struct m := v.MethodByName("Method1") // Call the method m.Call(nil) } Detailed Explanation of Reflection The reflect package in the Go language mainly provides two important types: Type and Value. Type Type The Type type is an interface that represents a type in the Go language. It has multiple methods for querying type information: Kind(): Returns the kind of the type, such as Int, Float, Slice, etc. Name(): Returns the name of the type. PkgPath(): Returns the package path of the type. NumMethod(): Returns the number of methods of the type. Method(int): Returns the i-th method of the type. NumField(): Returns the number of fields of a struct type. Field(int): Returns the i-th field of a struct type. Value Type The Value type represents a value in the Go language and provides numerous methods for operating on the value: Kind(): Returns the kind of the value. Type(): Returns the type of the value. Interface(): Returns the value as an interface{}. Int(), Float(), String(), etc.: Converts the value to the corresponding type and returns it. SetInt(int64), SetFloat(float64), SetString(string), etc.: Sets the value to the corresponding type. Addr(): Returns the address of the value. CanAddr(): Determines whether the value can be addressed. CanSet(): Determines whether the value can be set. NumField(): Returns the number of fields of a struct value. Field(int): Returns the i-th field of a struct value. NumMethod(): Returns the number of methods of the value. Method(int): Returns the i-th method of the value. Examples of Using Reflection Example One The following example demonstrates the use of reflect.Type and reflect.Value: package main import ( "fmt" "reflect" ) type Person struct { Name string Age int } func main() { p := Person{Name: "Alice", Age: 20} t := reflect.TypeOf(p) v := reflect.ValueOf(p) fmt.Println(t.Name()) // Output: Person fmt.Println(t.Kind()) // Output: struct fmt.Println(v.Type()) // Output: main.Person fmt.Println(v.Kind()) // Output: struct fmt.Println(v.NumField()) // Output: 2 fmt.Println(v.Field(0)) // Output: Alice fmt.Println(v.Field(1)) // Output: 20 } In the above example, first, the Person struct is defined, and an instance of it is created. Then, the reflection objects of the type and value of the instance are obtained through reflect.TypeOf and reflect.ValueOf, and the methods of Type and Value are used to query the information of the type and value. Example Two This example demonstrates the use of more functions of the reflect package, such as method calling and value modification: package main import ( "fmt" "reflect" ) type Person struct { Name string Age int } func (p *Person) SayHello() { fmt.Printf("Hello, my name is %s, and I am %d years old.\n", p.Name, p.Age) } func main() { p := &Person{Name: "Bob", Age: 29} v := reflect.ValueOf(p) // Call the method m := v.MethodByName("SayHello") m.Call(nil) // Modify the value v.Elem().FieldByName("Age").SetInt(29) p.SayHello() // Output: Hello, my name is Bob, and I am 29 years old. } In this example, first, the Person struct is defined, and the SayHello method is added. After creating an instance, its reflection value ob
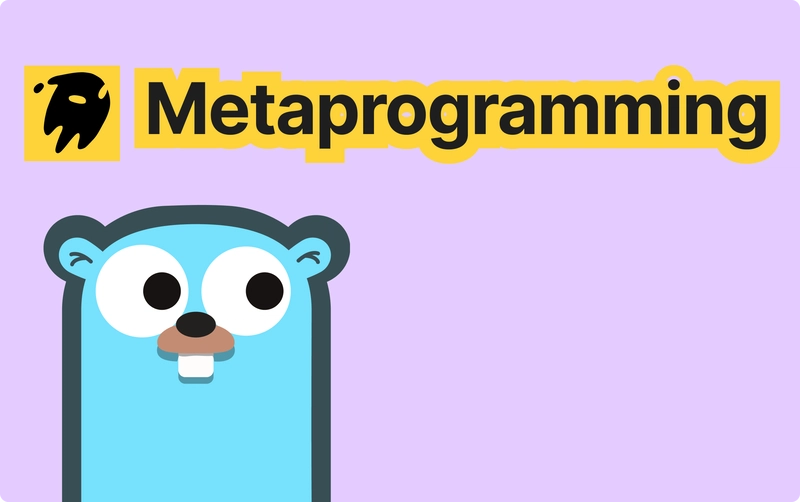
Leapcell: The Best of Serverless Web Hosting
Introduction
Reflection and metaprogramming, as advanced programming concepts, empower developers with the ability to inspect, modify, and control a program's behavior during its runtime. In the Go language ecosystem, although the language itself provides support for the reflection mechanism, considering that reflection operations incur runtime performance overhead and their implementation logic is relatively complex, it is not always the preferred choice in practical development. However, a deep understanding of the operation mechanisms of reflection and metaprogramming helps developers to have a more thorough grasp of the Go language and enables them to use it efficiently in necessary scenarios.
Introduction to Reflection
The Go language implements the reflection function through the reflect
package. This function allows developers to obtain the dynamic type information and values of interfaces during the program's runtime. Common reflection operations include obtaining the kind of a type (such as determining whether the type is a slice, struct, or function, etc.), reading and modifying the content of values, and calling functions.
package main
import (
"fmt"
"reflect"
)
type leapstruct struct {
Field1 int
Field2 string
}
func (ls *leapstruct) Method1() {
fmt.Println("Method1 called")
}
func main() {
// Create a struct instance
ls := leapstruct{10, "Hello"}
// Get the reflection Value object
v := reflect.ValueOf(&ls)
// Get the method of the struct
m := v.MethodByName("Method1")
// Call the method
m.Call(nil)
}
Detailed Explanation of Reflection
The reflect
package in the Go language mainly provides two important types: Type
and Value
.
Type
Type
The Type
type is an interface that represents a type in the Go language. It has multiple methods for querying type information:
-
Kind()
: Returns the kind of the type, such asInt
,Float
,Slice
, etc. -
Name()
: Returns the name of the type. -
PkgPath()
: Returns the package path of the type. -
NumMethod()
: Returns the number of methods of the type. -
Method(int)
: Returns thei
-th method of the type. -
NumField()
: Returns the number of fields of a struct type. -
Field(int)
: Returns thei
-th field of a struct type.
Value
Type
The Value
type represents a value in the Go language and provides numerous methods for operating on the value:
-
Kind()
: Returns the kind of the value. -
Type()
: Returns the type of the value. -
Interface()
: Returns the value as aninterface{}
. -
Int()
,Float()
,String()
, etc.: Converts the value to the corresponding type and returns it. -
SetInt(int64)
,SetFloat(float64)
,SetString(string)
, etc.: Sets the value to the corresponding type. -
Addr()
: Returns the address of the value. -
CanAddr()
: Determines whether the value can be addressed. -
CanSet()
: Determines whether the value can be set. -
NumField()
: Returns the number of fields of a struct value. -
Field(int)
: Returns thei
-th field of a struct value. -
NumMethod()
: Returns the number of methods of the value. -
Method(int)
: Returns thei
-th method of the value.
Examples of Using Reflection
Example One
The following example demonstrates the use of reflect.Type
and reflect.Value
:
package main
import (
"fmt"
"reflect"
)
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "Alice", Age: 20}
t := reflect.TypeOf(p)
v := reflect.ValueOf(p)
fmt.Println(t.Name()) // Output: Person
fmt.Println(t.Kind()) // Output: struct
fmt.Println(v.Type()) // Output: main.Person
fmt.Println(v.Kind()) // Output: struct
fmt.Println(v.NumField()) // Output: 2
fmt.Println(v.Field(0)) // Output: Alice
fmt.Println(v.Field(1)) // Output: 20
}
In the above example, first, the Person
struct is defined, and an instance of it is created. Then, the reflection objects of the type and value of the instance are obtained through reflect.TypeOf
and reflect.ValueOf
, and the methods of Type
and Value
are used to query the information of the type and value.
Example Two
This example demonstrates the use of more functions of the reflect
package, such as method calling and value modification:
package main
import (
"fmt"
"reflect"
)
type Person struct {
Name string
Age int
}
func (p *Person) SayHello() {
fmt.Printf("Hello, my name is %s, and I am %d years old.\n", p.Name, p.Age)
}
func main() {
p := &Person{Name: "Bob", Age: 29}
v := reflect.ValueOf(p)
// Call the method
m := v.MethodByName("SayHello")
m.Call(nil)
// Modify the value
v.Elem().FieldByName("Age").SetInt(29)
p.SayHello() // Output: Hello, my name is Bob, and I am 29 years old.
}
In this example, first, the Person
struct is defined, and the SayHello
method is added. After creating an instance, its reflection value object is obtained. The reflection object of the SayHello
method is obtained through Value.MethodByName
, and it is called using Value.Call
. Then, the value pointed to by the pointer is obtained through Value.Elem
, the reflection object of the Age
field is obtained using Value.FieldByName
, and the value is modified through Value.SetInt
. Finally, the SayHello
method is called again to verify that the Age
value has been modified. This example reflects the power of the reflection function and also shows the complexity of reflection operations. When using it, various errors and boundary conditions need to be handled carefully.
Basic Concepts and Practical Methods of Metaprogramming
Metaprogramming is a programming technique that allows programmers to manipulate code as data. Its main goals are to reduce code redundancy, improve the level of abstraction, and make the code easier to understand and maintain. Metaprogramming can be executed both at compile time and at runtime.
In the Go language, although it does not directly support metaprogramming features like C++ template metaprogramming or Python decorators, it provides some mechanisms and tools to achieve metaprogramming effects.
Code Generation
Code generation is the most common form of metaprogramming in the Go language, achieved by generating and compiling additional Go source code at compile time. The go generate
command provided by the Go standard toolchain executes commands by scanning special comments in the source code.
//go:generate stringer -type=Pill
type Pill int
const (
Placebo Pill = iota
Aspirin
Ibuprofen
Paracetamol
Amoxicillin
)
In the above example, the Pill
type and several constant values are defined, and the String
method for the Pill
type is generated through the go:generate
directive. stringer
is a tool provided by golang.org/x/tools/cmd/stringer
for generating String
methods for constants.
Reflection
Reflection is also a way to achieve metaprogramming, enabling the program to inspect the types of variables and values at runtime and operate on these values dynamically. The Go language implements the reflection function through the reflect
package.
func PrintFields(input interface{}) {
v := reflect.ValueOf(input)
for i := 0; i < v.NumField(); i++ {
field := v.Field(i)
fmt.Printf("Field %d: %v\n", i, field.Interface())
}
}
type leapstruct struct {
Field1 int
Field2 string
}
func main() {
ls := leapstruct{10, "Hello"}
PrintFields(ls)
}
In this example, the PrintFields
function is defined to print all the fields of any struct. The reflection value object of the input is obtained through the reflection reflect.ValueOf
, and then the NumField
and Field
methods are used to obtain and print all the fields.
Interfaces and Type Assertions
Interfaces and type assertions in the Go language can also achieve some metaprogramming effects. By defining interfaces and using type assertions, different types can be dynamically processed at runtime.
type Stringer interface {
String() string
}
func Print(input interface{}) {
if s, ok := input.(Stringer); ok {
fmt.Println(s.String())
} else {
fmt.Println(input)
}
}
type leapstruct struct {
Field string
}
func (ls leapstruct) String() string {
return "leapstruct: " + ls.Field
}
func main() {
ls := leapstruct{Field: "Hello"}
Print(ls) // Output: leapstruct: Hello
Print(23) // Output: 23
}
In this example, the Stringer
interface and its String()
method are defined, and then the Print
function is defined, which can accept inputs of any type. In the Print
function, an attempt is made to convert the input to the Stringer
interface. If the conversion is successful, the result of the String()
method is called and printed; otherwise, the input is printed directly. At the same time, the leapstruct
struct is defined and the Stringer
interface is implemented. In the main
function, the Print
function is called with both an instance of leapstruct
and an integer, demonstrating the ability of the Print
function to dynamically process different types at runtime.
Conclusion
Although the Go language does not directly provide metaprogramming features, with the help of mechanisms and tools such as code generation, reflection, interfaces, and type assertions, developers can achieve metaprogramming effects, manipulate the code during the programming process, improve the level of abstraction of the code, and enhance the understandability and maintainability of the code. However, when using these techniques, it should be noted that they may introduce complexity and increase the performance overhead at runtime. In reflection operations, various potential errors and boundary conditions should be handled carefully to ensure the stability and efficiency of the code.
Leapcell: The Best of Serverless Web Hosting
Finally, I would like to recommend the best platform for deploying Go services: Leapcell